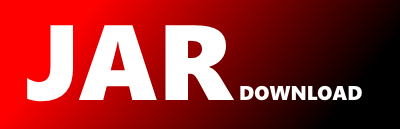
com.pulumi.awsnative.neptunegraph.kotlin.NeptunegraphFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.neptunegraph.kotlin
import com.pulumi.awsnative.neptunegraph.NeptunegraphFunctions.getGraphPlain
import com.pulumi.awsnative.neptunegraph.NeptunegraphFunctions.getPrivateGraphEndpointPlain
import com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetGraphPlainArgs
import com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetGraphPlainArgsBuilder
import com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetPrivateGraphEndpointPlainArgs
import com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetPrivateGraphEndpointPlainArgsBuilder
import com.pulumi.awsnative.neptunegraph.kotlin.outputs.GetGraphResult
import com.pulumi.awsnative.neptunegraph.kotlin.outputs.GetPrivateGraphEndpointResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.neptunegraph.kotlin.outputs.GetGraphResult.Companion.toKotlin as getGraphResultToKotlin
import com.pulumi.awsnative.neptunegraph.kotlin.outputs.GetPrivateGraphEndpointResult.Companion.toKotlin as getPrivateGraphEndpointResultToKotlin
public object NeptunegraphFunctions {
/**
* The AWS::NeptuneGraph::Graph resource creates an Amazon NeptuneGraph Graph.
* @param argument null
* @return null
*/
public suspend fun getGraph(argument: GetGraphPlainArgs): GetGraphResult =
getGraphResultToKotlin(getGraphPlain(argument.toJava()).await())
/**
* @see [getGraph].
* @param graphId The auto-generated id assigned by the service.
* @return null
*/
public suspend fun getGraph(graphId: String): GetGraphResult {
val argument = GetGraphPlainArgs(
graphId = graphId,
)
return getGraphResultToKotlin(getGraphPlain(argument.toJava()).await())
}
/**
* @see [getGraph].
* @param argument Builder for [com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetGraphPlainArgs].
* @return null
*/
public suspend fun getGraph(argument: suspend GetGraphPlainArgsBuilder.() -> Unit): GetGraphResult {
val builder = GetGraphPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGraphResultToKotlin(getGraphPlain(builtArgument.toJava()).await())
}
/**
* The AWS::NeptuneGraph::PrivateGraphEndpoint resource creates an Amazon NeptuneGraph PrivateGraphEndpoint.
* @param argument null
* @return null
*/
public suspend fun getPrivateGraphEndpoint(argument: GetPrivateGraphEndpointPlainArgs): GetPrivateGraphEndpointResult =
getPrivateGraphEndpointResultToKotlin(getPrivateGraphEndpointPlain(argument.toJava()).await())
/**
* @see [getPrivateGraphEndpoint].
* @param privateGraphEndpointIdentifier PrivateGraphEndpoint resource identifier generated by concatenating the associated GraphIdentifier and VpcId with an underscore separator.
* For example, if GraphIdentifier is `g-12a3bcdef4` and VpcId is `vpc-0a12bc34567de8f90`, the generated PrivateGraphEndpointIdentifier will be `g-12a3bcdef4_vpc-0a12bc34567de8f90`
* @return null
*/
public suspend fun getPrivateGraphEndpoint(privateGraphEndpointIdentifier: String): GetPrivateGraphEndpointResult {
val argument = GetPrivateGraphEndpointPlainArgs(
privateGraphEndpointIdentifier = privateGraphEndpointIdentifier,
)
return getPrivateGraphEndpointResultToKotlin(getPrivateGraphEndpointPlain(argument.toJava()).await())
}
/**
* @see [getPrivateGraphEndpoint].
* @param argument Builder for [com.pulumi.awsnative.neptunegraph.kotlin.inputs.GetPrivateGraphEndpointPlainArgs].
* @return null
*/
public suspend fun getPrivateGraphEndpoint(argument: suspend GetPrivateGraphEndpointPlainArgsBuilder.() -> Unit): GetPrivateGraphEndpointResult {
val builder = GetPrivateGraphEndpointPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getPrivateGraphEndpointResultToKotlin(getPrivateGraphEndpointPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy