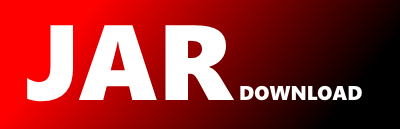
com.pulumi.awsnative.nimblestudio.kotlin.NimblestudioFunctions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.nimblestudio.kotlin
import com.pulumi.awsnative.nimblestudio.NimblestudioFunctions.getLaunchProfilePlain
import com.pulumi.awsnative.nimblestudio.NimblestudioFunctions.getStreamingImagePlain
import com.pulumi.awsnative.nimblestudio.NimblestudioFunctions.getStudioComponentPlain
import com.pulumi.awsnative.nimblestudio.NimblestudioFunctions.getStudioPlain
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetLaunchProfilePlainArgs
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetLaunchProfilePlainArgsBuilder
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStreamingImagePlainArgs
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStreamingImagePlainArgsBuilder
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioComponentPlainArgs
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioComponentPlainArgsBuilder
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioPlainArgs
import com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioPlainArgsBuilder
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetLaunchProfileResult
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStreamingImageResult
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStudioComponentResult
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStudioResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetLaunchProfileResult.Companion.toKotlin as getLaunchProfileResultToKotlin
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStreamingImageResult.Companion.toKotlin as getStreamingImageResultToKotlin
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStudioComponentResult.Companion.toKotlin as getStudioComponentResultToKotlin
import com.pulumi.awsnative.nimblestudio.kotlin.outputs.GetStudioResult.Companion.toKotlin as getStudioResultToKotlin
public object NimblestudioFunctions {
/**
* Represents a launch profile which delegates access to a collection of studio components to studio users
* @param argument null
* @return null
*/
public suspend fun getLaunchProfile(argument: GetLaunchProfilePlainArgs): GetLaunchProfileResult =
getLaunchProfileResultToKotlin(getLaunchProfilePlain(argument.toJava()).await())
/**
* @see [getLaunchProfile].
* @param launchProfileId The unique identifier for the launch profile resource.
* @param studioId The studio ID.
* @return null
*/
public suspend fun getLaunchProfile(launchProfileId: String, studioId: String): GetLaunchProfileResult {
val argument = GetLaunchProfilePlainArgs(
launchProfileId = launchProfileId,
studioId = studioId,
)
return getLaunchProfileResultToKotlin(getLaunchProfilePlain(argument.toJava()).await())
}
/**
* @see [getLaunchProfile].
* @param argument Builder for [com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetLaunchProfilePlainArgs].
* @return null
*/
public suspend fun getLaunchProfile(argument: suspend GetLaunchProfilePlainArgsBuilder.() -> Unit): GetLaunchProfileResult {
val builder = GetLaunchProfilePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getLaunchProfileResultToKotlin(getLaunchProfilePlain(builtArgument.toJava()).await())
}
/**
* Represents a streaming session machine image that can be used to launch a streaming session
* @param argument null
* @return null
*/
public suspend fun getStreamingImage(argument: GetStreamingImagePlainArgs): GetStreamingImageResult =
getStreamingImageResultToKotlin(getStreamingImagePlain(argument.toJava()).await())
/**
* @see [getStreamingImage].
* @param streamingImageId The unique identifier for the streaming image resource.
* @param studioId The studioId.
* @return null
*/
public suspend fun getStreamingImage(streamingImageId: String, studioId: String): GetStreamingImageResult {
val argument = GetStreamingImagePlainArgs(
streamingImageId = streamingImageId,
studioId = studioId,
)
return getStreamingImageResultToKotlin(getStreamingImagePlain(argument.toJava()).await())
}
/**
* @see [getStreamingImage].
* @param argument Builder for [com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStreamingImagePlainArgs].
* @return null
*/
public suspend fun getStreamingImage(argument: suspend GetStreamingImagePlainArgsBuilder.() -> Unit): GetStreamingImageResult {
val builder = GetStreamingImagePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStreamingImageResultToKotlin(getStreamingImagePlain(builtArgument.toJava()).await())
}
/**
* Represents a studio that contains other Nimble Studio resources
* @param argument null
* @return null
*/
public suspend fun getStudio(argument: GetStudioPlainArgs): GetStudioResult =
getStudioResultToKotlin(getStudioPlain(argument.toJava()).await())
/**
* @see [getStudio].
* @param studioId The unique identifier for the studio resource.
* @return null
*/
public suspend fun getStudio(studioId: String): GetStudioResult {
val argument = GetStudioPlainArgs(
studioId = studioId,
)
return getStudioResultToKotlin(getStudioPlain(argument.toJava()).await())
}
/**
* @see [getStudio].
* @param argument Builder for [com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioPlainArgs].
* @return null
*/
public suspend fun getStudio(argument: suspend GetStudioPlainArgsBuilder.() -> Unit): GetStudioResult {
val builder = GetStudioPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStudioResultToKotlin(getStudioPlain(builtArgument.toJava()).await())
}
/**
* Represents a studio component that connects a non-Nimble Studio resource in your account to your studio
* @param argument null
* @return null
*/
public suspend fun getStudioComponent(argument: GetStudioComponentPlainArgs): GetStudioComponentResult =
getStudioComponentResultToKotlin(getStudioComponentPlain(argument.toJava()).await())
/**
* @see [getStudioComponent].
* @param studioComponentId The unique identifier for the studio component resource.
* @param studioId The studio ID.
* @return null
*/
public suspend fun getStudioComponent(studioComponentId: String, studioId: String): GetStudioComponentResult {
val argument = GetStudioComponentPlainArgs(
studioComponentId = studioComponentId,
studioId = studioId,
)
return getStudioComponentResultToKotlin(getStudioComponentPlain(argument.toJava()).await())
}
/**
* @see [getStudioComponent].
* @param argument Builder for [com.pulumi.awsnative.nimblestudio.kotlin.inputs.GetStudioComponentPlainArgs].
* @return null
*/
public suspend fun getStudioComponent(argument: suspend GetStudioComponentPlainArgsBuilder.() -> Unit): GetStudioComponentResult {
val builder = GetStudioComponentPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getStudioComponentResultToKotlin(getStudioComponentPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy