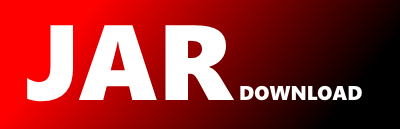
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateKpiOptionsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateKpiOptionsArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplatePrimaryValueDisplayType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property comparison The comparison configuration of a KPI visual.
* @property primaryValueDisplayType The options that determine the primary value display type.
* @property primaryValueFontConfiguration The options that determine the primary value font configuration.
* @property progressBar The options that determine the presentation of the progress bar of a KPI visual.
* @property secondaryValue The options that determine the presentation of the secondary value of a KPI visual.
* @property secondaryValueFontConfiguration The options that determine the secondary value font configuration.
* @property sparkline The options that determine the visibility, color, type, and tooltip visibility of the sparkline of a KPI visual.
* @property trendArrows The options that determine the presentation of trend arrows in a KPI visual.
* @property visualLayoutOptions The options that determine the layout a KPI visual.
*/
public data class TemplateKpiOptionsArgs(
public val comparison: Output? = null,
public val primaryValueDisplayType: Output? = null,
public val primaryValueFontConfiguration: Output? = null,
public val progressBar: Output? = null,
public val secondaryValue: Output? = null,
public val secondaryValueFontConfiguration: Output? = null,
public val sparkline: Output? = null,
public val trendArrows: Output? = null,
public val visualLayoutOptions: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateKpiOptionsArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateKpiOptionsArgs.builder()
.comparison(comparison?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.primaryValueDisplayType(
primaryValueDisplayType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.primaryValueFontConfiguration(
primaryValueFontConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.progressBar(progressBar?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secondaryValue(secondaryValue?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secondaryValueFontConfiguration(
secondaryValueFontConfiguration?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.sparkline(sparkline?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.trendArrows(trendArrows?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.visualLayoutOptions(
visualLayoutOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [TemplateKpiOptionsArgs].
*/
@PulumiTagMarker
public class TemplateKpiOptionsArgsBuilder internal constructor() {
private var comparison: Output? = null
private var primaryValueDisplayType: Output? = null
private var primaryValueFontConfiguration: Output? = null
private var progressBar: Output? = null
private var secondaryValue: Output? = null
private var secondaryValueFontConfiguration: Output? = null
private var sparkline: Output? = null
private var trendArrows: Output? = null
private var visualLayoutOptions: Output? = null
/**
* @param value The comparison configuration of a KPI visual.
*/
@JvmName("fcnmeureehxpnkth")
public suspend fun comparison(`value`: Output) {
this.comparison = value
}
/**
* @param value The options that determine the primary value display type.
*/
@JvmName("rdgycrouklfoouet")
public suspend fun primaryValueDisplayType(`value`: Output) {
this.primaryValueDisplayType = value
}
/**
* @param value The options that determine the primary value font configuration.
*/
@JvmName("vcjmvropdynoupkx")
public suspend fun primaryValueFontConfiguration(`value`: Output) {
this.primaryValueFontConfiguration = value
}
/**
* @param value The options that determine the presentation of the progress bar of a KPI visual.
*/
@JvmName("lxfowyfbtpqegapd")
public suspend fun progressBar(`value`: Output) {
this.progressBar = value
}
/**
* @param value The options that determine the presentation of the secondary value of a KPI visual.
*/
@JvmName("kiwaqwkyourvqrij")
public suspend fun secondaryValue(`value`: Output) {
this.secondaryValue = value
}
/**
* @param value The options that determine the secondary value font configuration.
*/
@JvmName("rqbsjcwmdbyefegg")
public suspend fun secondaryValueFontConfiguration(`value`: Output) {
this.secondaryValueFontConfiguration = value
}
/**
* @param value The options that determine the visibility, color, type, and tooltip visibility of the sparkline of a KPI visual.
*/
@JvmName("rfrjjuuejkqxamhl")
public suspend fun sparkline(`value`: Output) {
this.sparkline = value
}
/**
* @param value The options that determine the presentation of trend arrows in a KPI visual.
*/
@JvmName("lxibvvntwiucwltm")
public suspend fun trendArrows(`value`: Output) {
this.trendArrows = value
}
/**
* @param value The options that determine the layout a KPI visual.
*/
@JvmName("oikautnscheidrym")
public suspend fun visualLayoutOptions(`value`: Output) {
this.visualLayoutOptions = value
}
/**
* @param value The comparison configuration of a KPI visual.
*/
@JvmName("uvcljhveyvtjxrae")
public suspend fun comparison(`value`: TemplateComparisonConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.comparison = mapped
}
/**
* @param argument The comparison configuration of a KPI visual.
*/
@JvmName("gxlsvejketrmonnt")
public suspend fun comparison(argument: suspend TemplateComparisonConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TemplateComparisonConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.comparison = mapped
}
/**
* @param value The options that determine the primary value display type.
*/
@JvmName("ewkccqvgdvyasofg")
public suspend fun primaryValueDisplayType(`value`: TemplatePrimaryValueDisplayType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryValueDisplayType = mapped
}
/**
* @param value The options that determine the primary value font configuration.
*/
@JvmName("xfnagmgnidxhvwig")
public suspend fun primaryValueFontConfiguration(`value`: TemplateFontConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.primaryValueFontConfiguration = mapped
}
/**
* @param argument The options that determine the primary value font configuration.
*/
@JvmName("ihptjpqdbysixgru")
public suspend fun primaryValueFontConfiguration(argument: suspend TemplateFontConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TemplateFontConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.primaryValueFontConfiguration = mapped
}
/**
* @param value The options that determine the presentation of the progress bar of a KPI visual.
*/
@JvmName("fhpeccabcixxjjjm")
public suspend fun progressBar(`value`: TemplateProgressBarOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.progressBar = mapped
}
/**
* @param argument The options that determine the presentation of the progress bar of a KPI visual.
*/
@JvmName("lepdraoqquhgdoht")
public suspend fun progressBar(argument: suspend TemplateProgressBarOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateProgressBarOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.progressBar = mapped
}
/**
* @param value The options that determine the presentation of the secondary value of a KPI visual.
*/
@JvmName("qmnfqfgbnvjydtie")
public suspend fun secondaryValue(`value`: TemplateSecondaryValueOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryValue = mapped
}
/**
* @param argument The options that determine the presentation of the secondary value of a KPI visual.
*/
@JvmName("ruhwvxkfkbiiitcs")
public suspend fun secondaryValue(argument: suspend TemplateSecondaryValueOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateSecondaryValueOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secondaryValue = mapped
}
/**
* @param value The options that determine the secondary value font configuration.
*/
@JvmName("kqqvpgqyxencdrxd")
public suspend fun secondaryValueFontConfiguration(`value`: TemplateFontConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondaryValueFontConfiguration = mapped
}
/**
* @param argument The options that determine the secondary value font configuration.
*/
@JvmName("ydgegisjuiukvdex")
public suspend fun secondaryValueFontConfiguration(argument: suspend TemplateFontConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TemplateFontConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secondaryValueFontConfiguration = mapped
}
/**
* @param value The options that determine the visibility, color, type, and tooltip visibility of the sparkline of a KPI visual.
*/
@JvmName("lphgkjamggolvfas")
public suspend fun sparkline(`value`: TemplateKpiSparklineOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sparkline = mapped
}
/**
* @param argument The options that determine the visibility, color, type, and tooltip visibility of the sparkline of a KPI visual.
*/
@JvmName("tiumoevjyalkgofj")
public suspend fun sparkline(argument: suspend TemplateKpiSparklineOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateKpiSparklineOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sparkline = mapped
}
/**
* @param value The options that determine the presentation of trend arrows in a KPI visual.
*/
@JvmName("xfmggkakjbulxxij")
public suspend fun trendArrows(`value`: TemplateTrendArrowOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trendArrows = mapped
}
/**
* @param argument The options that determine the presentation of trend arrows in a KPI visual.
*/
@JvmName("hxogxtmkfuvlsfvx")
public suspend fun trendArrows(argument: suspend TemplateTrendArrowOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTrendArrowOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.trendArrows = mapped
}
/**
* @param value The options that determine the layout a KPI visual.
*/
@JvmName("iqeppwuiwwtiydhp")
public suspend fun visualLayoutOptions(`value`: TemplateKpiVisualLayoutOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visualLayoutOptions = mapped
}
/**
* @param argument The options that determine the layout a KPI visual.
*/
@JvmName("xrbohjgybuqocmis")
public suspend fun visualLayoutOptions(argument: suspend TemplateKpiVisualLayoutOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateKpiVisualLayoutOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.visualLayoutOptions = mapped
}
internal fun build(): TemplateKpiOptionsArgs = TemplateKpiOptionsArgs(
comparison = comparison,
primaryValueDisplayType = primaryValueDisplayType,
primaryValueFontConfiguration = primaryValueFontConfiguration,
progressBar = progressBar,
secondaryValue = secondaryValue,
secondaryValueFontConfiguration = secondaryValueFontConfiguration,
sparkline = sparkline,
trendArrows = trendArrows,
visualLayoutOptions = visualLayoutOptions,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy