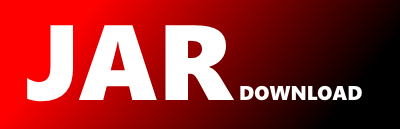
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisGaugeChartConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisGaugeChartConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dataLabels The data label configuration of a `GaugeChartVisual` .
* @property fieldWells The field well configuration of a `GaugeChartVisual` .
* @property gaugeChartOptions The options that determine the presentation of the `GaugeChartVisual` .
* @property tooltipOptions The tooltip configuration of a `GaugeChartVisual` .
* @property visualPalette The visual palette configuration of a `GaugeChartVisual` .
*/
public data class AnalysisGaugeChartConfigurationArgs(
public val dataLabels: Output? = null,
public val fieldWells: Output? = null,
public val gaugeChartOptions: Output? = null,
public val tooltipOptions: Output? = null,
public val visualPalette: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisGaugeChartConfigurationArgs = com.pulumi.awsnative.quicksight.inputs.AnalysisGaugeChartConfigurationArgs.builder()
.dataLabels(dataLabels?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fieldWells(fieldWells?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gaugeChartOptions(gaugeChartOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tooltipOptions(tooltipOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.visualPalette(visualPalette?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalysisGaugeChartConfigurationArgs].
*/
@PulumiTagMarker
public class AnalysisGaugeChartConfigurationArgsBuilder internal constructor() {
private var dataLabels: Output? = null
private var fieldWells: Output? = null
private var gaugeChartOptions: Output? = null
private var tooltipOptions: Output? = null
private var visualPalette: Output? = null
/**
* @param value The data label configuration of a `GaugeChartVisual` .
*/
@JvmName("hstyuxneomaymrdu")
public suspend fun dataLabels(`value`: Output) {
this.dataLabels = value
}
/**
* @param value The field well configuration of a `GaugeChartVisual` .
*/
@JvmName("wehbubdrjckorbyt")
public suspend fun fieldWells(`value`: Output) {
this.fieldWells = value
}
/**
* @param value The options that determine the presentation of the `GaugeChartVisual` .
*/
@JvmName("ykuavsstmwunhsns")
public suspend fun gaugeChartOptions(`value`: Output) {
this.gaugeChartOptions = value
}
/**
* @param value The tooltip configuration of a `GaugeChartVisual` .
*/
@JvmName("vqjlmcwwoeoyxmlm")
public suspend fun tooltipOptions(`value`: Output) {
this.tooltipOptions = value
}
/**
* @param value The visual palette configuration of a `GaugeChartVisual` .
*/
@JvmName("sgqdwmhknjbpivpt")
public suspend fun visualPalette(`value`: Output) {
this.visualPalette = value
}
/**
* @param value The data label configuration of a `GaugeChartVisual` .
*/
@JvmName("ycwrnybncaxgbbom")
public suspend fun dataLabels(`value`: AnalysisDataLabelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataLabels = mapped
}
/**
* @param argument The data label configuration of a `GaugeChartVisual` .
*/
@JvmName("qbyumtprhkwgnkak")
public suspend fun dataLabels(argument: suspend AnalysisDataLabelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisDataLabelOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.dataLabels = mapped
}
/**
* @param value The field well configuration of a `GaugeChartVisual` .
*/
@JvmName("xbxhtiutomsjoadq")
public suspend fun fieldWells(`value`: AnalysisGaugeChartFieldWellsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldWells = mapped
}
/**
* @param argument The field well configuration of a `GaugeChartVisual` .
*/
@JvmName("emnexsqwxvxmhtva")
public suspend fun fieldWells(argument: suspend AnalysisGaugeChartFieldWellsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisGaugeChartFieldWellsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fieldWells = mapped
}
/**
* @param value The options that determine the presentation of the `GaugeChartVisual` .
*/
@JvmName("dharaogavoqwgmqq")
public suspend fun gaugeChartOptions(`value`: AnalysisGaugeChartOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gaugeChartOptions = mapped
}
/**
* @param argument The options that determine the presentation of the `GaugeChartVisual` .
*/
@JvmName("dnvbpsdkxdxyhdmq")
public suspend fun gaugeChartOptions(argument: suspend AnalysisGaugeChartOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisGaugeChartOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.gaugeChartOptions = mapped
}
/**
* @param value The tooltip configuration of a `GaugeChartVisual` .
*/
@JvmName("oqvngjhrugkifpnb")
public suspend fun tooltipOptions(`value`: AnalysisTooltipOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tooltipOptions = mapped
}
/**
* @param argument The tooltip configuration of a `GaugeChartVisual` .
*/
@JvmName("fjxrxkmbwrlnexyg")
public suspend fun tooltipOptions(argument: suspend AnalysisTooltipOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisTooltipOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.tooltipOptions = mapped
}
/**
* @param value The visual palette configuration of a `GaugeChartVisual` .
*/
@JvmName("jdnjoqpcyjlvvbfq")
public suspend fun visualPalette(`value`: AnalysisVisualPaletteArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visualPalette = mapped
}
/**
* @param argument The visual palette configuration of a `GaugeChartVisual` .
*/
@JvmName("kwbkjhodqcvbmamq")
public suspend fun visualPalette(argument: suspend AnalysisVisualPaletteArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisVisualPaletteArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.visualPalette = mapped
}
internal fun build(): AnalysisGaugeChartConfigurationArgs = AnalysisGaugeChartConfigurationArgs(
dataLabels = dataLabels,
fieldWells = fieldWells,
gaugeChartOptions = gaugeChartOptions,
tooltipOptions = tooltipOptions,
visualPalette = visualPalette,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy