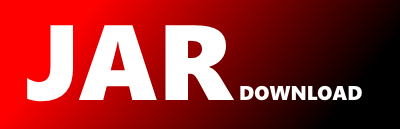
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisPanelConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisPanelConfigurationArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisPanelBorderStyle
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backgroundColor Sets the background color for each panel.
* @property backgroundVisibility Determines whether or not a background for each small multiples panel is rendered.
* @property borderColor Sets the line color of panel borders.
* @property borderStyle Sets the line style of panel borders.
* @property borderThickness String based length that is composed of value and unit in px
* @property borderVisibility Determines whether or not each panel displays a border.
* @property gutterSpacing String based length that is composed of value and unit in px
* @property gutterVisibility Determines whether or not negative space between sibling panels is rendered.
* @property title Configures the title display within each small multiples panel.
*/
public data class AnalysisPanelConfigurationArgs(
public val backgroundColor: Output? = null,
public val backgroundVisibility: Output? = null,
public val borderColor: Output? = null,
public val borderStyle: Output? = null,
public val borderThickness: Output? = null,
public val borderVisibility: Output? = null,
public val gutterSpacing: Output? = null,
public val gutterVisibility: Output? = null,
public val title: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisPanelConfigurationArgs =
com.pulumi.awsnative.quicksight.inputs.AnalysisPanelConfigurationArgs.builder()
.backgroundColor(backgroundColor?.applyValue({ args0 -> args0 }))
.backgroundVisibility(
backgroundVisibility?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.borderColor(borderColor?.applyValue({ args0 -> args0 }))
.borderStyle(borderStyle?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.borderThickness(borderThickness?.applyValue({ args0 -> args0 }))
.borderVisibility(borderVisibility?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gutterSpacing(gutterSpacing?.applyValue({ args0 -> args0 }))
.gutterVisibility(gutterVisibility?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.title(title?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalysisPanelConfigurationArgs].
*/
@PulumiTagMarker
public class AnalysisPanelConfigurationArgsBuilder internal constructor() {
private var backgroundColor: Output? = null
private var backgroundVisibility: Output? = null
private var borderColor: Output? = null
private var borderStyle: Output? = null
private var borderThickness: Output? = null
private var borderVisibility: Output? = null
private var gutterSpacing: Output? = null
private var gutterVisibility: Output? = null
private var title: Output? = null
/**
* @param value Sets the background color for each panel.
*/
@JvmName("qbvurvybdouhliij")
public suspend fun backgroundColor(`value`: Output) {
this.backgroundColor = value
}
/**
* @param value Determines whether or not a background for each small multiples panel is rendered.
*/
@JvmName("gxfovnbwudnbuats")
public suspend fun backgroundVisibility(`value`: Output) {
this.backgroundVisibility = value
}
/**
* @param value Sets the line color of panel borders.
*/
@JvmName("ecpbrpyjaeqldsie")
public suspend fun borderColor(`value`: Output) {
this.borderColor = value
}
/**
* @param value Sets the line style of panel borders.
*/
@JvmName("cvvdvuwmbyflpcqn")
public suspend fun borderStyle(`value`: Output) {
this.borderStyle = value
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("vupoaubsqnqyjdny")
public suspend fun borderThickness(`value`: Output) {
this.borderThickness = value
}
/**
* @param value Determines whether or not each panel displays a border.
*/
@JvmName("whwketmggnshuebw")
public suspend fun borderVisibility(`value`: Output) {
this.borderVisibility = value
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("peqwfdtwoyvthedu")
public suspend fun gutterSpacing(`value`: Output) {
this.gutterSpacing = value
}
/**
* @param value Determines whether or not negative space between sibling panels is rendered.
*/
@JvmName("xppvxmmmwbhggglk")
public suspend fun gutterVisibility(`value`: Output) {
this.gutterVisibility = value
}
/**
* @param value Configures the title display within each small multiples panel.
*/
@JvmName("jvnowvmruscvausf")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value Sets the background color for each panel.
*/
@JvmName("fjsixypcjkbrbcus")
public suspend fun backgroundColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundColor = mapped
}
/**
* @param value Determines whether or not a background for each small multiples panel is rendered.
*/
@JvmName("vcauykpqjwjxcjch")
public suspend fun backgroundVisibility(`value`: AnalysisVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundVisibility = mapped
}
/**
* @param value Sets the line color of panel borders.
*/
@JvmName("ietsutgwqcjlgjny")
public suspend fun borderColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.borderColor = mapped
}
/**
* @param value Sets the line style of panel borders.
*/
@JvmName("maiudsrvrffnkbsh")
public suspend fun borderStyle(`value`: AnalysisPanelBorderStyle?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.borderStyle = mapped
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("cneygpgcuqdhjbif")
public suspend fun borderThickness(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.borderThickness = mapped
}
/**
* @param value Determines whether or not each panel displays a border.
*/
@JvmName("uwynwohefbmlkpwd")
public suspend fun borderVisibility(`value`: AnalysisVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.borderVisibility = mapped
}
/**
* @param value String based length that is composed of value and unit in px
*/
@JvmName("txdfssapmllkdmgi")
public suspend fun gutterSpacing(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gutterSpacing = mapped
}
/**
* @param value Determines whether or not negative space between sibling panels is rendered.
*/
@JvmName("jmxrbgfasnborjuw")
public suspend fun gutterVisibility(`value`: AnalysisVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gutterVisibility = mapped
}
/**
* @param value Configures the title display within each small multiples panel.
*/
@JvmName("npxywfdergpkykbm")
public suspend fun title(`value`: AnalysisPanelTitleOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.title = mapped
}
/**
* @param argument Configures the title display within each small multiples panel.
*/
@JvmName("pskyyvwjgojseacu")
public suspend fun title(argument: suspend AnalysisPanelTitleOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisPanelTitleOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.title = mapped
}
internal fun build(): AnalysisPanelConfigurationArgs = AnalysisPanelConfigurationArgs(
backgroundColor = backgroundColor,
backgroundVisibility = backgroundVisibility,
borderColor = borderColor,
borderStyle = borderStyle,
borderThickness = borderThickness,
borderVisibility = borderVisibility,
gutterSpacing = gutterSpacing,
gutterVisibility = gutterVisibility,
title = title,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy