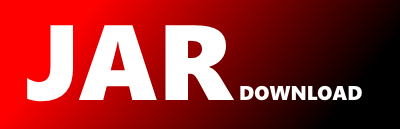
com.pulumi.awsnative.opensearchserverless.kotlin.CollectionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.opensearchserverless.kotlin
import com.pulumi.awsnative.kotlin.inputs.CreateOnlyTagArgs
import com.pulumi.awsnative.kotlin.inputs.CreateOnlyTagArgsBuilder
import com.pulumi.awsnative.opensearchserverless.CollectionArgs.builder
import com.pulumi.awsnative.opensearchserverless.kotlin.enums.CollectionStandbyReplicas
import com.pulumi.awsnative.opensearchserverless.kotlin.enums.CollectionType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Amazon OpenSearchServerless collection resource
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property description The description of the collection
* @property name The name of the collection.
* The name must meet the following criteria:
* Unique to your account and AWS Region
* Starts with a lowercase letter
* Contains only lowercase letters a-z, the numbers 0-9 and the hyphen (-)
* Contains between 3 and 32 characters
* @property standbyReplicas Indicates whether to use standby replicas for the collection. You can't update this property after the collection is already created. If you attempt to modify this property, the collection continues to use the original value.
* @property tags List of tags to be added to the resource
* @property type The type of collection. Possible values are `SEARCH` , `TIMESERIES` , and `VECTORSEARCH` . For more information, see [Choosing a collection type](https://docs.aws.amazon.com/opensearch-service/latest/developerguide/serverless-overview.html#serverless-usecase) .
*/
public data class CollectionArgs(
public val description: Output? = null,
public val name: Output? = null,
public val standbyReplicas: Output? = null,
public val tags: Output>? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.opensearchserverless.CollectionArgs =
com.pulumi.awsnative.opensearchserverless.CollectionArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.standbyReplicas(standbyReplicas?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tags(tags?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CollectionArgs].
*/
@PulumiTagMarker
public class CollectionArgsBuilder internal constructor() {
private var description: Output? = null
private var name: Output? = null
private var standbyReplicas: Output? = null
private var tags: Output>? = null
private var type: Output? = null
/**
* @param value The description of the collection
*/
@JvmName("apgeedsqpwfpqdyb")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The name of the collection.
* The name must meet the following criteria:
* Unique to your account and AWS Region
* Starts with a lowercase letter
* Contains only lowercase letters a-z, the numbers 0-9 and the hyphen (-)
* Contains between 3 and 32 characters
*/
@JvmName("vjnipiymqderpljk")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Indicates whether to use standby replicas for the collection. You can't update this property after the collection is already created. If you attempt to modify this property, the collection continues to use the original value.
*/
@JvmName("bjqlibmeumayllbo")
public suspend fun standbyReplicas(`value`: Output) {
this.standbyReplicas = value
}
/**
* @param value List of tags to be added to the resource
*/
@JvmName("kymspixwrbfgefdc")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("cpxdnvmxhurropdg")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values List of tags to be added to the resource
*/
@JvmName("hgfwnmxtlhifeiux")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy