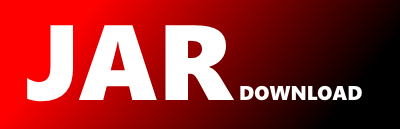
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateTopBottomRankedComputationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateTopBottomRankedComputationArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateTopBottomComputationType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property category The category field that is used in a computation.
* @property computationId The ID for a computation.
* @property name The name of a computation.
* @property resultSize The result size of a top and bottom ranked computation.
* @property type The computation type. Choose one of the following options:
* - TOP: A top ranked computation.
* - BOTTOM: A bottom ranked computation.
* @property value The value field that is used in a computation.
*/
public data class TemplateTopBottomRankedComputationArgs(
public val category: Output? = null,
public val computationId: Output,
public val name: Output? = null,
public val resultSize: Output? = null,
public val type: Output,
public val `value`: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateTopBottomRankedComputationArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateTopBottomRankedComputationArgs.builder()
.category(category?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.computationId(computationId.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.resultSize(resultSize?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.`value`(`value`?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [TemplateTopBottomRankedComputationArgs].
*/
@PulumiTagMarker
public class TemplateTopBottomRankedComputationArgsBuilder internal constructor() {
private var category: Output? = null
private var computationId: Output? = null
private var name: Output? = null
private var resultSize: Output? = null
private var type: Output? = null
private var `value`: Output? = null
/**
* @param value The category field that is used in a computation.
*/
@JvmName("vunolhwiwxgpvpqj")
public suspend fun category(`value`: Output) {
this.category = value
}
/**
* @param value The ID for a computation.
*/
@JvmName("hgaymxyjxjnisyvp")
public suspend fun computationId(`value`: Output) {
this.computationId = value
}
/**
* @param value The name of a computation.
*/
@JvmName("qlgyqktipqlepirh")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The result size of a top and bottom ranked computation.
*/
@JvmName("wskgeifyjogjrltc")
public suspend fun resultSize(`value`: Output) {
this.resultSize = value
}
/**
* @param value The computation type. Choose one of the following options:
* - TOP: A top ranked computation.
* - BOTTOM: A bottom ranked computation.
*/
@JvmName("akeeujtjflctgigg")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The value field that is used in a computation.
*/
@JvmName("viacgmyndyjgpatm")
public suspend fun `value`(`value`: Output) {
this.`value` = value
}
/**
* @param value The category field that is used in a computation.
*/
@JvmName("birgjvexemlufaou")
public suspend fun category(`value`: TemplateDimensionFieldArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.category = mapped
}
/**
* @param argument The category field that is used in a computation.
*/
@JvmName("hdypdexgfpjyolcc")
public suspend fun category(argument: suspend TemplateDimensionFieldArgsBuilder.() -> Unit) {
val toBeMapped = TemplateDimensionFieldArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.category = mapped
}
/**
* @param value The ID for a computation.
*/
@JvmName("xwhacjbyetjnekir")
public suspend fun computationId(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.computationId = mapped
}
/**
* @param value The name of a computation.
*/
@JvmName("fmyspyphtrelrmtn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The result size of a top and bottom ranked computation.
*/
@JvmName("haixcpawtadtpbex")
public suspend fun resultSize(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resultSize = mapped
}
/**
* @param value The computation type. Choose one of the following options:
* - TOP: A top ranked computation.
* - BOTTOM: A bottom ranked computation.
*/
@JvmName("oewqfejlrabldlan")
public suspend fun type(`value`: TemplateTopBottomComputationType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value The value field that is used in a computation.
*/
@JvmName("epehpyuokejvpkig")
public suspend fun `value`(`value`: TemplateMeasureFieldArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`value` = mapped
}
/**
* @param argument The value field that is used in a computation.
*/
@JvmName("chltpflbqmfpsdck")
public suspend fun `value`(argument: suspend TemplateMeasureFieldArgsBuilder.() -> Unit) {
val toBeMapped = TemplateMeasureFieldArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.`value` = mapped
}
internal fun build(): TemplateTopBottomRankedComputationArgs =
TemplateTopBottomRankedComputationArgs(
category = category,
computationId = computationId ?: throw PulumiNullFieldException("computationId"),
name = name,
resultSize = resultSize,
type = type ?: throw PulumiNullFieldException("type"),
`value` = `value`,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy