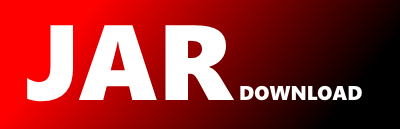
com.pulumi.awsnative.paymentcryptography.kotlin.Key.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.paymentcryptography.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyCheckValueAlgorithm
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyOrigin
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyState
import com.pulumi.awsnative.paymentcryptography.kotlin.outputs.KeyAttributes
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyCheckValueAlgorithm.Companion.toKotlin as keyCheckValueAlgorithmToKotlin
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyOrigin.Companion.toKotlin as keyOriginToKotlin
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyState.Companion.toKotlin as keyStateToKotlin
import com.pulumi.awsnative.paymentcryptography.kotlin.outputs.KeyAttributes.Companion.toKotlin as keyAttributesToKotlin
/**
* Builder for [Key].
*/
@PulumiTagMarker
public class KeyResourceBuilder internal constructor() {
public var name: String? = null
public var args: KeyArgs = KeyArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend KeyArgsBuilder.() -> Unit) {
val builder = KeyArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Key {
val builtJavaResource = com.pulumi.awsnative.paymentcryptography.Key(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Key(builtJavaResource)
}
}
/**
* Definition of AWS::PaymentCryptography::Key Resource Type
*/
public class Key internal constructor(
override val javaResource: com.pulumi.awsnative.paymentcryptography.Key,
) : KotlinCustomResource(javaResource, KeyMapper) {
/**
* Specifies whether the key is enabled.
*/
public val enabled: Output?
get() = javaResource.enabled().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies whether the key is exportable. This data is immutable after the key is created.
*/
public val exportable: Output
get() = javaResource.exportable().applyValue({ args0 -> args0 })
/**
* The role of the key, the algorithm it supports, and the cryptographic operations allowed with the key. This data is immutable after the key is created.
*/
public val keyAttributes: Output
get() = javaResource.keyAttributes().applyValue({ args0 ->
args0.let({ args0 ->
keyAttributesToKotlin(args0)
})
})
/**
* The algorithm that AWS Payment Cryptography uses to calculate the key check value (KCV). It is used to validate the key integrity.
* For TDES keys, the KCV is computed by encrypting 8 bytes, each with value of zero, with the key to be checked and retaining the 3 highest order bytes of the encrypted result. For AES keys, the KCV is computed using a CMAC algorithm where the input data is 16 bytes of zero and retaining the 3 highest order bytes of the encrypted result.
*/
public val keyCheckValueAlgorithm: Output?
get() = javaResource.keyCheckValueAlgorithm().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> keyCheckValueAlgorithmToKotlin(args0) })
}).orElse(null)
})
public val keyIdentifier: Output
get() = javaResource.keyIdentifier().applyValue({ args0 -> args0 })
/**
* The source of the key material. For keys created within AWS Payment Cryptography, the value is `AWS_PAYMENT_CRYPTOGRAPHY` . For keys imported into AWS Payment Cryptography, the value is `EXTERNAL` .
*/
public val keyOrigin: Output
get() = javaResource.keyOrigin().applyValue({ args0 ->
args0.let({ args0 ->
keyOriginToKotlin(args0)
})
})
/**
* The state of key that is being created or deleted.
*/
public val keyState: Output
get() = javaResource.keyState().applyValue({ args0 ->
args0.let({ args0 ->
keyStateToKotlin(args0)
})
})
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object KeyMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.paymentcryptography.Key::class == javaResource::class
override fun map(javaResource: Resource): Key = Key(
javaResource as
com.pulumi.awsnative.paymentcryptography.Key,
)
}
/**
* @see [Key].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Key].
*/
public suspend fun key(name: String, block: suspend KeyResourceBuilder.() -> Unit): Key {
val builder = KeyResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Key].
* @param name The _unique_ name of the resulting resource.
*/
public fun key(name: String): Key {
val builder = KeyResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy