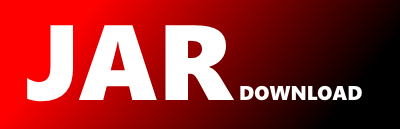
com.pulumi.awsnative.quicksight.kotlin.inputs.AnalysisTableCellStyleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.AnalysisTableCellStyleArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisHorizontalTextAlignment
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisTextWrap
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisVerticalTextAlignment
import com.pulumi.awsnative.quicksight.kotlin.enums.AnalysisVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backgroundColor The background color for the table cells.
* @property border The borders for the table cells.
* @property fontConfiguration The font configuration of the table cells.
* @property height The height color for the table cells.
* @property horizontalTextAlignment The horizontal text alignment (left, center, right, auto) for the table cells.
* @property textWrap The text wrap (none, wrap) for the table cells.
* @property verticalTextAlignment The vertical text alignment (top, middle, bottom) for the table cells.
* @property visibility The visibility of the table cells.
*/
public data class AnalysisTableCellStyleArgs(
public val backgroundColor: Output? = null,
public val border: Output? = null,
public val fontConfiguration: Output? = null,
public val height: Output? = null,
public val horizontalTextAlignment: Output? = null,
public val textWrap: Output? = null,
public val verticalTextAlignment: Output? = null,
public val visibility: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.AnalysisTableCellStyleArgs =
com.pulumi.awsnative.quicksight.inputs.AnalysisTableCellStyleArgs.builder()
.backgroundColor(backgroundColor?.applyValue({ args0 -> args0 }))
.border(border?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fontConfiguration(fontConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.height(height?.applyValue({ args0 -> args0 }))
.horizontalTextAlignment(
horizontalTextAlignment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.textWrap(textWrap?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.verticalTextAlignment(
verticalTextAlignment?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.visibility(visibility?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [AnalysisTableCellStyleArgs].
*/
@PulumiTagMarker
public class AnalysisTableCellStyleArgsBuilder internal constructor() {
private var backgroundColor: Output? = null
private var border: Output? = null
private var fontConfiguration: Output? = null
private var height: Output? = null
private var horizontalTextAlignment: Output? = null
private var textWrap: Output? = null
private var verticalTextAlignment: Output? = null
private var visibility: Output? = null
/**
* @param value The background color for the table cells.
*/
@JvmName("hwtnmvpvnjfyvtib")
public suspend fun backgroundColor(`value`: Output) {
this.backgroundColor = value
}
/**
* @param value The borders for the table cells.
*/
@JvmName("cdonwjylsfdlrbgf")
public suspend fun border(`value`: Output) {
this.border = value
}
/**
* @param value The font configuration of the table cells.
*/
@JvmName("bgcdkiisvdkxhlff")
public suspend fun fontConfiguration(`value`: Output) {
this.fontConfiguration = value
}
/**
* @param value The height color for the table cells.
*/
@JvmName("boosqefbnwjvtfrw")
public suspend fun height(`value`: Output) {
this.height = value
}
/**
* @param value The horizontal text alignment (left, center, right, auto) for the table cells.
*/
@JvmName("xlgdswkohicutfwc")
public suspend fun horizontalTextAlignment(`value`: Output) {
this.horizontalTextAlignment = value
}
/**
* @param value The text wrap (none, wrap) for the table cells.
*/
@JvmName("sctlneyilsukbjxc")
public suspend fun textWrap(`value`: Output) {
this.textWrap = value
}
/**
* @param value The vertical text alignment (top, middle, bottom) for the table cells.
*/
@JvmName("tjxxtdnvnrijhoaa")
public suspend fun verticalTextAlignment(`value`: Output) {
this.verticalTextAlignment = value
}
/**
* @param value The visibility of the table cells.
*/
@JvmName("axaqsqjsjfvnjkfl")
public suspend fun visibility(`value`: Output) {
this.visibility = value
}
/**
* @param value The background color for the table cells.
*/
@JvmName("qcfwygkghjeqwccr")
public suspend fun backgroundColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backgroundColor = mapped
}
/**
* @param value The borders for the table cells.
*/
@JvmName("aujmqwqgljplaxkf")
public suspend fun border(`value`: AnalysisGlobalTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.border = mapped
}
/**
* @param argument The borders for the table cells.
*/
@JvmName("rtpjsmoivntsmrws")
public suspend fun border(argument: suspend AnalysisGlobalTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisGlobalTableBorderOptionsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.border = mapped
}
/**
* @param value The font configuration of the table cells.
*/
@JvmName("aytsblffjyvowlev")
public suspend fun fontConfiguration(`value`: AnalysisFontConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fontConfiguration = mapped
}
/**
* @param argument The font configuration of the table cells.
*/
@JvmName("pyjynnmyprkihisg")
public suspend fun fontConfiguration(argument: suspend AnalysisFontConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = AnalysisFontConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fontConfiguration = mapped
}
/**
* @param value The height color for the table cells.
*/
@JvmName("viktilmtlgcdbqej")
public suspend fun height(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.height = mapped
}
/**
* @param value The horizontal text alignment (left, center, right, auto) for the table cells.
*/
@JvmName("nalokfxifesyonkt")
public suspend fun horizontalTextAlignment(`value`: AnalysisHorizontalTextAlignment?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.horizontalTextAlignment = mapped
}
/**
* @param value The text wrap (none, wrap) for the table cells.
*/
@JvmName("ekbriwasyrbgjnrd")
public suspend fun textWrap(`value`: AnalysisTextWrap?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.textWrap = mapped
}
/**
* @param value The vertical text alignment (top, middle, bottom) for the table cells.
*/
@JvmName("eeaarcbdeaoiwquo")
public suspend fun verticalTextAlignment(`value`: AnalysisVerticalTextAlignment?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verticalTextAlignment = mapped
}
/**
* @param value The visibility of the table cells.
*/
@JvmName("hdtjooslwrmpjtpd")
public suspend fun visibility(`value`: AnalysisVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visibility = mapped
}
internal fun build(): AnalysisTableCellStyleArgs = AnalysisTableCellStyleArgs(
backgroundColor = backgroundColor,
border = border,
fontConfiguration = fontConfiguration,
height = height,
horizontalTextAlignment = horizontalTextAlignment,
textWrap = textWrap,
verticalTextAlignment = verticalTextAlignment,
visibility = visibility,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy