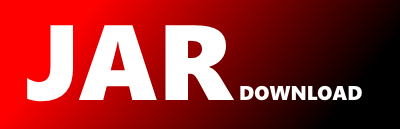
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateTableSideBorderOptionsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateTableSideBorderOptionsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property bottom The table border options of the bottom border.
* @property innerHorizontal The table border options of the inner horizontal border.
* @property innerVertical The table border options of the inner vertical border.
* @property left The table border options of the left border.
* @property right The table border options of the right border.
* @property top The table border options of the top border.
*/
public data class TemplateTableSideBorderOptionsArgs(
public val bottom: Output? = null,
public val innerHorizontal: Output? = null,
public val innerVertical: Output? = null,
public val left: Output? = null,
public val right: Output? = null,
public val top: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateTableSideBorderOptionsArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateTableSideBorderOptionsArgs.builder()
.bottom(bottom?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.innerHorizontal(innerHorizontal?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.innerVertical(innerVertical?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.left(left?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.right(right?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.top(top?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TemplateTableSideBorderOptionsArgs].
*/
@PulumiTagMarker
public class TemplateTableSideBorderOptionsArgsBuilder internal constructor() {
private var bottom: Output? = null
private var innerHorizontal: Output? = null
private var innerVertical: Output? = null
private var left: Output? = null
private var right: Output? = null
private var top: Output? = null
/**
* @param value The table border options of the bottom border.
*/
@JvmName("gebjganacftnalge")
public suspend fun bottom(`value`: Output) {
this.bottom = value
}
/**
* @param value The table border options of the inner horizontal border.
*/
@JvmName("mrmpkbwuuxjpkuay")
public suspend fun innerHorizontal(`value`: Output) {
this.innerHorizontal = value
}
/**
* @param value The table border options of the inner vertical border.
*/
@JvmName("ifwukvoykxruceyw")
public suspend fun innerVertical(`value`: Output) {
this.innerVertical = value
}
/**
* @param value The table border options of the left border.
*/
@JvmName("srchuwddaoxnsyqb")
public suspend fun left(`value`: Output) {
this.left = value
}
/**
* @param value The table border options of the right border.
*/
@JvmName("ilvcfqryrjnkxrcw")
public suspend fun right(`value`: Output) {
this.right = value
}
/**
* @param value The table border options of the top border.
*/
@JvmName("gstiruvxfxdsrwgc")
public suspend fun top(`value`: Output) {
this.top = value
}
/**
* @param value The table border options of the bottom border.
*/
@JvmName("fsnmvhiijynkmlvj")
public suspend fun bottom(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bottom = mapped
}
/**
* @param argument The table border options of the bottom border.
*/
@JvmName("gcedherekncpupvo")
public suspend fun bottom(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.bottom = mapped
}
/**
* @param value The table border options of the inner horizontal border.
*/
@JvmName("rdnlkbykwaldqtis")
public suspend fun innerHorizontal(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.innerHorizontal = mapped
}
/**
* @param argument The table border options of the inner horizontal border.
*/
@JvmName("sxfwvuqlmwokaywh")
public suspend fun innerHorizontal(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.innerHorizontal = mapped
}
/**
* @param value The table border options of the inner vertical border.
*/
@JvmName("lydbotopkxekfhpc")
public suspend fun innerVertical(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.innerVertical = mapped
}
/**
* @param argument The table border options of the inner vertical border.
*/
@JvmName("vgykeoxdwwnwonpp")
public suspend fun innerVertical(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.innerVertical = mapped
}
/**
* @param value The table border options of the left border.
*/
@JvmName("cobtflyckrvjbdwt")
public suspend fun left(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.left = mapped
}
/**
* @param argument The table border options of the left border.
*/
@JvmName("ueoedhacwlyqaath")
public suspend fun left(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.left = mapped
}
/**
* @param value The table border options of the right border.
*/
@JvmName("utlmlduejldppwsr")
public suspend fun right(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.right = mapped
}
/**
* @param argument The table border options of the right border.
*/
@JvmName("enobsxvwfyhdvlvd")
public suspend fun right(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.right = mapped
}
/**
* @param value The table border options of the top border.
*/
@JvmName("ontrlncetogxusia")
public suspend fun top(`value`: TemplateTableBorderOptionsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.top = mapped
}
/**
* @param argument The table border options of the top border.
*/
@JvmName("nibggmlcgpfcaebv")
public suspend fun top(argument: suspend TemplateTableBorderOptionsArgsBuilder.() -> Unit) {
val toBeMapped = TemplateTableBorderOptionsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.top = mapped
}
internal fun build(): TemplateTableSideBorderOptionsArgs = TemplateTableSideBorderOptionsArgs(
bottom = bottom,
innerHorizontal = innerHorizontal,
innerVertical = innerVertical,
left = left,
right = right,
top = top,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy