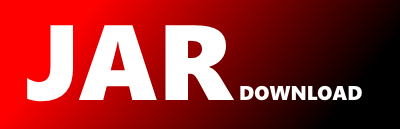
com.pulumi.awsnative.mediapackage.kotlin.outputs.GetOriginEndpointResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.mediapackage.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointOrigination
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property arn The Amazon Resource Name (ARN) assigned to the OriginEndpoint.
* @property authorization Parameters for CDN authorization.
* @property channelId The ID of the Channel the OriginEndpoint is associated with.
* @property cmafPackage Parameters for Common Media Application Format (CMAF) packaging.
* @property dashPackage Parameters for DASH packaging.
* @property description A short text description of the OriginEndpoint.
* @property hlsPackage Parameters for Apple HLS packaging.
* @property manifestName A short string appended to the end of the OriginEndpoint URL.
* @property mssPackage Parameters for Microsoft Smooth Streaming packaging.
* @property origination Control whether origination of video is allowed for this OriginEndpoint. If set to ALLOW, the OriginEndpoint may by requested, pursuant to any other form of access control. If set to DENY, the OriginEndpoint may not be requested. This can be helpful for Live to VOD harvesting, or for temporarily disabling origination
* @property startoverWindowSeconds Maximum duration (seconds) of content to retain for startover playback. If not specified, startover playback will be disabled for the OriginEndpoint.
* @property tags A collection of tags associated with a resource
* @property timeDelaySeconds Amount of delay (seconds) to enforce on the playback of live content. If not specified, there will be no time delay in effect for the OriginEndpoint.
* @property url The URL of the packaged OriginEndpoint for consumption.
* @property whitelist A list of source IP CIDR blocks that will be allowed to access the OriginEndpoint.
*/
public data class GetOriginEndpointResult(
public val arn: String? = null,
public val authorization: OriginEndpointAuthorization? = null,
public val channelId: String? = null,
public val cmafPackage: OriginEndpointCmafPackage? = null,
public val dashPackage: OriginEndpointDashPackage? = null,
public val description: String? = null,
public val hlsPackage: OriginEndpointHlsPackage? = null,
public val manifestName: String? = null,
public val mssPackage: OriginEndpointMssPackage? = null,
public val origination: OriginEndpointOrigination? = null,
public val startoverWindowSeconds: Int? = null,
public val tags: List? = null,
public val timeDelaySeconds: Int? = null,
public val url: String? = null,
public val whitelist: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.mediapackage.outputs.GetOriginEndpointResult): GetOriginEndpointResult = GetOriginEndpointResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
authorization = javaType.authorization().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointAuthorization.Companion.toKotlin(args0)
})
}).orElse(null),
channelId = javaType.channelId().map({ args0 -> args0 }).orElse(null),
cmafPackage = javaType.cmafPackage().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointCmafPackage.Companion.toKotlin(args0)
})
}).orElse(null),
dashPackage = javaType.dashPackage().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointDashPackage.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
hlsPackage = javaType.hlsPackage().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointHlsPackage.Companion.toKotlin(args0)
})
}).orElse(null),
manifestName = javaType.manifestName().map({ args0 -> args0 }).orElse(null),
mssPackage = javaType.mssPackage().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.outputs.OriginEndpointMssPackage.Companion.toKotlin(args0)
})
}).orElse(null),
origination = javaType.origination().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.mediapackage.kotlin.enums.OriginEndpointOrigination.Companion.toKotlin(args0)
})
}).orElse(null),
startoverWindowSeconds = javaType.startoverWindowSeconds().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
timeDelaySeconds = javaType.timeDelaySeconds().map({ args0 -> args0 }).orElse(null),
url = javaType.url().map({ args0 -> args0 }).orElse(null),
whitelist = javaType.whitelist().map({ args0 -> args0 }),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy