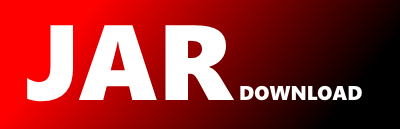
com.pulumi.awsnative.qbusiness.kotlin.WebExperience.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.qbusiness.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.qbusiness.kotlin.enums.WebExperienceSamplePromptsControlMode
import com.pulumi.awsnative.qbusiness.kotlin.enums.WebExperienceStatus
import com.pulumi.awsnative.qbusiness.kotlin.outputs.WebExperienceIdentityProviderConfiguration0Properties
import com.pulumi.awsnative.qbusiness.kotlin.outputs.WebExperienceIdentityProviderConfiguration1Properties
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.enums.WebExperienceSamplePromptsControlMode.Companion.toKotlin as webExperienceSamplePromptsControlModeToKotlin
import com.pulumi.awsnative.qbusiness.kotlin.enums.WebExperienceStatus.Companion.toKotlin as webExperienceStatusToKotlin
/**
* Builder for [WebExperience].
*/
@PulumiTagMarker
public class WebExperienceResourceBuilder internal constructor() {
public var name: String? = null
public var args: WebExperienceArgs = WebExperienceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend WebExperienceArgsBuilder.() -> Unit) {
val builder = WebExperienceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): WebExperience {
val builtJavaResource = com.pulumi.awsnative.qbusiness.WebExperience(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return WebExperience(builtJavaResource)
}
}
/**
* Definition of AWS::QBusiness::WebExperience Resource Type
*/
public class WebExperience internal constructor(
override val javaResource: com.pulumi.awsnative.qbusiness.WebExperience,
) : KotlinCustomResource(javaResource, WebExperienceMapper) {
/**
* The identifier of the Amazon Q Business web experience.
*/
public val applicationId: Output
get() = javaResource.applicationId().applyValue({ args0 -> args0 })
/**
* The Unix timestamp when the Amazon Q Business application was last updated.
*/
public val createdAt: Output
get() = javaResource.createdAt().applyValue({ args0 -> args0 })
/**
* The endpoint URLs for your Amazon Q Business web experience. The URLs are unique and fully hosted by AWS .
*/
public val defaultEndpoint: Output
get() = javaResource.defaultEndpoint().applyValue({ args0 -> args0 })
/**
* Provides information about the identity provider (IdP) used to authenticate end users of an Amazon Q Business web experience.
*/
public val identityProviderConfiguration:
Output>?
get() = javaResource.identityProviderConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.transform(
{ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.qbusiness.kotlin.outputs.WebExperienceIdentityProviderConfiguration0Properties.Companion.toKotlin(args0)
})
},
{ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.qbusiness.kotlin.outputs.WebExperienceIdentityProviderConfiguration1Properties.Companion.toKotlin(args0)
})
},
)
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) of the service role attached to your web experience.
* > You must provide this value if you're using IAM Identity Center to manage end user access to your application. If you're using legacy identity management to manage user access, you don't need to provide this value.
*/
public val roleArn: Output?
get() = javaResource.roleArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Determines whether sample prompts are enabled in the web experience for an end user.
*/
public val samplePromptsControlMode: Output?
get() = javaResource.samplePromptsControlMode().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> webExperienceSamplePromptsControlModeToKotlin(args0) })
}).orElse(null)
})
/**
* The status of your Amazon Q Business web experience.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 ->
args0.let({ args0 ->
webExperienceStatusToKotlin(args0)
})
})
/**
* A subtitle to personalize your Amazon Q Business web experience.
*/
public val subtitle: Output?
get() = javaResource.subtitle().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A list of key-value pairs that identify or categorize your Amazon Q Business web experience. You can also use tags to help control access to the web experience. Tag keys and values can consist of Unicode letters, digits, white space, and any of the following symbols: _ . : / = + - @.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The title for your Amazon Q Business web experience.
*/
public val title: Output?
get() = javaResource.title().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The Unix timestamp when your Amazon Q Business web experience was updated.
*/
public val updatedAt: Output
get() = javaResource.updatedAt().applyValue({ args0 -> args0 })
/**
* The Amazon Resource Name (ARN) of an Amazon Q Business web experience.
*/
public val webExperienceArn: Output
get() = javaResource.webExperienceArn().applyValue({ args0 -> args0 })
/**
* The identifier of your Amazon Q Business web experience.
*/
public val webExperienceId: Output
get() = javaResource.webExperienceId().applyValue({ args0 -> args0 })
/**
* A message in an Amazon Q Business web experience.
*/
public val welcomeMessage: Output?
get() = javaResource.welcomeMessage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object WebExperienceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.qbusiness.WebExperience::class == javaResource::class
override fun map(javaResource: Resource): WebExperience = WebExperience(
javaResource as
com.pulumi.awsnative.qbusiness.WebExperience,
)
}
/**
* @see [WebExperience].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [WebExperience].
*/
public suspend fun webExperience(
name: String,
block: suspend WebExperienceResourceBuilder.() -> Unit,
): WebExperience {
val builder = WebExperienceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [WebExperience].
* @param name The _unique_ name of the resulting resource.
*/
public fun webExperience(name: String): WebExperience {
val builder = WebExperienceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy