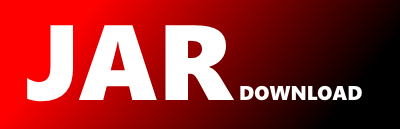
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateParameterControlArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateParameterControlArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property dateTimePicker A control from a date parameter that specifies date and time.
* @property dropdown A control to display a dropdown list with buttons that are used to select a single value.
* @property list A control to display a list with buttons or boxes that are used to select either a single value or multiple values.
* @property slider A control to display a horizontal toggle bar. This is used to change a value by sliding the toggle.
* @property textArea A control to display a text box that is used to enter multiple entries.
* @property textField A control to display a text box that is used to enter a single entry.
*/
public data class TemplateParameterControlArgs(
public val dateTimePicker: Output? = null,
public val dropdown: Output? = null,
public val list: Output? = null,
public val slider: Output? = null,
public val textArea: Output? = null,
public val textField: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateParameterControlArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateParameterControlArgs.builder()
.dateTimePicker(dateTimePicker?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.dropdown(dropdown?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.list(list?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.slider(slider?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.textArea(textArea?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.textField(textField?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TemplateParameterControlArgs].
*/
@PulumiTagMarker
public class TemplateParameterControlArgsBuilder internal constructor() {
private var dateTimePicker: Output? = null
private var dropdown: Output? = null
private var list: Output? = null
private var slider: Output? = null
private var textArea: Output? = null
private var textField: Output? = null
/**
* @param value A control from a date parameter that specifies date and time.
*/
@JvmName("yumpbxtxblwqtnes")
public suspend fun dateTimePicker(`value`: Output) {
this.dateTimePicker = value
}
/**
* @param value A control to display a dropdown list with buttons that are used to select a single value.
*/
@JvmName("qipbxgclbrquxfgo")
public suspend fun dropdown(`value`: Output) {
this.dropdown = value
}
/**
* @param value A control to display a list with buttons or boxes that are used to select either a single value or multiple values.
*/
@JvmName("hcqlopaarqwukgsi")
public suspend fun list(`value`: Output) {
this.list = value
}
/**
* @param value A control to display a horizontal toggle bar. This is used to change a value by sliding the toggle.
*/
@JvmName("lygqcnbwcdcvvqjv")
public suspend fun slider(`value`: Output) {
this.slider = value
}
/**
* @param value A control to display a text box that is used to enter multiple entries.
*/
@JvmName("ecsaaackuxhbshsb")
public suspend fun textArea(`value`: Output) {
this.textArea = value
}
/**
* @param value A control to display a text box that is used to enter a single entry.
*/
@JvmName("bgehajrkvrpjwind")
public suspend fun textField(`value`: Output) {
this.textField = value
}
/**
* @param value A control from a date parameter that specifies date and time.
*/
@JvmName("npnbsjgsilkbfopf")
public suspend fun dateTimePicker(`value`: TemplateParameterDateTimePickerControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dateTimePicker = mapped
}
/**
* @param argument A control from a date parameter that specifies date and time.
*/
@JvmName("rykfvnkqunqqeopw")
public suspend fun dateTimePicker(argument: suspend TemplateParameterDateTimePickerControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterDateTimePickerControlArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dateTimePicker = mapped
}
/**
* @param value A control to display a dropdown list with buttons that are used to select a single value.
*/
@JvmName("lxnwgbsavlmfvgmp")
public suspend fun dropdown(`value`: TemplateParameterDropDownControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dropdown = mapped
}
/**
* @param argument A control to display a dropdown list with buttons that are used to select a single value.
*/
@JvmName("mxyarnwaslqbrien")
public suspend fun dropdown(argument: suspend TemplateParameterDropDownControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterDropDownControlArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dropdown = mapped
}
/**
* @param value A control to display a list with buttons or boxes that are used to select either a single value or multiple values.
*/
@JvmName("ybmrnapsmvtckuva")
public suspend fun list(`value`: TemplateParameterListControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.list = mapped
}
/**
* @param argument A control to display a list with buttons or boxes that are used to select either a single value or multiple values.
*/
@JvmName("icyisofyivfiddoy")
public suspend fun list(argument: suspend TemplateParameterListControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterListControlArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.list = mapped
}
/**
* @param value A control to display a horizontal toggle bar. This is used to change a value by sliding the toggle.
*/
@JvmName("opjtxdyhrtlxrpru")
public suspend fun slider(`value`: TemplateParameterSliderControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.slider = mapped
}
/**
* @param argument A control to display a horizontal toggle bar. This is used to change a value by sliding the toggle.
*/
@JvmName("lqrgjdgkywkvddbq")
public suspend fun slider(argument: suspend TemplateParameterSliderControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterSliderControlArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.slider = mapped
}
/**
* @param value A control to display a text box that is used to enter multiple entries.
*/
@JvmName("psenjrobhncvfmgf")
public suspend fun textArea(`value`: TemplateParameterTextAreaControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.textArea = mapped
}
/**
* @param argument A control to display a text box that is used to enter multiple entries.
*/
@JvmName("voadhpthreglcbjn")
public suspend fun textArea(argument: suspend TemplateParameterTextAreaControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterTextAreaControlArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.textArea = mapped
}
/**
* @param value A control to display a text box that is used to enter a single entry.
*/
@JvmName("ohbjtpuelkqycpxm")
public suspend fun textField(`value`: TemplateParameterTextFieldControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.textField = mapped
}
/**
* @param argument A control to display a text box that is used to enter a single entry.
*/
@JvmName("rntlilxpioewkjfg")
public suspend fun textField(argument: suspend TemplateParameterTextFieldControlArgsBuilder.() -> Unit) {
val toBeMapped = TemplateParameterTextFieldControlArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.textField = mapped
}
internal fun build(): TemplateParameterControlArgs = TemplateParameterControlArgs(
dateTimePicker = dateTimePicker,
dropdown = dropdown,
list = list,
slider = slider,
textArea = textArea,
textField = textField,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy