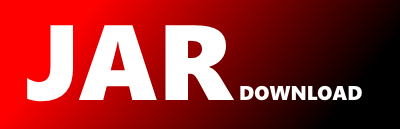
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisNumberDisplayFormatConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisNumberDisplayFormatConfigurationArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisNumberScale
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property decimalPlacesConfiguration The option that determines the decimal places configuration.
* @property negativeValueConfiguration The options that determine the negative value configuration.
* @property nullValueFormatConfiguration The options that determine the null value format configuration.
* @property numberScale Determines the number scale value of the number format.
* @property prefix Determines the prefix value of the number format.
* @property separatorConfiguration The options that determine the numeric separator configuration.
* @property suffix Determines the suffix value of the number format.
*/
public data class AnalysisNumberDisplayFormatConfigurationArgs(
public val decimalPlacesConfiguration: Output? = null,
public val negativeValueConfiguration: Output? = null,
public val nullValueFormatConfiguration: Output? = null,
public val numberScale: Output? = null,
public val prefix: Output? = null,
public val separatorConfiguration: Output? = null,
public val suffix: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisNumberDisplayFormatConfigurationArgs =
com.pulumi.awsnative.quicksight.inputs.AnalysisNumberDisplayFormatConfigurationArgs.builder()
.decimalPlacesConfiguration(
decimalPlacesConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.negativeValueConfiguration(
negativeValueConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.nullValueFormatConfiguration(
nullValueFormatConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.numberScale(numberScale?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.separatorConfiguration(
separatorConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.suffix(suffix?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AnalysisNumberDisplayFormatConfigurationArgs].
*/
@PulumiTagMarker
public class AnalysisNumberDisplayFormatConfigurationArgsBuilder internal constructor() {
private var decimalPlacesConfiguration: Output? = null
private var negativeValueConfiguration: Output? = null
private var nullValueFormatConfiguration: Output? = null
private var numberScale: Output? = null
private var prefix: Output? = null
private var separatorConfiguration: Output? = null
private var suffix: Output? = null
/**
* @param value The option that determines the decimal places configuration.
*/
@JvmName("ppphiauhxqannpqj")
public suspend fun decimalPlacesConfiguration(`value`: Output) {
this.decimalPlacesConfiguration = value
}
/**
* @param value The options that determine the negative value configuration.
*/
@JvmName("lsxsqadcfepukkwr")
public suspend fun negativeValueConfiguration(`value`: Output) {
this.negativeValueConfiguration = value
}
/**
* @param value The options that determine the null value format configuration.
*/
@JvmName("bfeqawkxroqnfmus")
public suspend fun nullValueFormatConfiguration(`value`: Output) {
this.nullValueFormatConfiguration = value
}
/**
* @param value Determines the number scale value of the number format.
*/
@JvmName("lytvlcuttxbqntnr")
public suspend fun numberScale(`value`: Output) {
this.numberScale = value
}
/**
* @param value Determines the prefix value of the number format.
*/
@JvmName("kjlfsthlwlyloefd")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value The options that determine the numeric separator configuration.
*/
@JvmName("jqkswcxaatlgugal")
public suspend fun separatorConfiguration(`value`: Output) {
this.separatorConfiguration = value
}
/**
* @param value Determines the suffix value of the number format.
*/
@JvmName("lqphylfkyqklngia")
public suspend fun suffix(`value`: Output) {
this.suffix = value
}
/**
* @param value The option that determines the decimal places configuration.
*/
@JvmName("llgfcklrkncomeyd")
public suspend fun decimalPlacesConfiguration(`value`: AnalysisDecimalPlacesConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.decimalPlacesConfiguration = mapped
}
/**
* @param argument The option that determines the decimal places configuration.
*/
@JvmName("gmgudprqwncqiilu")
public suspend fun decimalPlacesConfiguration(argument: suspend AnalysisDecimalPlacesConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisDecimalPlacesConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.decimalPlacesConfiguration = mapped
}
/**
* @param value The options that determine the negative value configuration.
*/
@JvmName("yrmlyhefogucpqym")
public suspend fun negativeValueConfiguration(`value`: AnalysisNegativeValueConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.negativeValueConfiguration = mapped
}
/**
* @param argument The options that determine the negative value configuration.
*/
@JvmName("mfbnfdxgpfdnwhol")
public suspend fun negativeValueConfiguration(argument: suspend AnalysisNegativeValueConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisNegativeValueConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.negativeValueConfiguration = mapped
}
/**
* @param value The options that determine the null value format configuration.
*/
@JvmName("ittcfwpvljfqkdrw")
public suspend fun nullValueFormatConfiguration(`value`: AnalysisNullValueFormatConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nullValueFormatConfiguration = mapped
}
/**
* @param argument The options that determine the null value format configuration.
*/
@JvmName("xwujwkwgplkflpjw")
public suspend fun nullValueFormatConfiguration(argument: suspend AnalysisNullValueFormatConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisNullValueFormatConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.nullValueFormatConfiguration = mapped
}
/**
* @param value Determines the number scale value of the number format.
*/
@JvmName("bnwsinoirqdsjwho")
public suspend fun numberScale(`value`: AnalysisNumberScale?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.numberScale = mapped
}
/**
* @param value Determines the prefix value of the number format.
*/
@JvmName("psdhlosxqvfnewbt")
public suspend fun prefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.prefix = mapped
}
/**
* @param value The options that determine the numeric separator configuration.
*/
@JvmName("iycsssnibxcqnago")
public suspend fun separatorConfiguration(`value`: AnalysisNumericSeparatorConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.separatorConfiguration = mapped
}
/**
* @param argument The options that determine the numeric separator configuration.
*/
@JvmName("ukxbutnkbtraptet")
public suspend fun separatorConfiguration(argument: suspend AnalysisNumericSeparatorConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisNumericSeparatorConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.separatorConfiguration = mapped
}
/**
* @param value Determines the suffix value of the number format.
*/
@JvmName("hwnjqisqakbnxbrp")
public suspend fun suffix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.suffix = mapped
}
internal fun build(): AnalysisNumberDisplayFormatConfigurationArgs =
AnalysisNumberDisplayFormatConfigurationArgs(
decimalPlacesConfiguration = decimalPlacesConfiguration,
negativeValueConfiguration = negativeValueConfiguration,
nullValueFormatConfiguration = nullValueFormatConfiguration,
numberScale = numberScale,
prefix = prefix,
separatorConfiguration = separatorConfiguration,
suffix = suffix,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy