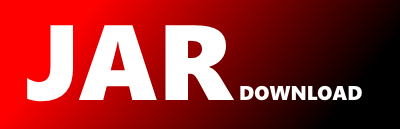
com.pulumi.awsnative.personalize.kotlin.SolutionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.personalize.kotlin
import com.pulumi.awsnative.personalize.SolutionArgs.builder
import com.pulumi.awsnative.personalize.kotlin.inputs.SolutionConfigArgs
import com.pulumi.awsnative.personalize.kotlin.inputs.SolutionConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::Personalize::Solution.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property datasetGroupArn The ARN of the dataset group that provides the training data.
* @property eventType When your have multiple event types (using an EVENT_TYPE schema field), this parameter specifies which event type (for example, 'click' or 'like') is used for training the model. If you do not provide an eventType, Amazon Personalize will use all interactions for training with equal weight regardless of type.
* @property name The name for the solution
* @property performAutoMl Whether to perform automated machine learning (AutoML). The default is false. For this case, you must specify recipeArn.
* @property performHpo Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is false. When performing AutoML, this parameter is always true and you should not set it to false.
* @property recipeArn The ARN of the recipe to use for model training. Only specified when performAutoML is false.
* @property solutionConfig Describes the configuration properties for the solution.
*/
public data class SolutionArgs(
public val datasetGroupArn: Output? = null,
public val eventType: Output? = null,
public val name: Output? = null,
public val performAutoMl: Output? = null,
public val performHpo: Output? = null,
public val recipeArn: Output? = null,
public val solutionConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.personalize.SolutionArgs =
com.pulumi.awsnative.personalize.SolutionArgs.builder()
.datasetGroupArn(datasetGroupArn?.applyValue({ args0 -> args0 }))
.eventType(eventType?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.performAutoMl(performAutoMl?.applyValue({ args0 -> args0 }))
.performHpo(performHpo?.applyValue({ args0 -> args0 }))
.recipeArn(recipeArn?.applyValue({ args0 -> args0 }))
.solutionConfig(
solutionConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [SolutionArgs].
*/
@PulumiTagMarker
public class SolutionArgsBuilder internal constructor() {
private var datasetGroupArn: Output? = null
private var eventType: Output? = null
private var name: Output? = null
private var performAutoMl: Output? = null
private var performHpo: Output? = null
private var recipeArn: Output? = null
private var solutionConfig: Output? = null
/**
* @param value The ARN of the dataset group that provides the training data.
*/
@JvmName("rfwjdhnqlchwmbuk")
public suspend fun datasetGroupArn(`value`: Output) {
this.datasetGroupArn = value
}
/**
* @param value When your have multiple event types (using an EVENT_TYPE schema field), this parameter specifies which event type (for example, 'click' or 'like') is used for training the model. If you do not provide an eventType, Amazon Personalize will use all interactions for training with equal weight regardless of type.
*/
@JvmName("dxeweyvlywdwpujo")
public suspend fun eventType(`value`: Output) {
this.eventType = value
}
/**
* @param value The name for the solution
*/
@JvmName("snjofrujpmyjlwbn")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Whether to perform automated machine learning (AutoML). The default is false. For this case, you must specify recipeArn.
*/
@JvmName("odvrjyahdshqagwq")
public suspend fun performAutoMl(`value`: Output) {
this.performAutoMl = value
}
/**
* @param value Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is false. When performing AutoML, this parameter is always true and you should not set it to false.
*/
@JvmName("idtcgaybxobilpaf")
public suspend fun performHpo(`value`: Output) {
this.performHpo = value
}
/**
* @param value The ARN of the recipe to use for model training. Only specified when performAutoML is false.
*/
@JvmName("bbyiiwqolkqbjigp")
public suspend fun recipeArn(`value`: Output) {
this.recipeArn = value
}
/**
* @param value Describes the configuration properties for the solution.
*/
@JvmName("xhjvwtawxwknvbvn")
public suspend fun solutionConfig(`value`: Output) {
this.solutionConfig = value
}
/**
* @param value The ARN of the dataset group that provides the training data.
*/
@JvmName("jjkjbahqglrejjak")
public suspend fun datasetGroupArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.datasetGroupArn = mapped
}
/**
* @param value When your have multiple event types (using an EVENT_TYPE schema field), this parameter specifies which event type (for example, 'click' or 'like') is used for training the model. If you do not provide an eventType, Amazon Personalize will use all interactions for training with equal weight regardless of type.
*/
@JvmName("jkneomrjfvuoqetr")
public suspend fun eventType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventType = mapped
}
/**
* @param value The name for the solution
*/
@JvmName("qnpuuvpdayxqacrp")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Whether to perform automated machine learning (AutoML). The default is false. For this case, you must specify recipeArn.
*/
@JvmName("fbgpaebrbqvrdoyo")
public suspend fun performAutoMl(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.performAutoMl = mapped
}
/**
* @param value Whether to perform hyperparameter optimization (HPO) on the specified or selected recipe. The default is false. When performing AutoML, this parameter is always true and you should not set it to false.
*/
@JvmName("kgyybcrqaoommfrt")
public suspend fun performHpo(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.performHpo = mapped
}
/**
* @param value The ARN of the recipe to use for model training. Only specified when performAutoML is false.
*/
@JvmName("xaprxstpvdmdutcr")
public suspend fun recipeArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recipeArn = mapped
}
/**
* @param value Describes the configuration properties for the solution.
*/
@JvmName("mujglgoxpswjimem")
public suspend fun solutionConfig(`value`: SolutionConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.solutionConfig = mapped
}
/**
* @param argument Describes the configuration properties for the solution.
*/
@JvmName("fqmavjjhcetcpssg")
public suspend fun solutionConfig(argument: suspend SolutionConfigArgsBuilder.() -> Unit) {
val toBeMapped = SolutionConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.solutionConfig = mapped
}
internal fun build(): SolutionArgs = SolutionArgs(
datasetGroupArn = datasetGroupArn,
eventType = eventType,
name = name,
performAutoMl = performAutoMl,
performHpo = performHpo,
recipeArn = recipeArn,
solutionConfig = solutionConfig,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy