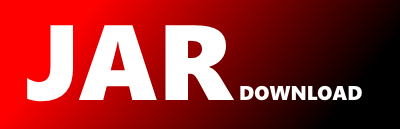
com.pulumi.awsnative.memorydb.kotlin.outputs.GetClusterResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.memorydb.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property aclName The name of the Access Control List to associate with the cluster.
* @property arn The Amazon Resource Name (ARN) of the cluster.
* @property autoMinorVersionUpgrade A flag that enables automatic minor version upgrade when set to true.
* You cannot modify the value of AutoMinorVersionUpgrade after the cluster is created. To enable AutoMinorVersionUpgrade on a cluster you must set AutoMinorVersionUpgrade to true when you create a cluster.
* @property clusterEndpoint The cluster endpoint.
* @property description An optional description of the cluster.
* @property engineVersion The Redis engine version used by the cluster.
* @property maintenanceWindow Specifies the weekly time range during which maintenance on the cluster is performed. It is specified as a range in the format ddd:hh24:mi-ddd:hh24:mi (24H Clock UTC). The minimum maintenance window is a 60 minute period.
* @property nodeType The compute and memory capacity of the nodes in the cluster.
* @property numReplicasPerShard The number of replicas to apply to each shard. The limit is 5.
* @property numShards The number of shards the cluster will contain.
* @property parameterGroupName The name of the parameter group associated with the cluster.
* @property parameterGroupStatus The status of the parameter group used by the cluster.
* @property securityGroupIds One or more Amazon VPC security groups associated with this cluster.
* @property snapshotRetentionLimit The number of days for which MemoryDB retains automatic snapshots before deleting them. For example, if you set SnapshotRetentionLimit to 5, a snapshot that was taken today is retained for 5 days before being deleted.
* @property snapshotWindow The daily time range (in UTC) during which MemoryDB begins taking a daily snapshot of your cluster.
* @property snsTopicArn The Amazon Resource Name (ARN) of the Amazon Simple Notification Service (SNS) topic to which notifications are sent.
* @property snsTopicStatus The status of the Amazon SNS notification topic. Notifications are sent only if the status is enabled.
* @property status The status of the cluster. For example, Available, Updating, Creating.
* @property tags An array of key-value pairs to apply to this cluster.
*/
public data class GetClusterResult(
public val aclName: String? = null,
public val arn: String? = null,
public val autoMinorVersionUpgrade: Boolean? = null,
public val clusterEndpoint: ClusterEndpoint? = null,
public val description: String? = null,
public val engineVersion: String? = null,
public val maintenanceWindow: String? = null,
public val nodeType: String? = null,
public val numReplicasPerShard: Int? = null,
public val numShards: Int? = null,
public val parameterGroupName: String? = null,
public val parameterGroupStatus: String? = null,
public val securityGroupIds: List? = null,
public val snapshotRetentionLimit: Int? = null,
public val snapshotWindow: String? = null,
public val snsTopicArn: String? = null,
public val snsTopicStatus: String? = null,
public val status: String? = null,
public val tags: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.memorydb.outputs.GetClusterResult): GetClusterResult = GetClusterResult(
aclName = javaType.aclName().map({ args0 -> args0 }).orElse(null),
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
autoMinorVersionUpgrade = javaType.autoMinorVersionUpgrade().map({ args0 -> args0 }).orElse(null),
clusterEndpoint = javaType.clusterEndpoint().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.memorydb.kotlin.outputs.ClusterEndpoint.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
engineVersion = javaType.engineVersion().map({ args0 -> args0 }).orElse(null),
maintenanceWindow = javaType.maintenanceWindow().map({ args0 -> args0 }).orElse(null),
nodeType = javaType.nodeType().map({ args0 -> args0 }).orElse(null),
numReplicasPerShard = javaType.numReplicasPerShard().map({ args0 -> args0 }).orElse(null),
numShards = javaType.numShards().map({ args0 -> args0 }).orElse(null),
parameterGroupName = javaType.parameterGroupName().map({ args0 -> args0 }).orElse(null),
parameterGroupStatus = javaType.parameterGroupStatus().map({ args0 -> args0 }).orElse(null),
securityGroupIds = javaType.securityGroupIds().map({ args0 -> args0 }),
snapshotRetentionLimit = javaType.snapshotRetentionLimit().map({ args0 -> args0 }).orElse(null),
snapshotWindow = javaType.snapshotWindow().map({ args0 -> args0 }).orElse(null),
snsTopicArn = javaType.snsTopicArn().map({ args0 -> args0 }).orElse(null),
snsTopicStatus = javaType.snsTopicStatus().map({ args0 -> args0 }).orElse(null),
status = javaType.status().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy