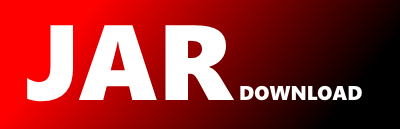
com.pulumi.awsnative.quicksight.kotlin.inputs.TemplateTotalOptionsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.quicksight.kotlin.inputs
import com.pulumi.awsnative.quicksight.inputs.TemplateTotalOptionsArgs.builder
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateTableTotalsPlacement
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateTableTotalsScrollStatus
import com.pulumi.awsnative.quicksight.kotlin.enums.TemplateVisibility
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property customLabel The custom label string for the total cells.
* @property placement The placement (start, end) for the total cells.
* @property scrollStatus The scroll status (pinned, scrolled) for the total cells.
* @property totalAggregationOptions The total aggregation settings for each value field.
* @property totalCellStyle Cell styling options for the total cells.
* @property totalsVisibility The visibility configuration for the total cells.
*/
public data class TemplateTotalOptionsArgs(
public val customLabel: Output? = null,
public val placement: Output? = null,
public val scrollStatus: Output? = null,
public val totalAggregationOptions: Output>? = null,
public val totalCellStyle: Output? = null,
public val totalsVisibility: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.quicksight.inputs.TemplateTotalOptionsArgs =
com.pulumi.awsnative.quicksight.inputs.TemplateTotalOptionsArgs.builder()
.customLabel(customLabel?.applyValue({ args0 -> args0 }))
.placement(placement?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scrollStatus(scrollStatus?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.totalAggregationOptions(
totalAggregationOptions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> args0.toJava() })
})
}),
)
.totalCellStyle(totalCellStyle?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.totalsVisibility(
totalsVisibility?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [TemplateTotalOptionsArgs].
*/
@PulumiTagMarker
public class TemplateTotalOptionsArgsBuilder internal constructor() {
private var customLabel: Output? = null
private var placement: Output? = null
private var scrollStatus: Output? = null
private var totalAggregationOptions: Output>? = null
private var totalCellStyle: Output? = null
private var totalsVisibility: Output? = null
/**
* @param value The custom label string for the total cells.
*/
@JvmName("hoaflnhmuvibmhew")
public suspend fun customLabel(`value`: Output) {
this.customLabel = value
}
/**
* @param value The placement (start, end) for the total cells.
*/
@JvmName("cikcebyrvlfomqrk")
public suspend fun placement(`value`: Output) {
this.placement = value
}
/**
* @param value The scroll status (pinned, scrolled) for the total cells.
*/
@JvmName("vqaytiawmalybypn")
public suspend fun scrollStatus(`value`: Output) {
this.scrollStatus = value
}
/**
* @param value The total aggregation settings for each value field.
*/
@JvmName("hbklvgaodgubwujp")
public suspend fun totalAggregationOptions(`value`: Output>) {
this.totalAggregationOptions = value
}
@JvmName("pxyuvoqnbiythfxx")
public suspend fun totalAggregationOptions(vararg values: Output) {
this.totalAggregationOptions = Output.all(values.asList())
}
/**
* @param values The total aggregation settings for each value field.
*/
@JvmName("lhemvqlfdyhlvpuj")
public suspend fun totalAggregationOptions(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy