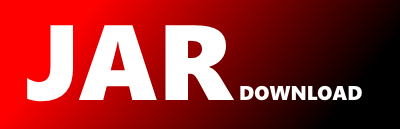
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateRadarChartConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateRadarChartConfigurationArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateRadarChartAxesRangeScale
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateRadarChartShape
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property alternateBandColorsVisibility Determines the visibility of the colors of alternatign bands in a radar chart.
* @property alternateBandEvenColor The color of the even-numbered alternate bands of a radar chart.
* @property alternateBandOddColor The color of the odd-numbered alternate bands of a radar chart.
* @property axesRangeScale The axis behavior options of a radar chart.
* @property baseSeriesSettings The base sreies settings of a radar chart.
* @property categoryAxis The category axis of a radar chart.
* @property categoryLabelOptions The category label options of a radar chart.
* @property colorAxis The color axis of a radar chart.
* @property colorLabelOptions The color label options of a radar chart.
* @property fieldWells The field well configuration of a `RadarChartVisual` .
* @property legend The legend display setup of the visual.
* @property shape The shape of the radar chart.
* @property sortConfiguration The sort configuration of a `RadarChartVisual` .
* @property startAngle The start angle of a radar chart's axis.
* @property visualPalette The palette (chart color) display setup of the visual.
*/
public data class TemplateRadarChartConfigurationArgs(
public val alternateBandColorsVisibility: Output? = null,
public val alternateBandEvenColor: Output? = null,
public val alternateBandOddColor: Output? = null,
public val axesRangeScale: Output? = null,
public val baseSeriesSettings: Output? = null,
public val categoryAxis: Output? = null,
public val categoryLabelOptions: Output? = null,
public val colorAxis: Output? = null,
public val colorLabelOptions: Output? = null,
public val fieldWells: Output? = null,
public val legend: Output? = null,
public val shape: Output? = null,
public val sortConfiguration: Output? = null,
public val startAngle: Output? = null,
public val visualPalette: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateRadarChartConfigurationArgs = com.pulumi.awsnative.quicksight.inputs.TemplateRadarChartConfigurationArgs.builder()
.alternateBandColorsVisibility(
alternateBandColorsVisibility?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.alternateBandEvenColor(alternateBandEvenColor?.applyValue({ args0 -> args0 }))
.alternateBandOddColor(alternateBandOddColor?.applyValue({ args0 -> args0 }))
.axesRangeScale(axesRangeScale?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.baseSeriesSettings(
baseSeriesSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.categoryAxis(categoryAxis?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.categoryLabelOptions(
categoryLabelOptions?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.colorAxis(colorAxis?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.colorLabelOptions(colorLabelOptions?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.fieldWells(fieldWells?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.legend(legend?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.shape(shape?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sortConfiguration(sortConfiguration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.startAngle(startAngle?.applyValue({ args0 -> args0 }))
.visualPalette(visualPalette?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TemplateRadarChartConfigurationArgs].
*/
@PulumiTagMarker
public class TemplateRadarChartConfigurationArgsBuilder internal constructor() {
private var alternateBandColorsVisibility: Output? = null
private var alternateBandEvenColor: Output? = null
private var alternateBandOddColor: Output? = null
private var axesRangeScale: Output? = null
private var baseSeriesSettings: Output? = null
private var categoryAxis: Output? = null
private var categoryLabelOptions: Output? = null
private var colorAxis: Output? = null
private var colorLabelOptions: Output? = null
private var fieldWells: Output? = null
private var legend: Output? = null
private var shape: Output? = null
private var sortConfiguration: Output? = null
private var startAngle: Output? = null
private var visualPalette: Output? = null
/**
* @param value Determines the visibility of the colors of alternatign bands in a radar chart.
*/
@JvmName("hajqoonxsovhgidc")
public suspend fun alternateBandColorsVisibility(`value`: Output) {
this.alternateBandColorsVisibility = value
}
/**
* @param value The color of the even-numbered alternate bands of a radar chart.
*/
@JvmName("ifqsrqanfbctpsey")
public suspend fun alternateBandEvenColor(`value`: Output) {
this.alternateBandEvenColor = value
}
/**
* @param value The color of the odd-numbered alternate bands of a radar chart.
*/
@JvmName("vjocgsvdopfavsxg")
public suspend fun alternateBandOddColor(`value`: Output) {
this.alternateBandOddColor = value
}
/**
* @param value The axis behavior options of a radar chart.
*/
@JvmName("ajixnxxbausfuaqb")
public suspend fun axesRangeScale(`value`: Output) {
this.axesRangeScale = value
}
/**
* @param value The base sreies settings of a radar chart.
*/
@JvmName("gelxdfjrbeqebhcp")
public suspend fun baseSeriesSettings(`value`: Output) {
this.baseSeriesSettings = value
}
/**
* @param value The category axis of a radar chart.
*/
@JvmName("xcloxpbjdqhudmlf")
public suspend fun categoryAxis(`value`: Output) {
this.categoryAxis = value
}
/**
* @param value The category label options of a radar chart.
*/
@JvmName("jsaqagotdjhhialy")
public suspend fun categoryLabelOptions(`value`: Output) {
this.categoryLabelOptions = value
}
/**
* @param value The color axis of a radar chart.
*/
@JvmName("dhfkoblruvecikem")
public suspend fun colorAxis(`value`: Output) {
this.colorAxis = value
}
/**
* @param value The color label options of a radar chart.
*/
@JvmName("haaqodgyqcsdogmp")
public suspend fun colorLabelOptions(`value`: Output) {
this.colorLabelOptions = value
}
/**
* @param value The field well configuration of a `RadarChartVisual` .
*/
@JvmName("qgiiqhvtactpajgj")
public suspend fun fieldWells(`value`: Output) {
this.fieldWells = value
}
/**
* @param value The legend display setup of the visual.
*/
@JvmName("roecbrlnuvpmefdr")
public suspend fun legend(`value`: Output) {
this.legend = value
}
/**
* @param value The shape of the radar chart.
*/
@JvmName("kesoklfsvkysdhpf")
public suspend fun shape(`value`: Output) {
this.shape = value
}
/**
* @param value The sort configuration of a `RadarChartVisual` .
*/
@JvmName("jweftcboqpavpuxf")
public suspend fun sortConfiguration(`value`: Output) {
this.sortConfiguration = value
}
/**
* @param value The start angle of a radar chart's axis.
*/
@JvmName("fdgpblolawewrire")
public suspend fun startAngle(`value`: Output) {
this.startAngle = value
}
/**
* @param value The palette (chart color) display setup of the visual.
*/
@JvmName("wejjyokjbtcysqtu")
public suspend fun visualPalette(`value`: Output) {
this.visualPalette = value
}
/**
* @param value Determines the visibility of the colors of alternatign bands in a radar chart.
*/
@JvmName("wlqdmwlhiigxrndo")
public suspend fun alternateBandColorsVisibility(`value`: TemplateVisibility?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alternateBandColorsVisibility = mapped
}
/**
* @param value The color of the even-numbered alternate bands of a radar chart.
*/
@JvmName("dmhnatwioefktpmb")
public suspend fun alternateBandEvenColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alternateBandEvenColor = mapped
}
/**
* @param value The color of the odd-numbered alternate bands of a radar chart.
*/
@JvmName("jvwjomgaewghvpyb")
public suspend fun alternateBandOddColor(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alternateBandOddColor = mapped
}
/**
* @param value The axis behavior options of a radar chart.
*/
@JvmName("nqgwmeukibusthdh")
public suspend fun axesRangeScale(`value`: TemplateRadarChartAxesRangeScale?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.axesRangeScale = mapped
}
/**
* @param value The base sreies settings of a radar chart.
*/
@JvmName("hfehtscdvsjahtne")
public suspend fun baseSeriesSettings(`value`: TemplateRadarChartSeriesSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseSeriesSettings = mapped
}
/**
* @param argument The base sreies settings of a radar chart.
*/
@JvmName("semwfaojnlbexxyf")
public suspend fun baseSeriesSettings(argument: suspend TemplateRadarChartSeriesSettingsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateRadarChartSeriesSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.baseSeriesSettings = mapped
}
/**
* @param value The category axis of a radar chart.
*/
@JvmName("xwcynosyrltyslyo")
public suspend fun categoryAxis(`value`: TemplateAxisDisplayOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.categoryAxis = mapped
}
/**
* @param argument The category axis of a radar chart.
*/
@JvmName("euinreamiugfxlmd")
public suspend fun categoryAxis(argument: suspend TemplateAxisDisplayOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateAxisDisplayOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.categoryAxis = mapped
}
/**
* @param value The category label options of a radar chart.
*/
@JvmName("fmiriybhgheuetcw")
public suspend fun categoryLabelOptions(`value`: TemplateChartAxisLabelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.categoryLabelOptions = mapped
}
/**
* @param argument The category label options of a radar chart.
*/
@JvmName("vnvdkjfdrshbsoad")
public suspend fun categoryLabelOptions(argument: suspend TemplateChartAxisLabelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateChartAxisLabelOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.categoryLabelOptions = mapped
}
/**
* @param value The color axis of a radar chart.
*/
@JvmName("idtibnxxvdjpmjnc")
public suspend fun colorAxis(`value`: TemplateAxisDisplayOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.colorAxis = mapped
}
/**
* @param argument The color axis of a radar chart.
*/
@JvmName("bvtmacjfxctgsltx")
public suspend fun colorAxis(argument: suspend TemplateAxisDisplayOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateAxisDisplayOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.colorAxis = mapped
}
/**
* @param value The color label options of a radar chart.
*/
@JvmName("giajxbqwjuoojrtk")
public suspend fun colorLabelOptions(`value`: TemplateChartAxisLabelOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.colorLabelOptions = mapped
}
/**
* @param argument The color label options of a radar chart.
*/
@JvmName("xibqowhbactldesw")
public suspend fun colorLabelOptions(argument: suspend TemplateChartAxisLabelOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateChartAxisLabelOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.colorLabelOptions = mapped
}
/**
* @param value The field well configuration of a `RadarChartVisual` .
*/
@JvmName("bgoicyfaqdexaflc")
public suspend fun fieldWells(`value`: TemplateRadarChartFieldWellsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldWells = mapped
}
/**
* @param argument The field well configuration of a `RadarChartVisual` .
*/
@JvmName("pampmvvuiuqtgiuf")
public suspend fun fieldWells(argument: suspend TemplateRadarChartFieldWellsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateRadarChartFieldWellsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.fieldWells = mapped
}
/**
* @param value The legend display setup of the visual.
*/
@JvmName("qwkdekebtxqrxmln")
public suspend fun legend(`value`: TemplateLegendOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.legend = mapped
}
/**
* @param argument The legend display setup of the visual.
*/
@JvmName("dwbykhdstodnumdi")
public suspend fun legend(argument: suspend TemplateLegendOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateLegendOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.legend = mapped
}
/**
* @param value The shape of the radar chart.
*/
@JvmName("qytaywtoxkcqhftb")
public suspend fun shape(`value`: TemplateRadarChartShape?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shape = mapped
}
/**
* @param value The sort configuration of a `RadarChartVisual` .
*/
@JvmName("iqgjhrwovnwupvjj")
public suspend fun sortConfiguration(`value`: TemplateRadarChartSortConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sortConfiguration = mapped
}
/**
* @param argument The sort configuration of a `RadarChartVisual` .
*/
@JvmName("qsvckirjfitljflj")
public suspend fun sortConfiguration(argument: suspend TemplateRadarChartSortConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = TemplateRadarChartSortConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sortConfiguration = mapped
}
/**
* @param value The start angle of a radar chart's axis.
*/
@JvmName("rfsmjdvqupxcociy")
public suspend fun startAngle(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startAngle = mapped
}
/**
* @param value The palette (chart color) display setup of the visual.
*/
@JvmName("trmyldrwkewkirxj")
public suspend fun visualPalette(`value`: TemplateVisualPaletteArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visualPalette = mapped
}
/**
* @param argument The palette (chart color) display setup of the visual.
*/
@JvmName("vtgycexeygtvwtve")
public suspend fun visualPalette(argument: suspend TemplateVisualPaletteArgsBuilder.() -> Unit) {
val toBeMapped = TemplateVisualPaletteArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.visualPalette = mapped
}
internal fun build(): TemplateRadarChartConfigurationArgs = TemplateRadarChartConfigurationArgs(
alternateBandColorsVisibility = alternateBandColorsVisibility,
alternateBandEvenColor = alternateBandEvenColor,
alternateBandOddColor = alternateBandOddColor,
axesRangeScale = axesRangeScale,
baseSeriesSettings = baseSeriesSettings,
categoryAxis = categoryAxis,
categoryLabelOptions = categoryLabelOptions,
colorAxis = colorAxis,
colorLabelOptions = colorLabelOptions,
fieldWells = fieldWells,
legend = legend,
shape = shape,
sortConfiguration = sortConfiguration,
startAngle = startAngle,
visualPalette = visualPalette,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy