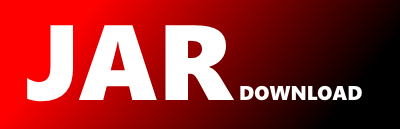
com.pulumi.awsnative.pinpoint.kotlin.inputs.InAppTemplateInAppMessageContentArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.pinpoint.kotlin.inputs
import com.pulumi.awsnative.pinpoint.inputs.InAppTemplateInAppMessageContentArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backgroundColor The background color for an in-app message banner, expressed as a hex color code (such as #000000 for black).
* @property bodyConfig An object that contains configuration information about the header or title text of the in-app message.
* @property headerConfig An object that contains configuration information about the header or title text of the in-app message.
* @property imageUrl The URL of the image that appears on an in-app message banner.
* @property primaryBtn An object that contains configuration information about the primary button in an in-app message.
* @property secondaryBtn An object that contains configuration information about the secondary button in an in-app message.
*/
public data class InAppTemplateInAppMessageContentArgs(
public val backgroundColor: Output? = null,
public val bodyConfig: Output? = null,
public val headerConfig: Output? = null,
public val imageUrl: Output? = null,
public val primaryBtn: Output? = null,
public val secondaryBtn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.pinpoint.inputs.InAppTemplateInAppMessageContentArgs =
com.pulumi.awsnative.pinpoint.inputs.InAppTemplateInAppMessageContentArgs.builder()
.backgroundColor(backgroundColor?.applyValue({ args0 -> args0 }))
.bodyConfig(bodyConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.headerConfig(headerConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.imageUrl(imageUrl?.applyValue({ args0 -> args0 }))
.primaryBtn(primaryBtn?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secondaryBtn(secondaryBtn?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [InAppTemplateInAppMessageContentArgs].
*/
@PulumiTagMarker
public class InAppTemplateInAppMessageContentArgsBuilder internal constructor() {
private var backgroundColor: Output? = null
private var bodyConfig: Output? = null
private var headerConfig: Output? = null
private var imageUrl: Output? = null
private var primaryBtn: Output? = null
private var secondaryBtn: Output? = null
/**
* @param value The background color for an in-app message banner, expressed as a hex color code (such as #000000 for black).
*/
@JvmName("jqasjptlrxoirlee")
public suspend fun backgroundColor(`value`: Output) {
this.backgroundColor = value
}
/**
* @param value An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("dutwgahbskdhycxc")
public suspend fun bodyConfig(`value`: Output) {
this.bodyConfig = value
}
/**
* @param value An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("xokjibmjvntviblu")
public suspend fun headerConfig(`value`: Output) {
this.headerConfig = value
}
/**
* @param value The URL of the image that appears on an in-app message banner.
*/
@JvmName("dthpkvswyevqkthd")
public suspend fun imageUrl(`value`: Output) {
this.imageUrl = value
}
/**
* @param value An object that contains configuration information about the primary button in an in-app message.
*/
@JvmName("bmiphepqwwjgphqr")
public suspend fun primaryBtn(`value`: Output) {
this.primaryBtn = value
}
/**
* @param value An object that contains configuration information about the secondary button in an in-app message.
*/
@JvmName("siftikqmliiudfcl")
public suspend fun secondaryBtn(`value`: Output) {
this.secondaryBtn = value
}
/**
* @param value The background color for an in-app message banner, expressed as a hex color code (such as #000000 for black).
*/
@JvmName("oltgskpkeqaesate")
public suspend fun backgroundColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundColor = mapped
}
/**
* @param value An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("agfthfmrgxsjdtnc")
public suspend fun bodyConfig(`value`: InAppTemplateBodyConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bodyConfig = mapped
}
/**
* @param argument An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("chvsmvxbxifsdgiq")
public suspend fun bodyConfig(argument: suspend InAppTemplateBodyConfigArgsBuilder.() -> Unit) {
val toBeMapped = InAppTemplateBodyConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.bodyConfig = mapped
}
/**
* @param value An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("dyilgrypiwpjurwb")
public suspend fun headerConfig(`value`: InAppTemplateHeaderConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.headerConfig = mapped
}
/**
* @param argument An object that contains configuration information about the header or title text of the in-app message.
*/
@JvmName("bentxrnmjljscjhy")
public suspend fun headerConfig(argument: suspend InAppTemplateHeaderConfigArgsBuilder.() -> Unit) {
val toBeMapped = InAppTemplateHeaderConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.headerConfig = mapped
}
/**
* @param value The URL of the image that appears on an in-app message banner.
*/
@JvmName("dlftsneubciuspty")
public suspend fun imageUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.imageUrl = mapped
}
/**
* @param value An object that contains configuration information about the primary button in an in-app message.
*/
@JvmName("lysqhdidbboslahd")
public suspend fun primaryBtn(`value`: InAppTemplateButtonConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryBtn = mapped
}
/**
* @param argument An object that contains configuration information about the primary button in an in-app message.
*/
@JvmName("hpajifkscehiuoey")
public suspend fun primaryBtn(argument: suspend InAppTemplateButtonConfigArgsBuilder.() -> Unit) {
val toBeMapped = InAppTemplateButtonConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.primaryBtn = mapped
}
/**
* @param value An object that contains configuration information about the secondary button in an in-app message.
*/
@JvmName("toagmjgylvojqrqj")
public suspend fun secondaryBtn(`value`: InAppTemplateButtonConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryBtn = mapped
}
/**
* @param argument An object that contains configuration information about the secondary button in an in-app message.
*/
@JvmName("vrrtqtgbdnkwalkw")
public suspend fun secondaryBtn(argument: suspend InAppTemplateButtonConfigArgsBuilder.() -> Unit) {
val toBeMapped = InAppTemplateButtonConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secondaryBtn = mapped
}
internal fun build(): InAppTemplateInAppMessageContentArgs = InAppTemplateInAppMessageContentArgs(
backgroundColor = backgroundColor,
bodyConfig = bodyConfig,
headerConfig = headerConfig,
imageUrl = imageUrl,
primaryBtn = primaryBtn,
secondaryBtn = secondaryBtn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy