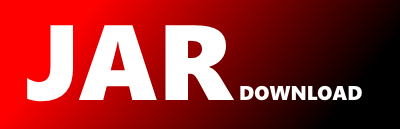
com.pulumi.awsnative.networkfirewall.kotlin.FirewallArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.networkfirewall.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.networkfirewall.FirewallArgs.builder
import com.pulumi.awsnative.networkfirewall.kotlin.inputs.FirewallSubnetMappingArgs
import com.pulumi.awsnative.networkfirewall.kotlin.inputs.FirewallSubnetMappingArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource type definition for AWS::NetworkFirewall::Firewall
* @property deleteProtection A flag indicating whether it is possible to delete the firewall. A setting of `TRUE` indicates that the firewall is protected against deletion. Use this setting to protect against accidentally deleting a firewall that is in use. When you create a firewall, the operation initializes this flag to `TRUE` .
* @property description A description of the firewall.
* @property firewallName The descriptive name of the firewall. You can't change the name of a firewall after you create it.
* @property firewallPolicyArn The Amazon Resource Name (ARN) of the firewall policy.
* The relationship of firewall to firewall policy is many to one. Each firewall requires one firewall policy association, and you can use the same firewall policy for multiple firewalls.
* @property firewallPolicyChangeProtection A setting indicating whether the firewall is protected against a change to the firewall policy association. Use this setting to protect against accidentally modifying the firewall policy for a firewall that is in use. When you create a firewall, the operation initializes this setting to `TRUE` .
* @property subnetChangeProtection A setting indicating whether the firewall is protected against changes to the subnet associations. Use this setting to protect against accidentally modifying the subnet associations for a firewall that is in use. When you create a firewall, the operation initializes this setting to `TRUE` .
* @property subnetMappings The public subnets that Network Firewall is using for the firewall. Each subnet must belong to a different Availability Zone.
* @property tags An array of key-value pairs to apply to this resource.
* For more information, see [Tag](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-properties-resource-tags.html) .
* @property vpcId The unique identifier of the VPC where the firewall is in use. You can't change the VPC of a firewall after you create the firewall.
*/
public data class FirewallArgs(
public val deleteProtection: Output? = null,
public val description: Output? = null,
public val firewallName: Output? = null,
public val firewallPolicyArn: Output? = null,
public val firewallPolicyChangeProtection: Output? = null,
public val subnetChangeProtection: Output? = null,
public val subnetMappings: Output>? = null,
public val tags: Output>? = null,
public val vpcId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.networkfirewall.FirewallArgs =
com.pulumi.awsnative.networkfirewall.FirewallArgs.builder()
.deleteProtection(deleteProtection?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.firewallName(firewallName?.applyValue({ args0 -> args0 }))
.firewallPolicyArn(firewallPolicyArn?.applyValue({ args0 -> args0 }))
.firewallPolicyChangeProtection(firewallPolicyChangeProtection?.applyValue({ args0 -> args0 }))
.subnetChangeProtection(subnetChangeProtection?.applyValue({ args0 -> args0 }))
.subnetMappings(
subnetMappings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.vpcId(vpcId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FirewallArgs].
*/
@PulumiTagMarker
public class FirewallArgsBuilder internal constructor() {
private var deleteProtection: Output? = null
private var description: Output? = null
private var firewallName: Output? = null
private var firewallPolicyArn: Output? = null
private var firewallPolicyChangeProtection: Output? = null
private var subnetChangeProtection: Output? = null
private var subnetMappings: Output>? = null
private var tags: Output>? = null
private var vpcId: Output? = null
/**
* @param value A flag indicating whether it is possible to delete the firewall. A setting of `TRUE` indicates that the firewall is protected against deletion. Use this setting to protect against accidentally deleting a firewall that is in use. When you create a firewall, the operation initializes this flag to `TRUE` .
*/
@JvmName("mhjvecugtgyxwcnr")
public suspend fun deleteProtection(`value`: Output) {
this.deleteProtection = value
}
/**
* @param value A description of the firewall.
*/
@JvmName("lqbkjpfriyqbwpne")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The descriptive name of the firewall. You can't change the name of a firewall after you create it.
*/
@JvmName("jucsvlttiashpqps")
public suspend fun firewallName(`value`: Output) {
this.firewallName = value
}
/**
* @param value The Amazon Resource Name (ARN) of the firewall policy.
* The relationship of firewall to firewall policy is many to one. Each firewall requires one firewall policy association, and you can use the same firewall policy for multiple firewalls.
*/
@JvmName("aaqjrgkulrdiwykm")
public suspend fun firewallPolicyArn(`value`: Output) {
this.firewallPolicyArn = value
}
/**
* @param value A setting indicating whether the firewall is protected against a change to the firewall policy association. Use this setting to protect against accidentally modifying the firewall policy for a firewall that is in use. When you create a firewall, the operation initializes this setting to `TRUE` .
*/
@JvmName("gdweqngklgffpswf")
public suspend fun firewallPolicyChangeProtection(`value`: Output) {
this.firewallPolicyChangeProtection = value
}
/**
* @param value A setting indicating whether the firewall is protected against changes to the subnet associations. Use this setting to protect against accidentally modifying the subnet associations for a firewall that is in use. When you create a firewall, the operation initializes this setting to `TRUE` .
*/
@JvmName("jkugyjrogeudsaew")
public suspend fun subnetChangeProtection(`value`: Output) {
this.subnetChangeProtection = value
}
/**
* @param value The public subnets that Network Firewall is using for the firewall. Each subnet must belong to a different Availability Zone.
*/
@JvmName("axafroveoyubeajp")
public suspend fun subnetMappings(`value`: Output>) {
this.subnetMappings = value
}
@JvmName("kkbixonndtvauceb")
public suspend fun subnetMappings(vararg values: Output) {
this.subnetMappings = Output.all(values.asList())
}
/**
* @param values The public subnets that Network Firewall is using for the firewall. Each subnet must belong to a different Availability Zone.
*/
@JvmName("csgvtibacelufgdm")
public suspend fun subnetMappings(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy