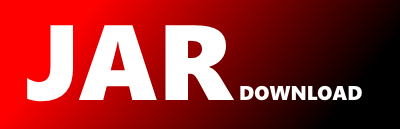
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisNumericalAggregationFunctionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisNumericalAggregationFunctionArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisSimpleNumericalAggregationFunction
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property percentileAggregation An aggregation based on the percentile of values in a dimension or measure.
* @property simpleNumericalAggregation Built-in aggregation functions for numerical values.
* - `SUM` : The sum of a dimension or measure.
* - `AVERAGE` : The average of a dimension or measure.
* - `MIN` : The minimum value of a dimension or measure.
* - `MAX` : The maximum value of a dimension or measure.
* - `COUNT` : The count of a dimension or measure.
* - `DISTINCT_COUNT` : The count of distinct values in a dimension or measure.
* - `VAR` : The variance of a dimension or measure.
* - `VARP` : The partitioned variance of a dimension or measure.
* - `STDEV` : The standard deviation of a dimension or measure.
* - `STDEVP` : The partitioned standard deviation of a dimension or measure.
* - `MEDIAN` : The median value of a dimension or measure.
*/
public data class AnalysisNumericalAggregationFunctionArgs(
public val percentileAggregation: Output? = null,
public val simpleNumericalAggregation: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisNumericalAggregationFunctionArgs =
com.pulumi.awsnative.quicksight.inputs.AnalysisNumericalAggregationFunctionArgs.builder()
.percentileAggregation(
percentileAggregation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.simpleNumericalAggregation(
simpleNumericalAggregation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [AnalysisNumericalAggregationFunctionArgs].
*/
@PulumiTagMarker
public class AnalysisNumericalAggregationFunctionArgsBuilder internal constructor() {
private var percentileAggregation: Output? = null
private var simpleNumericalAggregation: Output? = null
/**
* @param value An aggregation based on the percentile of values in a dimension or measure.
*/
@JvmName("qgxmtvudkankjcwg")
public suspend fun percentileAggregation(`value`: Output) {
this.percentileAggregation = value
}
/**
* @param value Built-in aggregation functions for numerical values.
* - `SUM` : The sum of a dimension or measure.
* - `AVERAGE` : The average of a dimension or measure.
* - `MIN` : The minimum value of a dimension or measure.
* - `MAX` : The maximum value of a dimension or measure.
* - `COUNT` : The count of a dimension or measure.
* - `DISTINCT_COUNT` : The count of distinct values in a dimension or measure.
* - `VAR` : The variance of a dimension or measure.
* - `VARP` : The partitioned variance of a dimension or measure.
* - `STDEV` : The standard deviation of a dimension or measure.
* - `STDEVP` : The partitioned standard deviation of a dimension or measure.
* - `MEDIAN` : The median value of a dimension or measure.
*/
@JvmName("eipdwlaunciyrodx")
public suspend fun simpleNumericalAggregation(`value`: Output) {
this.simpleNumericalAggregation = value
}
/**
* @param value An aggregation based on the percentile of values in a dimension or measure.
*/
@JvmName("yrjulvplgjfgcaxd")
public suspend fun percentileAggregation(`value`: AnalysisPercentileAggregationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.percentileAggregation = mapped
}
/**
* @param argument An aggregation based on the percentile of values in a dimension or measure.
*/
@JvmName("oqfvlyfepgvwntka")
public suspend fun percentileAggregation(argument: suspend AnalysisPercentileAggregationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisPercentileAggregationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.percentileAggregation = mapped
}
/**
* @param value Built-in aggregation functions for numerical values.
* - `SUM` : The sum of a dimension or measure.
* - `AVERAGE` : The average of a dimension or measure.
* - `MIN` : The minimum value of a dimension or measure.
* - `MAX` : The maximum value of a dimension or measure.
* - `COUNT` : The count of a dimension or measure.
* - `DISTINCT_COUNT` : The count of distinct values in a dimension or measure.
* - `VAR` : The variance of a dimension or measure.
* - `VARP` : The partitioned variance of a dimension or measure.
* - `STDEV` : The standard deviation of a dimension or measure.
* - `STDEVP` : The partitioned standard deviation of a dimension or measure.
* - `MEDIAN` : The median value of a dimension or measure.
*/
@JvmName("dxqnfbpgqiqqwqxg")
public suspend fun simpleNumericalAggregation(`value`: AnalysisSimpleNumericalAggregationFunction?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.simpleNumericalAggregation = mapped
}
internal fun build(): AnalysisNumericalAggregationFunctionArgs =
AnalysisNumericalAggregationFunctionArgs(
percentileAggregation = percentileAggregation,
simpleNumericalAggregation = simpleNumericalAggregation,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy