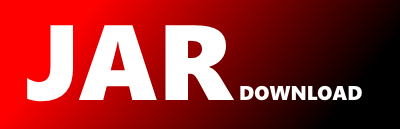
com.pulumi.awsnative.qldb.kotlin.StreamArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.qldb.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.qldb.StreamArgs.builder
import com.pulumi.awsnative.qldb.kotlin.inputs.StreamKinesisConfigurationArgs
import com.pulumi.awsnative.qldb.kotlin.inputs.StreamKinesisConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::QLDB::Stream.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property exclusiveEndTime The exclusive date and time that specifies when the stream ends. If you don't define this parameter, the stream runs indefinitely until you cancel it.
* The `ExclusiveEndTime` must be in `ISO 8601` date and time format and in Universal Coordinated Time (UTC). For example: `2019-06-13T21:36:34Z` .
* @property inclusiveStartTime The inclusive start date and time from which to start streaming journal data. This parameter must be in `ISO 8601` date and time format and in Universal Coordinated Time (UTC). For example: `2019-06-13T21:36:34Z` .
* The `InclusiveStartTime` cannot be in the future and must be before `ExclusiveEndTime` .
* If you provide an `InclusiveStartTime` that is before the ledger's `CreationDateTime` , QLDB effectively defaults it to the ledger's `CreationDateTime` .
* @property kinesisConfiguration The configuration settings of the Kinesis Data Streams destination for your stream request.
* @property ledgerName The name of the ledger.
* @property roleArn The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal stream to write data records to a Kinesis Data Streams resource.
* To pass a role to QLDB when requesting a journal stream, you must have permissions to perform the `iam:PassRole` action on the IAM role resource. This is required for all journal stream requests.
* @property streamName The name that you want to assign to the QLDB journal stream. User-defined names can help identify and indicate the purpose of a stream.
* Your stream name must be unique among other *active* streams for a given ledger. Stream names have the same naming constraints as ledger names, as defined in [Quotas in Amazon QLDB](https://docs.aws.amazon.com/qldb/latest/developerguide/limits.html#limits.naming) in the *Amazon QLDB Developer Guide* .
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class StreamArgs(
public val exclusiveEndTime: Output? = null,
public val inclusiveStartTime: Output? = null,
public val kinesisConfiguration: Output? = null,
public val ledgerName: Output? = null,
public val roleArn: Output? = null,
public val streamName: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.qldb.StreamArgs =
com.pulumi.awsnative.qldb.StreamArgs.builder()
.exclusiveEndTime(exclusiveEndTime?.applyValue({ args0 -> args0 }))
.inclusiveStartTime(inclusiveStartTime?.applyValue({ args0 -> args0 }))
.kinesisConfiguration(
kinesisConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.ledgerName(ledgerName?.applyValue({ args0 -> args0 }))
.roleArn(roleArn?.applyValue({ args0 -> args0 }))
.streamName(streamName?.applyValue({ args0 -> args0 }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [StreamArgs].
*/
@PulumiTagMarker
public class StreamArgsBuilder internal constructor() {
private var exclusiveEndTime: Output? = null
private var inclusiveStartTime: Output? = null
private var kinesisConfiguration: Output? = null
private var ledgerName: Output? = null
private var roleArn: Output? = null
private var streamName: Output? = null
private var tags: Output>? = null
/**
* @param value The exclusive date and time that specifies when the stream ends. If you don't define this parameter, the stream runs indefinitely until you cancel it.
* The `ExclusiveEndTime` must be in `ISO 8601` date and time format and in Universal Coordinated Time (UTC). For example: `2019-06-13T21:36:34Z` .
*/
@JvmName("jgyvscbeylvldqrt")
public suspend fun exclusiveEndTime(`value`: Output) {
this.exclusiveEndTime = value
}
/**
* @param value The inclusive start date and time from which to start streaming journal data. This parameter must be in `ISO 8601` date and time format and in Universal Coordinated Time (UTC). For example: `2019-06-13T21:36:34Z` .
* The `InclusiveStartTime` cannot be in the future and must be before `ExclusiveEndTime` .
* If you provide an `InclusiveStartTime` that is before the ledger's `CreationDateTime` , QLDB effectively defaults it to the ledger's `CreationDateTime` .
*/
@JvmName("rgrwehnqldmuxkbg")
public suspend fun inclusiveStartTime(`value`: Output) {
this.inclusiveStartTime = value
}
/**
* @param value The configuration settings of the Kinesis Data Streams destination for your stream request.
*/
@JvmName("hvjyjatsmtykiuug")
public suspend fun kinesisConfiguration(`value`: Output) {
this.kinesisConfiguration = value
}
/**
* @param value The name of the ledger.
*/
@JvmName("uugpylipcputfbec")
public suspend fun ledgerName(`value`: Output) {
this.ledgerName = value
}
/**
* @param value The Amazon Resource Name (ARN) of the IAM role that grants QLDB permissions for a journal stream to write data records to a Kinesis Data Streams resource.
* To pass a role to QLDB when requesting a journal stream, you must have permissions to perform the `iam:PassRole` action on the IAM role resource. This is required for all journal stream requests.
*/
@JvmName("ejdtidufgtrifuca")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The name that you want to assign to the QLDB journal stream. User-defined names can help identify and indicate the purpose of a stream.
* Your stream name must be unique among other *active* streams for a given ledger. Stream names have the same naming constraints as ledger names, as defined in [Quotas in Amazon QLDB](https://docs.aws.amazon.com/qldb/latest/developerguide/limits.html#limits.naming) in the *Amazon QLDB Developer Guide* .
*/
@JvmName("ethnxfplrtofjfnw")
public suspend fun streamName(`value`: Output) {
this.streamName = value
}
/**
* @param value An array of key-value pairs to apply to this resource.
*/
@JvmName("baahctsrgeugoxbx")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("qtsfwybejbsoagjp")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values An array of key-value pairs to apply to this resource.
*/
@JvmName("uhbnxmyiwtrlnvbb")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy