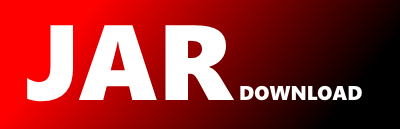
com.pulumi.awsnative.mediaconnect.kotlin.inputs.FlowFmtpArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.mediaconnect.kotlin.inputs
import com.pulumi.awsnative.mediaconnect.inputs.FlowFmtpArgs.builder
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFmtpColorimetry
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFmtpRange
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFmtpScanMode
import com.pulumi.awsnative.mediaconnect.kotlin.enums.FlowFmtpTcs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A set of parameters that define the media stream.
* @property channelOrder The format of the audio channel.
* @property colorimetry The format used for the representation of color.
* @property exactFramerate The frame rate for the video stream, in frames/second. For example: 60000/1001.
* @property par The pixel aspect ratio (PAR) of the video.
* @property range The encoding range of the video.
* @property scanMode The type of compression that was used to smooth the video's appearance.
* @property tcs The transfer characteristic system (TCS) that is used in the video.
*/
public data class FlowFmtpArgs(
public val channelOrder: Output? = null,
public val colorimetry: Output? = null,
public val exactFramerate: Output? = null,
public val par: Output? = null,
public val range: Output? = null,
public val scanMode: Output? = null,
public val tcs: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.mediaconnect.inputs.FlowFmtpArgs =
com.pulumi.awsnative.mediaconnect.inputs.FlowFmtpArgs.builder()
.channelOrder(channelOrder?.applyValue({ args0 -> args0 }))
.colorimetry(colorimetry?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.exactFramerate(exactFramerate?.applyValue({ args0 -> args0 }))
.par(par?.applyValue({ args0 -> args0 }))
.range(range?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scanMode(scanMode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tcs(tcs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FlowFmtpArgs].
*/
@PulumiTagMarker
public class FlowFmtpArgsBuilder internal constructor() {
private var channelOrder: Output? = null
private var colorimetry: Output? = null
private var exactFramerate: Output? = null
private var par: Output? = null
private var range: Output? = null
private var scanMode: Output? = null
private var tcs: Output? = null
/**
* @param value The format of the audio channel.
*/
@JvmName("egrvmighiyywjuko")
public suspend fun channelOrder(`value`: Output) {
this.channelOrder = value
}
/**
* @param value The format used for the representation of color.
*/
@JvmName("cgctntwlcjkxrvjq")
public suspend fun colorimetry(`value`: Output) {
this.colorimetry = value
}
/**
* @param value The frame rate for the video stream, in frames/second. For example: 60000/1001.
*/
@JvmName("rgkfvtjrnqvgtbjj")
public suspend fun exactFramerate(`value`: Output) {
this.exactFramerate = value
}
/**
* @param value The pixel aspect ratio (PAR) of the video.
*/
@JvmName("mielqiinvibeerun")
public suspend fun par(`value`: Output) {
this.par = value
}
/**
* @param value The encoding range of the video.
*/
@JvmName("ntbcgsvpqolpdcil")
public suspend fun range(`value`: Output) {
this.range = value
}
/**
* @param value The type of compression that was used to smooth the video's appearance.
*/
@JvmName("ibhujnklmeujfklq")
public suspend fun scanMode(`value`: Output) {
this.scanMode = value
}
/**
* @param value The transfer characteristic system (TCS) that is used in the video.
*/
@JvmName("cfbdgbtdxkwpkrdx")
public suspend fun tcs(`value`: Output) {
this.tcs = value
}
/**
* @param value The format of the audio channel.
*/
@JvmName("mqdbcmvceabwcyce")
public suspend fun channelOrder(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelOrder = mapped
}
/**
* @param value The format used for the representation of color.
*/
@JvmName("jrxcrajkjfogtetr")
public suspend fun colorimetry(`value`: FlowFmtpColorimetry?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.colorimetry = mapped
}
/**
* @param value The frame rate for the video stream, in frames/second. For example: 60000/1001.
*/
@JvmName("rjwcifhuaktwpgwa")
public suspend fun exactFramerate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exactFramerate = mapped
}
/**
* @param value The pixel aspect ratio (PAR) of the video.
*/
@JvmName("ekimeocndcstedhs")
public suspend fun par(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.par = mapped
}
/**
* @param value The encoding range of the video.
*/
@JvmName("unhnflautgirmqrh")
public suspend fun range(`value`: FlowFmtpRange?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.range = mapped
}
/**
* @param value The type of compression that was used to smooth the video's appearance.
*/
@JvmName("mosmxioemulcmeog")
public suspend fun scanMode(`value`: FlowFmtpScanMode?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scanMode = mapped
}
/**
* @param value The transfer characteristic system (TCS) that is used in the video.
*/
@JvmName("hmxmotqefmauwmxx")
public suspend fun tcs(`value`: FlowFmtpTcs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tcs = mapped
}
internal fun build(): FlowFmtpArgs = FlowFmtpArgs(
channelOrder = channelOrder,
colorimetry = colorimetry,
exactFramerate = exactFramerate,
par = par,
range = range,
scanMode = scanMode,
tcs = tcs,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy