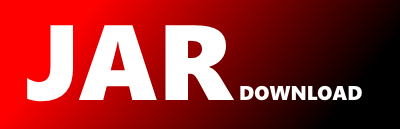
com.pulumi.awsnative.paymentcryptography.kotlin.inputs.KeyAttributesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.paymentcryptography.kotlin.inputs
import com.pulumi.awsnative.paymentcryptography.inputs.KeyAttributesArgs.builder
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyAlgorithm
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyClass
import com.pulumi.awsnative.paymentcryptography.kotlin.enums.KeyUsage
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property keyAlgorithm The key algorithm to be use during creation of an AWS Payment Cryptography key.
* For symmetric keys, AWS Payment Cryptography supports `AES` and `TDES` algorithms. For asymmetric keys, AWS Payment Cryptography supports `RSA` and `ECC_NIST` algorithms.
* @property keyClass The type of AWS Payment Cryptography key to create, which determines the classification of the cryptographic method and whether AWS Payment Cryptography key contains a symmetric key or an asymmetric key pair.
* @property keyModesOfUse The list of cryptographic operations that you can perform using the key.
* @property keyUsage The cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
public data class KeyAttributesArgs(
public val keyAlgorithm: Output,
public val keyClass: Output,
public val keyModesOfUse: Output,
public val keyUsage: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.paymentcryptography.inputs.KeyAttributesArgs =
com.pulumi.awsnative.paymentcryptography.inputs.KeyAttributesArgs.builder()
.keyAlgorithm(keyAlgorithm.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.keyClass(keyClass.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.keyModesOfUse(keyModesOfUse.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.keyUsage(keyUsage.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [KeyAttributesArgs].
*/
@PulumiTagMarker
public class KeyAttributesArgsBuilder internal constructor() {
private var keyAlgorithm: Output? = null
private var keyClass: Output? = null
private var keyModesOfUse: Output? = null
private var keyUsage: Output? = null
/**
* @param value The key algorithm to be use during creation of an AWS Payment Cryptography key.
* For symmetric keys, AWS Payment Cryptography supports `AES` and `TDES` algorithms. For asymmetric keys, AWS Payment Cryptography supports `RSA` and `ECC_NIST` algorithms.
*/
@JvmName("qbctatjjyopiupkc")
public suspend fun keyAlgorithm(`value`: Output) {
this.keyAlgorithm = value
}
/**
* @param value The type of AWS Payment Cryptography key to create, which determines the classification of the cryptographic method and whether AWS Payment Cryptography key contains a symmetric key or an asymmetric key pair.
*/
@JvmName("blfkppuevfkbxajh")
public suspend fun keyClass(`value`: Output) {
this.keyClass = value
}
/**
* @param value The list of cryptographic operations that you can perform using the key.
*/
@JvmName("lqjugpkigldpssnq")
public suspend fun keyModesOfUse(`value`: Output) {
this.keyModesOfUse = value
}
/**
* @param value The cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
@JvmName("rxoisfbalglwvrme")
public suspend fun keyUsage(`value`: Output) {
this.keyUsage = value
}
/**
* @param value The key algorithm to be use during creation of an AWS Payment Cryptography key.
* For symmetric keys, AWS Payment Cryptography supports `AES` and `TDES` algorithms. For asymmetric keys, AWS Payment Cryptography supports `RSA` and `ECC_NIST` algorithms.
*/
@JvmName("xbfvhelhlpkmgcvq")
public suspend fun keyAlgorithm(`value`: KeyAlgorithm) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyAlgorithm = mapped
}
/**
* @param value The type of AWS Payment Cryptography key to create, which determines the classification of the cryptographic method and whether AWS Payment Cryptography key contains a symmetric key or an asymmetric key pair.
*/
@JvmName("buicnjdvjyxypwlu")
public suspend fun keyClass(`value`: KeyClass) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyClass = mapped
}
/**
* @param value The list of cryptographic operations that you can perform using the key.
*/
@JvmName("qpjqrhwcomeolpgr")
public suspend fun keyModesOfUse(`value`: KeyModesOfUseArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyModesOfUse = mapped
}
/**
* @param argument The list of cryptographic operations that you can perform using the key.
*/
@JvmName("rbkngbjvwvukwixw")
public suspend fun keyModesOfUse(argument: suspend KeyModesOfUseArgsBuilder.() -> Unit) {
val toBeMapped = KeyModesOfUseArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.keyModesOfUse = mapped
}
/**
* @param value The cryptographic usage of an AWS Payment Cryptography key as defined in section A.5.2 of the TR-31 spec.
*/
@JvmName("otqxyjjdfdgieric")
public suspend fun keyUsage(`value`: KeyUsage) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.keyUsage = mapped
}
internal fun build(): KeyAttributesArgs = KeyAttributesArgs(
keyAlgorithm = keyAlgorithm ?: throw PulumiNullFieldException("keyAlgorithm"),
keyClass = keyClass ?: throw PulumiNullFieldException("keyClass"),
keyModesOfUse = keyModesOfUse ?: throw PulumiNullFieldException("keyModesOfUse"),
keyUsage = keyUsage ?: throw PulumiNullFieldException("keyUsage"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy