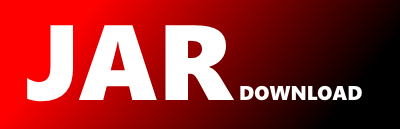
com.pulumi.awsnative.medialive.kotlin.MultiplexprogramArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.medialive.kotlin
import com.pulumi.awsnative.medialive.MultiplexprogramArgs.builder
import com.pulumi.awsnative.medialive.kotlin.enums.MultiplexprogramPreferredChannelPipeline
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramPacketIdentifiersMapArgs
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramPacketIdentifiersMapArgsBuilder
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramPipelineDetailArgs
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramPipelineDetailArgsBuilder
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramSettingsArgs
import com.pulumi.awsnative.medialive.kotlin.inputs.MultiplexprogramMultiplexProgramSettingsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::MediaLive::Multiplexprogram
* @property multiplexId The ID of the multiplex that the program belongs to.
* @property multiplexProgramSettings The settings for this multiplex program.
* @property packetIdentifiersMap The packet identifier map for this multiplex program.
* @property pipelineDetails Contains information about the current sources for the specified program in the specified multiplex. Keep in mind that each multiplex pipeline connects to both pipelines in a given source channel (the channel identified by the program). But only one of those channel pipelines is ever active at one time.
* @property preferredChannelPipeline The settings for this multiplex program.
* @property programName The name of the multiplex program.
*/
public data class MultiplexprogramArgs(
public val multiplexId: Output? = null,
public val multiplexProgramSettings: Output? = null,
public val packetIdentifiersMap: Output? =
null,
public val pipelineDetails: Output>? =
null,
public val preferredChannelPipeline: Output? = null,
public val programName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.medialive.MultiplexprogramArgs =
com.pulumi.awsnative.medialive.MultiplexprogramArgs.builder()
.multiplexId(multiplexId?.applyValue({ args0 -> args0 }))
.multiplexProgramSettings(
multiplexProgramSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.packetIdentifiersMap(
packetIdentifiersMap?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.pipelineDetails(
pipelineDetails?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.preferredChannelPipeline(
preferredChannelPipeline?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.programName(programName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MultiplexprogramArgs].
*/
@PulumiTagMarker
public class MultiplexprogramArgsBuilder internal constructor() {
private var multiplexId: Output? = null
private var multiplexProgramSettings: Output? = null
private var packetIdentifiersMap:
Output? = null
private var pipelineDetails: Output>? =
null
private var preferredChannelPipeline: Output? = null
private var programName: Output? = null
/**
* @param value The ID of the multiplex that the program belongs to.
*/
@JvmName("rnnqaeykmeslimkk")
public suspend fun multiplexId(`value`: Output) {
this.multiplexId = value
}
/**
* @param value The settings for this multiplex program.
*/
@JvmName("vgfyfnfrjqgbgvri")
public suspend fun multiplexProgramSettings(`value`: Output) {
this.multiplexProgramSettings = value
}
/**
* @param value The packet identifier map for this multiplex program.
*/
@JvmName("nreyymkqqgcanvpk")
public suspend fun packetIdentifiersMap(`value`: Output) {
this.packetIdentifiersMap = value
}
/**
* @param value Contains information about the current sources for the specified program in the specified multiplex. Keep in mind that each multiplex pipeline connects to both pipelines in a given source channel (the channel identified by the program). But only one of those channel pipelines is ever active at one time.
*/
@JvmName("geosxtnlkcojuutd")
public suspend fun pipelineDetails(`value`: Output>) {
this.pipelineDetails = value
}
@JvmName("qcshnevixktmfitw")
public suspend fun pipelineDetails(vararg values: Output) {
this.pipelineDetails = Output.all(values.asList())
}
/**
* @param values Contains information about the current sources for the specified program in the specified multiplex. Keep in mind that each multiplex pipeline connects to both pipelines in a given source channel (the channel identified by the program). But only one of those channel pipelines is ever active at one time.
*/
@JvmName("cjakyowmbfngsteh")
public suspend fun pipelineDetails(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy