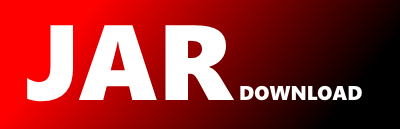
com.pulumi.awsnative.pipes.kotlin.outputs.GetPipeResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.pipes.kotlin.outputs
import com.pulumi.awsnative.pipes.kotlin.enums.PipeRequestedPipeState
import com.pulumi.awsnative.pipes.kotlin.enums.PipeState
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
/**
*
* @property arn The ARN of the pipe.
* @property creationTime The time the pipe was created.
* @property currentState The state the pipe is in.
* @property description A description of the pipe.
* @property desiredState The state the pipe should be in.
* @property enrichment The ARN of the enrichment resource.
* @property enrichmentParameters The parameters required to set up enrichment on your pipe.
* @property lastModifiedTime When the pipe was last updated, in [ISO-8601 format](https://docs.aws.amazon.com/https://www.w3.org/TR/NOTE-datetime) (YYYY-MM-DDThh:mm:ss.sTZD).
* @property logConfiguration The logging configuration settings for the pipe.
* @property roleArn The ARN of the role that allows the pipe to send data to the target.
* @property stateReason The reason the pipe is in its current state.
* @property tags The list of key-value pairs to associate with the pipe.
* @property target The ARN of the target resource.
*/
public data class GetPipeResult(
public val arn: String? = null,
public val creationTime: String? = null,
public val currentState: PipeState? = null,
public val description: String? = null,
public val desiredState: PipeRequestedPipeState? = null,
public val enrichment: String? = null,
public val enrichmentParameters: PipeEnrichmentParameters? = null,
public val lastModifiedTime: String? = null,
public val logConfiguration: PipeLogConfiguration? = null,
public val roleArn: String? = null,
public val stateReason: String? = null,
public val tags: Map? = null,
public val target: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.pipes.outputs.GetPipeResult): GetPipeResult =
GetPipeResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
creationTime = javaType.creationTime().map({ args0 -> args0 }).orElse(null),
currentState = javaType.currentState().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.pipes.kotlin.enums.PipeState.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
desiredState = javaType.desiredState().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.pipes.kotlin.enums.PipeRequestedPipeState.Companion.toKotlin(args0)
})
}).orElse(null),
enrichment = javaType.enrichment().map({ args0 -> args0 }).orElse(null),
enrichmentParameters = javaType.enrichmentParameters().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.pipes.kotlin.outputs.PipeEnrichmentParameters.Companion.toKotlin(args0)
})
}).orElse(null),
lastModifiedTime = javaType.lastModifiedTime().map({ args0 -> args0 }).orElse(null),
logConfiguration = javaType.logConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.pipes.kotlin.outputs.PipeLogConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
roleArn = javaType.roleArn().map({ args0 -> args0 }).orElse(null),
stateReason = javaType.stateReason().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
target = javaType.target().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy