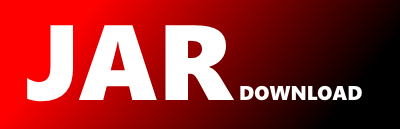
com.pulumi.awsnative.mediatailor.kotlin.Channel.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.mediatailor.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.mediatailor.kotlin.enums.ChannelPlaybackMode
import com.pulumi.awsnative.mediatailor.kotlin.enums.ChannelTier
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelLogConfigurationForChannel
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelRequestOutputItem
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelSlateSource
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelTimeShiftConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.enums.ChannelPlaybackMode.Companion.toKotlin as channelPlaybackModeToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.enums.ChannelTier.Companion.toKotlin as channelTierToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelLogConfigurationForChannel.Companion.toKotlin as channelLogConfigurationForChannelToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelRequestOutputItem.Companion.toKotlin as channelRequestOutputItemToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelSlateSource.Companion.toKotlin as channelSlateSourceToKotlin
import com.pulumi.awsnative.mediatailor.kotlin.outputs.ChannelTimeShiftConfiguration.Companion.toKotlin as channelTimeShiftConfigurationToKotlin
/**
* Builder for [Channel].
*/
@PulumiTagMarker
public class ChannelResourceBuilder internal constructor() {
public var name: String? = null
public var args: ChannelArgs = ChannelArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ChannelArgsBuilder.() -> Unit) {
val builder = ChannelArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Channel {
val builtJavaResource = com.pulumi.awsnative.mediatailor.Channel(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Channel(builtJavaResource)
}
}
/**
* Definition of AWS::MediaTailor::Channel Resource Type
*/
public class Channel internal constructor(
override val javaResource: com.pulumi.awsnative.mediatailor.Channel,
) : KotlinCustomResource(javaResource, ChannelMapper) {
/**
* The ARN of the channel.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The list of audiences defined in channel.
*/
public val audiences: Output>?
get() = javaResource.audiences().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* The name of the channel.
*/
public val channelName: Output
get() = javaResource.channelName().applyValue({ args0 -> args0 })
/**
* The slate used to fill gaps between programs in the schedule. You must configure filler slate if your channel uses the `LINEAR` `PlaybackMode` . MediaTailor doesn't support filler slate for channels using the `LOOP` `PlaybackMode` .
*/
public val fillerSlate: Output?
get() = javaResource.fillerSlate().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
channelSlateSourceToKotlin(args0)
})
}).orElse(null)
})
/**
* The log configuration.
*/
public val logConfiguration: Output?
get() = javaResource.logConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> channelLogConfigurationForChannelToKotlin(args0) })
}).orElse(null)
})
/**
* The channel's output properties.
*/
public val outputs: Output>
get() = javaResource.outputs().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
channelRequestOutputItemToKotlin(args0)
})
})
})
/**
* The type of playback mode for this channel.
* `LINEAR` - Programs play back-to-back only once.
* `LOOP` - Programs play back-to-back in an endless loop. When the last program in the schedule plays, playback loops back to the first program in the schedule.
*/
public val playbackMode: Output
get() = javaResource.playbackMode().applyValue({ args0 ->
args0.let({ args0 ->
channelPlaybackModeToKotlin(args0)
})
})
/**
* The tags to assign to the channel.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The tier for this channel. STANDARD tier channels can contain live programs.
*/
public val tier: Output?
get() = javaResource.tier().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
channelTierToKotlin(args0)
})
}).orElse(null)
})
/**
* The configuration for time-shifted viewing.
*/
public val timeShiftConfiguration: Output?
get() = javaResource.timeShiftConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> channelTimeShiftConfigurationToKotlin(args0) })
}).orElse(null)
})
}
public object ChannelMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.mediatailor.Channel::class == javaResource::class
override fun map(javaResource: Resource): Channel = Channel(
javaResource as
com.pulumi.awsnative.mediatailor.Channel,
)
}
/**
* @see [Channel].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Channel].
*/
public suspend fun channel(name: String, block: suspend ChannelResourceBuilder.() -> Unit): Channel {
val builder = ChannelResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Channel].
* @param name The _unique_ name of the resulting resource.
*/
public fun channel(name: String): Channel {
val builder = ChannelResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy