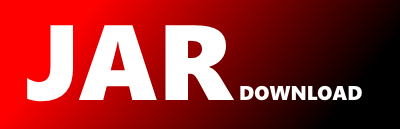
com.pulumi.awsnative.rds.kotlin.DbProxyTargetGroupArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.rds.kotlin
import com.pulumi.awsnative.rds.DbProxyTargetGroupArgs.builder
import com.pulumi.awsnative.rds.kotlin.enums.DbProxyTargetGroupTargetGroupName
import com.pulumi.awsnative.rds.kotlin.inputs.DbProxyTargetGroupConnectionPoolConfigurationInfoFormatArgs
import com.pulumi.awsnative.rds.kotlin.inputs.DbProxyTargetGroupConnectionPoolConfigurationInfoFormatArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS::RDS::DBProxyTargetGroup
* @property connectionPoolConfigurationInfo Displays the settings that control the size and behavior of the connection pool associated with a `DBProxyTarget` .
* @property dbClusterIdentifiers One or more DB cluster identifiers.
* @property dbInstanceIdentifiers One or more DB instance identifiers.
* @property dbProxyName The identifier for the proxy.
* @property targetGroupName The identifier for the DBProxyTargetGroup
*/
public data class DbProxyTargetGroupArgs(
public val connectionPoolConfigurationInfo: Output? = null,
public val dbClusterIdentifiers: Output>? = null,
public val dbInstanceIdentifiers: Output>? = null,
public val dbProxyName: Output? = null,
public val targetGroupName: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.rds.DbProxyTargetGroupArgs =
com.pulumi.awsnative.rds.DbProxyTargetGroupArgs.builder()
.connectionPoolConfigurationInfo(
connectionPoolConfigurationInfo?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.dbClusterIdentifiers(dbClusterIdentifiers?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.dbInstanceIdentifiers(dbInstanceIdentifiers?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.dbProxyName(dbProxyName?.applyValue({ args0 -> args0 }))
.targetGroupName(
targetGroupName?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [DbProxyTargetGroupArgs].
*/
@PulumiTagMarker
public class DbProxyTargetGroupArgsBuilder internal constructor() {
private var connectionPoolConfigurationInfo:
Output? = null
private var dbClusterIdentifiers: Output>? = null
private var dbInstanceIdentifiers: Output>? = null
private var dbProxyName: Output? = null
private var targetGroupName: Output? = null
/**
* @param value Displays the settings that control the size and behavior of the connection pool associated with a `DBProxyTarget` .
*/
@JvmName("dplujtvmivixqpmy")
public suspend fun connectionPoolConfigurationInfo(`value`: Output) {
this.connectionPoolConfigurationInfo = value
}
/**
* @param value One or more DB cluster identifiers.
*/
@JvmName("fpatcvifnolecudn")
public suspend fun dbClusterIdentifiers(`value`: Output>) {
this.dbClusterIdentifiers = value
}
@JvmName("npxndplflqbpjlei")
public suspend fun dbClusterIdentifiers(vararg values: Output) {
this.dbClusterIdentifiers = Output.all(values.asList())
}
/**
* @param values One or more DB cluster identifiers.
*/
@JvmName("saqngnbhatsgsaqe")
public suspend fun dbClusterIdentifiers(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy