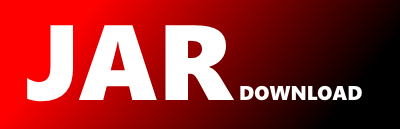
com.pulumi.awsnative.quicksight.kotlin.inputs.DashboardColumnTooltipItemArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.DashboardColumnTooltipItemArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.DashboardTooltipTarget
import com.pulumi.awsnative.quicksight.kotlin.enums.DashboardVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property aggregation The aggregation function of the column tooltip item.
* @property column The target column of the tooltip item.
* @property label The label of the tooltip item.
* @property tooltipTarget
* @property visibility The visibility of the tooltip item.
*/
public data class DashboardColumnTooltipItemArgs(
public val aggregation: Output? = null,
public val column: Output,
public val label: Output? = null,
public val tooltipTarget: Output? = null,
public val visibility: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.DashboardColumnTooltipItemArgs =
com.pulumi.awsnative.quicksight.inputs.DashboardColumnTooltipItemArgs.builder()
.aggregation(aggregation?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.column(column.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.label(label?.applyValue({ args0 -> args0 }))
.tooltipTarget(tooltipTarget?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.visibility(visibility?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DashboardColumnTooltipItemArgs].
*/
@PulumiTagMarker
public class DashboardColumnTooltipItemArgsBuilder internal constructor() {
private var aggregation: Output? = null
private var column: Output? = null
private var label: Output? = null
private var tooltipTarget: Output? = null
private var visibility: Output? = null
/**
* @param value The aggregation function of the column tooltip item.
*/
@JvmName("jwpvlupypealgncp")
public suspend fun aggregation(`value`: Output) {
this.aggregation = value
}
/**
* @param value The target column of the tooltip item.
*/
@JvmName("janbkvipaljdhgak")
public suspend fun column(`value`: Output) {
this.column = value
}
/**
* @param value The label of the tooltip item.
*/
@JvmName("qnlopsqkdsxlhpnu")
public suspend fun label(`value`: Output) {
this.label = value
}
/**
* @param value
*/
@JvmName("jvmyldprseusgstb")
public suspend fun tooltipTarget(`value`: Output) {
this.tooltipTarget = value
}
/**
* @param value The visibility of the tooltip item.
*/
@JvmName("epfevedovwnvfxhr")
public suspend fun visibility(`value`: Output) {
this.visibility = value
}
/**
* @param value The aggregation function of the column tooltip item.
*/
@JvmName("xcsmnghrnfdinwhe")
public suspend fun aggregation(`value`: DashboardAggregationFunctionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.aggregation = mapped
}
/**
* @param argument The aggregation function of the column tooltip item.
*/
@JvmName("wxboeikvccxfujob")
public suspend fun aggregation(argument: suspend DashboardAggregationFunctionArgsBuilder.() -> Unit) {
val toBeMapped = DashboardAggregationFunctionArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.aggregation = mapped
}
/**
* @param value The target column of the tooltip item.
*/
@JvmName("hfvnhqfoqlbvtuix")
public suspend fun column(`value`: DashboardColumnIdentifierArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.column = mapped
}
/**
* @param argument The target column of the tooltip item.
*/
@JvmName("ptqnsvhlncoekbqg")
public suspend fun column(argument: suspend DashboardColumnIdentifierArgsBuilder.() -> Unit) {
val toBeMapped = DashboardColumnIdentifierArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.column = mapped
}
/**
* @param value The label of the tooltip item.
*/
@JvmName("dwjmdsngdygxowuk")
public suspend fun label(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.label = mapped
}
/**
* @param value
*/
@JvmName("rqkdsnfyalkarboa")
public suspend fun tooltipTarget(`value`: DashboardTooltipTarget?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tooltipTarget = mapped
}
/**
* @param value The visibility of the tooltip item.
*/
@JvmName("ujgesjtemetikcby")
public suspend fun visibility(`value`: DashboardVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visibility = mapped
}
internal fun build(): DashboardColumnTooltipItemArgs = DashboardColumnTooltipItemArgs(
aggregation = aggregation,
column = column ?: throw PulumiNullFieldException("column"),
label = label,
tooltipTarget = tooltipTarget,
visibility = visibility,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy