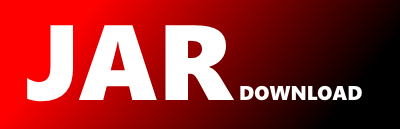
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisParameterDropDownControlArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisParameterDropDownControlArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisSheetControlListType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cascadingControlConfiguration The values that are displayed in a control can be configured to only show values that are valid based on what's selected in other controls.
* @property displayOptions The display options of a control.
* @property parameterControlId The ID of the `ParameterDropDownControl` .
* @property selectableValues A list of selectable values that are used in a control.
* @property sourceParameterName The source parameter name of the `ParameterDropDownControl` .
* @property title The title of the `ParameterDropDownControl` .
* @property type The type parameter name of the `ParameterDropDownControl` .
*/
public data class AnalysisParameterDropDownControlArgs(
public val cascadingControlConfiguration: Output? =
null,
public val displayOptions: Output? = null,
public val parameterControlId: Output,
public val selectableValues: Output? = null,
public val sourceParameterName: Output,
public val title: Output,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisParameterDropDownControlArgs = com.pulumi.awsnative.quicksight.inputs.AnalysisParameterDropDownControlArgs.builder()
.cascadingControlConfiguration(
cascadingControlConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.displayOptions(displayOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.parameterControlId(parameterControlId.applyValue({ args0 -> args0 }))
.selectableValues(selectableValues?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sourceParameterName(sourceParameterName.applyValue({ args0 -> args0 }))
.title(title.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalysisParameterDropDownControlArgs].
*/
@PulumiTagMarker
public class AnalysisParameterDropDownControlArgsBuilder internal constructor() {
private var cascadingControlConfiguration: Output? =
null
private var displayOptions: Output? = null
private var parameterControlId: Output? = null
private var selectableValues: Output? = null
private var sourceParameterName: Output? = null
private var title: Output? = null
private var type: Output? = null
/**
* @param value The values that are displayed in a control can be configured to only show values that are valid based on what's selected in other controls.
*/
@JvmName("otrlhtxuvveylhyd")
public suspend fun cascadingControlConfiguration(`value`: Output) {
this.cascadingControlConfiguration = value
}
/**
* @param value The display options of a control.
*/
@JvmName("aolvabgfluvnwrwj")
public suspend fun displayOptions(`value`: Output) {
this.displayOptions = value
}
/**
* @param value The ID of the `ParameterDropDownControl` .
*/
@JvmName("reujbrbyowrqyefu")
public suspend fun parameterControlId(`value`: Output) {
this.parameterControlId = value
}
/**
* @param value A list of selectable values that are used in a control.
*/
@JvmName("okvbikagjvmttkrk")
public suspend fun selectableValues(`value`: Output) {
this.selectableValues = value
}
/**
* @param value The source parameter name of the `ParameterDropDownControl` .
*/
@JvmName("mdkgmebwgtbolfkc")
public suspend fun sourceParameterName(`value`: Output) {
this.sourceParameterName = value
}
/**
* @param value The title of the `ParameterDropDownControl` .
*/
@JvmName("vqrjnmcmjosneyio")
public suspend fun title(`value`: Output) {
this.title = value
}
/**
* @param value The type parameter name of the `ParameterDropDownControl` .
*/
@JvmName("nmyduevcbrxwkejm")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The values that are displayed in a control can be configured to only show values that are valid based on what's selected in other controls.
*/
@JvmName("cndfkyqcodqvwmdj")
public suspend fun cascadingControlConfiguration(`value`: AnalysisCascadingControlConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cascadingControlConfiguration = mapped
}
/**
* @param argument The values that are displayed in a control can be configured to only show values that are valid based on what's selected in other controls.
*/
@JvmName("islaycajwyctfrhg")
public suspend fun cascadingControlConfiguration(argument: suspend AnalysisCascadingControlConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisCascadingControlConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cascadingControlConfiguration = mapped
}
/**
* @param value The display options of a control.
*/
@JvmName("wfmqhtauknkcfonp")
public suspend fun displayOptions(`value`: AnalysisDropDownControlDisplayOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.displayOptions = mapped
}
/**
* @param argument The display options of a control.
*/
@JvmName("moeblnelisuvqsjc")
public suspend fun displayOptions(argument: suspend AnalysisDropDownControlDisplayOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisDropDownControlDisplayOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.displayOptions = mapped
}
/**
* @param value The ID of the `ParameterDropDownControl` .
*/
@JvmName("mmkfjoyxfqsoygpm")
public suspend fun parameterControlId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.parameterControlId = mapped
}
/**
* @param value A list of selectable values that are used in a control.
*/
@JvmName("bclbtxulnxnufslq")
public suspend fun selectableValues(`value`: AnalysisParameterSelectableValuesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selectableValues = mapped
}
/**
* @param argument A list of selectable values that are used in a control.
*/
@JvmName("tekktwyedypkdglq")
public suspend fun selectableValues(argument: suspend AnalysisParameterSelectableValuesArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisParameterSelectableValuesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.selectableValues = mapped
}
/**
* @param value The source parameter name of the `ParameterDropDownControl` .
*/
@JvmName("pccapwuwwoexbpmb")
public suspend fun sourceParameterName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sourceParameterName = mapped
}
/**
* @param value The title of the `ParameterDropDownControl` .
*/
@JvmName("ejmregikygjhofdo")
public suspend fun title(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.title = mapped
}
/**
* @param value The type parameter name of the `ParameterDropDownControl` .
*/
@JvmName("wjbmrusqleodroeu")
public suspend fun type(`value`: AnalysisSheetControlListType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): AnalysisParameterDropDownControlArgs = AnalysisParameterDropDownControlArgs(
cascadingControlConfiguration = cascadingControlConfiguration,
displayOptions = displayOptions,
parameterControlId = parameterControlId ?: throw PulumiNullFieldException("parameterControlId"),
selectableValues = selectableValues,
sourceParameterName = sourceParameterName ?: throw PulumiNullFieldException("sourceParameterName"),
title = title ?: throw PulumiNullFieldException("title"),
type = type,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy