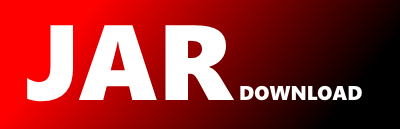
com.pulumi.awsnative.qbusiness.kotlin.DataSource.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.qbusiness.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.qbusiness.kotlin.enums.DataSourceStatus
import com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceDocumentEnrichmentConfiguration
import com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceVpcConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.enums.DataSourceStatus.Companion.toKotlin as dataSourceStatusToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceDocumentEnrichmentConfiguration.Companion.toKotlin as dataSourceDocumentEnrichmentConfigurationToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.outputs.DataSourceVpcConfiguration.Companion.toKotlin as dataSourceVpcConfigurationToKotlin
/**
* Builder for [DataSource].
*/
@PulumiTagMarker
public class DataSourceResourceBuilder internal constructor() {
public var name: String? = null
public var args: DataSourceArgs = DataSourceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DataSourceArgsBuilder.() -> Unit) {
val builder = DataSourceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): DataSource {
val builtJavaResource = com.pulumi.awsnative.qbusiness.DataSource(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return DataSource(builtJavaResource)
}
}
/**
* Definition of AWS::QBusiness::DataSource Resource Type
*/
public class DataSource internal constructor(
override val javaResource: com.pulumi.awsnative.qbusiness.DataSource,
) : KotlinCustomResource(javaResource, DataSourceMapper) {
/**
* The identifier of the Amazon Q Business application the data source will be attached to.
*/
public val applicationId: Output
get() = javaResource.applicationId().applyValue({ args0 -> args0 })
/**
* Configuration information to connect your data source repository to Amazon Q Business. Use this parameter to provide a JSON schema with configuration information specific to your data source connector.
* Each data source has a JSON schema provided by Amazon Q Business that you must use. For example, the Amazon S3 and Web Crawler connectors require the following JSON schemas:
* - [Amazon S3 JSON schema](https://docs.aws.amazon.com/amazonq/latest/qbusiness-ug/s3-api.html)
* - [Web Crawler JSON schema](https://docs.aws.amazon.com/amazonq/latest/qbusiness-ug/web-crawler-api.html)
* You can find configuration templates for your specific data source using the following steps:
* - Navigate to the [Supported connectors](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/connectors-list.html) page in the Amazon Q Business User Guide, and select the data source of your choice.
* - Then, from your specific data source connector page, select *Using the API* . You will find the JSON schema for your data source, including parameter descriptions, in this section.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::QBusiness::DataSource` for more information about the expected schema for this property.
*/
public val configuration: Output
get() = javaResource.configuration().applyValue({ args0 -> args0 })
/**
* The Unix timestamp when the Amazon Q Business data source was created.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of a data source in an Amazon Q Business application.
*/
public val dataSourceArn: Output
get() = javaResource.dataSourceArn().applyValue({ args0 -> args0 })
/**
* The identifier of the Amazon Q Business data source.
*/
public val dataSourceId: Output
get() = javaResource.dataSourceId().applyValue({ args0 -> args0 })
/**
* A description for the data source connector.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the Amazon Q Business data source.
*/
public val displayName: Output
get() = javaResource.displayName().applyValue({ args0 -> args0 })
/**
* Provides the configuration information for altering document metadata and content during the document ingestion process.
* For more information, see [Custom document enrichment](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/custom-document-enrichment.html) .
*/
public val documentEnrichmentConfiguration: Output?
get() = javaResource.documentEnrichmentConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
dataSourceDocumentEnrichmentConfigurationToKotlin(args0)
})
}).orElse(null)
})
/**
* The identifier of the index the data source is attached to.
*/
public val indexId: Output
get() = javaResource.indexId().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of an IAM role with permission to access the data source and required resources.
*/
public val roleArn: Output?
get() = javaResource.roleArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The status of the Amazon Q Business data source.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 ->
args0.let({ args0 ->
dataSourceStatusToKotlin(args0)
})
})
/**
* Sets the frequency for Amazon Q Business to check the documents in your data source repository and update your index. If you don't set a schedule, Amazon Q Business won't periodically update the index.
* Specify a `cron-` format schedule string or an empty string to indicate that the index is updated on demand. You can't specify the `Schedule` parameter when the `Type` parameter is set to `CUSTOM` . If you do, you receive a `ValidationException` exception.
*/
public val syncSchedule: Output?
get() = javaResource.syncSchedule().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of key-value pairs that identify or categorize the data source connector. You can also use tags to help control access to the data source connector. Tag keys and values can consist of Unicode letters, digits, white space, and any of the following symbols: _ . : / = + - @.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The type of the Amazon Q Business data source.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* The Unix timestamp when the Amazon Q Business data source was last updated.
*/
public val updatedAt: Output
get() = javaResource.updatedAt().applyValue({ args0 -> args0 })
/**
* Configuration information for an Amazon VPC (Virtual Private Cloud) to connect to your data source. For more information, see [Using Amazon VPC with Amazon Q Business connectors](https://docs.aws.amazon.com/amazonq/latest/business-use-dg/connector-vpc.html) .
*/
public val vpcConfiguration: Output?
get() = javaResource.vpcConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> dataSourceVpcConfigurationToKotlin(args0) })
}).orElse(null)
})
}
public object DataSourceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.qbusiness.DataSource::class == javaResource::class
override fun map(javaResource: Resource): DataSource = DataSource(
javaResource as
com.pulumi.awsnative.qbusiness.DataSource,
)
}
/**
* @see [DataSource].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [DataSource].
*/
public suspend fun dataSource(name: String, block: suspend DataSourceResourceBuilder.() -> Unit): DataSource {
val builder = DataSourceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [DataSource].
* @param name The _unique_ name of the resulting resource.
*/
public fun dataSource(name: String): DataSource {
val builder = DataSourceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy