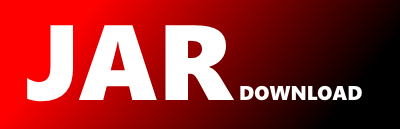
com.pulumi.awsnative.quicksight.kotlin.Template.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateResourcePermission
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateSourceEntity
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateValidationStrategy
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateVersion
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateVersionDefinition
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateResourcePermission.Companion.toKotlin as templateResourcePermissionToKotlin
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateSourceEntity.Companion.toKotlin as templateSourceEntityToKotlin
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateValidationStrategy.Companion.toKotlin as templateValidationStrategyToKotlin
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateVersion.Companion.toKotlin as templateVersionToKotlin
import com.pulumi.awsnative.quicksight.kotlin.outputs.TemplateVersionDefinition.Companion.toKotlin as templateVersionDefinitionToKotlin
/**
* Builder for [Template].
*/
@PulumiTagMarker
public class TemplateResourceBuilder internal constructor() {
public var name: String? = null
public var args: TemplateArgs = TemplateArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend TemplateArgsBuilder.() -> Unit) {
val builder = TemplateArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Template {
val builtJavaResource = com.pulumi.awsnative.quicksight.Template(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Template(builtJavaResource)
}
}
/**
* Definition of the AWS::QuickSight::Template Resource Type.
*/
public class Template internal constructor(
override val javaResource: com.pulumi.awsnative.quicksight.Template,
) : KotlinCustomResource(javaResource, TemplateMapper) {
/**
* The Amazon Resource Name (ARN) of the template.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The ID for the AWS account that the group is in. You use the ID for the AWS account that contains your Amazon QuickSight account.
*/
public val awsAccountId: Output
get() = javaResource.awsAccountId().applyValue({ args0 -> args0 })
/**
* Time when this was created.
*/
public val createdTime: Output
get() = javaResource.createdTime().applyValue({ args0 -> args0 })
public val definition: Output?
get() = javaResource.definition().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
templateVersionDefinitionToKotlin(args0)
})
}).orElse(null)
})
/**
* Time when this was last updated.
*/
public val lastUpdatedTime: Output
get() = javaResource.lastUpdatedTime().applyValue({ args0 -> args0 })
/**
* A display name for the template.
*/
public val name: Output?
get() = javaResource.name().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A list of resource permissions to be set on the template.
*/
public val permissions: Output>?
get() = javaResource.permissions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> templateResourcePermissionToKotlin(args0) })
})
}).orElse(null)
})
/**
* The entity that you are using as a source when you create the template. In `SourceEntity` , you specify the type of object you're using as source: `SourceTemplate` for a template or `SourceAnalysis` for an analysis. Both of these require an Amazon Resource Name (ARN). For `SourceTemplate` , specify the ARN of the source template. For `SourceAnalysis` , specify the ARN of the source analysis. The `SourceTemplate` ARN can contain any AWS account and any Amazon QuickSight-supported AWS Region .
* Use the `DataSetReferences` entity within `SourceTemplate` or `SourceAnalysis` to list the replacement datasets for the placeholders listed in the original. The schema in each dataset must match its placeholder.
* Either a `SourceEntity` or a `Definition` must be provided in order for the request to be valid.
*/
public val sourceEntity: Output?
get() = javaResource.sourceEntity().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
templateSourceEntityToKotlin(args0)
})
}).orElse(null)
})
/**
* Contains a map of the key-value pairs for the resource tag or tags assigned to the resource.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* An ID for the template that you want to create. This template is unique per AWS Region ; in each AWS account.
*/
public val templateId: Output
get() = javaResource.templateId().applyValue({ args0 -> args0 })
/**
* The option to relax the validation that is required to create and update analyses, dashboards, and templates with definition objects. When you set this value to `LENIENT` , validation is skipped for specific errors.
*/
public val validationStrategy: Output?
get() = javaResource.validationStrategy().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> templateValidationStrategyToKotlin(args0) })
}).orElse(null)
})
public val version: Output
get() = javaResource.version().applyValue({ args0 ->
args0.let({ args0 ->
templateVersionToKotlin(args0)
})
})
/**
* A description of the current template version being created. This API operation creates the first version of the template. Every time `UpdateTemplate` is called, a new version is created. Each version of the template maintains a description of the version in the `VersionDescription` field.
*/
public val versionDescription: Output?
get() = javaResource.versionDescription().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object TemplateMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.quicksight.Template::class == javaResource::class
override fun map(javaResource: Resource): Template = Template(
javaResource as
com.pulumi.awsnative.quicksight.Template,
)
}
/**
* @see [Template].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Template].
*/
public suspend fun template(name: String, block: suspend TemplateResourceBuilder.() -> Unit): Template {
val builder = TemplateResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Template].
* @param name The _unique_ name of the resulting resource.
*/
public fun template(name: String): Template {
val builder = TemplateResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy