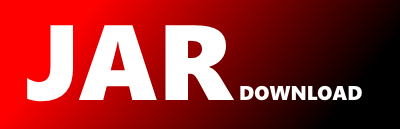
com.pulumi.awsnative.rds.kotlin.GlobalClusterArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.rds.kotlin
import com.pulumi.awsnative.rds.GlobalClusterArgs.builder
import com.pulumi.awsnative.rds.kotlin.enums.GlobalClusterEngine
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::RDS::GlobalCluster
* @property deletionProtection The deletion protection setting for the new global database. The global database can't be deleted when deletion protection is enabled.
* @property engine The name of the database engine to be used for this DB cluster. Valid Values: aurora (for MySQL 5.6-compatible Aurora), aurora-mysql (for MySQL 5.7-compatible Aurora).
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
* @property engineLifecycleSupport The life cycle type of the global cluster. You can use this setting to enroll your global cluster into Amazon RDS Extended Support.
* @property engineVersion The version number of the database engine to use. If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
* @property globalClusterIdentifier The cluster identifier of the new global database cluster. This parameter is stored as a lowercase string.
* @property sourceDbClusterIdentifier The Amazon Resource Name (ARN) to use as the primary cluster of the global database. This parameter is optional. This parameter is stored as a lowercase string.
* @property storageEncrypted The storage encryption setting for the new global database cluster.
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
public data class GlobalClusterArgs(
public val deletionProtection: Output? = null,
public val engine: Output? = null,
public val engineLifecycleSupport: Output? = null,
public val engineVersion: Output? = null,
public val globalClusterIdentifier: Output? = null,
public val sourceDbClusterIdentifier: Output? = null,
public val storageEncrypted: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.rds.GlobalClusterArgs =
com.pulumi.awsnative.rds.GlobalClusterArgs.builder()
.deletionProtection(deletionProtection?.applyValue({ args0 -> args0 }))
.engine(engine?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.engineLifecycleSupport(engineLifecycleSupport?.applyValue({ args0 -> args0 }))
.engineVersion(engineVersion?.applyValue({ args0 -> args0 }))
.globalClusterIdentifier(globalClusterIdentifier?.applyValue({ args0 -> args0 }))
.sourceDbClusterIdentifier(sourceDbClusterIdentifier?.applyValue({ args0 -> args0 }))
.storageEncrypted(storageEncrypted?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [GlobalClusterArgs].
*/
@PulumiTagMarker
public class GlobalClusterArgsBuilder internal constructor() {
private var deletionProtection: Output? = null
private var engine: Output? = null
private var engineLifecycleSupport: Output? = null
private var engineVersion: Output? = null
private var globalClusterIdentifier: Output? = null
private var sourceDbClusterIdentifier: Output? = null
private var storageEncrypted: Output? = null
/**
* @param value The deletion protection setting for the new global database. The global database can't be deleted when deletion protection is enabled.
*/
@JvmName("gcrylsossaqxxtay")
public suspend fun deletionProtection(`value`: Output) {
this.deletionProtection = value
}
/**
* @param value The name of the database engine to be used for this DB cluster. Valid Values: aurora (for MySQL 5.6-compatible Aurora), aurora-mysql (for MySQL 5.7-compatible Aurora).
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("sjimxrooxhijrtea")
public suspend fun engine(`value`: Output) {
this.engine = value
}
/**
* @param value The life cycle type of the global cluster. You can use this setting to enroll your global cluster into Amazon RDS Extended Support.
*/
@JvmName("tfnxpedqvipfrttw")
public suspend fun engineLifecycleSupport(`value`: Output) {
this.engineLifecycleSupport = value
}
/**
* @param value The version number of the database engine to use. If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("gjygulicniwqidsm")
public suspend fun engineVersion(`value`: Output) {
this.engineVersion = value
}
/**
* @param value The cluster identifier of the new global database cluster. This parameter is stored as a lowercase string.
*/
@JvmName("qbpsyjfjouvylmyr")
public suspend fun globalClusterIdentifier(`value`: Output) {
this.globalClusterIdentifier = value
}
/**
* @param value The Amazon Resource Name (ARN) to use as the primary cluster of the global database. This parameter is optional. This parameter is stored as a lowercase string.
*/
@JvmName("vurvpxkymqdcoptq")
public suspend fun sourceDbClusterIdentifier(`value`: Output) {
this.sourceDbClusterIdentifier = value
}
/**
* @param value The storage encryption setting for the new global database cluster.
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("gnvpstbrfgomkwgg")
public suspend fun storageEncrypted(`value`: Output) {
this.storageEncrypted = value
}
/**
* @param value The deletion protection setting for the new global database. The global database can't be deleted when deletion protection is enabled.
*/
@JvmName("gekpawmvikrwqmsd")
public suspend fun deletionProtection(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deletionProtection = mapped
}
/**
* @param value The name of the database engine to be used for this DB cluster. Valid Values: aurora (for MySQL 5.6-compatible Aurora), aurora-mysql (for MySQL 5.7-compatible Aurora).
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("sohjvvyrdvhkxcml")
public suspend fun engine(`value`: GlobalClusterEngine?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.engine = mapped
}
/**
* @param value The life cycle type of the global cluster. You can use this setting to enroll your global cluster into Amazon RDS Extended Support.
*/
@JvmName("fjhjxhvoswtjnqbh")
public suspend fun engineLifecycleSupport(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.engineLifecycleSupport = mapped
}
/**
* @param value The version number of the database engine to use. If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("qraqctpdurewmssf")
public suspend fun engineVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.engineVersion = mapped
}
/**
* @param value The cluster identifier of the new global database cluster. This parameter is stored as a lowercase string.
*/
@JvmName("xbafalyjykykcqqy")
public suspend fun globalClusterIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.globalClusterIdentifier = mapped
}
/**
* @param value The Amazon Resource Name (ARN) to use as the primary cluster of the global database. This parameter is optional. This parameter is stored as a lowercase string.
*/
@JvmName("iuaipeiqrukeeqig")
public suspend fun sourceDbClusterIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceDbClusterIdentifier = mapped
}
/**
* @param value The storage encryption setting for the new global database cluster.
* If you specify the SourceDBClusterIdentifier property, don't specify this property. The value is inherited from the cluster.
*/
@JvmName("udtkrovgytxgnvmp")
public suspend fun storageEncrypted(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageEncrypted = mapped
}
internal fun build(): GlobalClusterArgs = GlobalClusterArgs(
deletionProtection = deletionProtection,
engine = engine,
engineLifecycleSupport = engineLifecycleSupport,
engineVersion = engineVersion,
globalClusterIdentifier = globalClusterIdentifier,
sourceDbClusterIdentifier = sourceDbClusterIdentifier,
storageEncrypted = storageEncrypted,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy