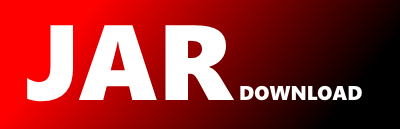
com.pulumi.awsnative.rds.kotlin.inputs.DbClusterScalingConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.rds.kotlin.inputs
import com.pulumi.awsnative.rds.inputs.DbClusterScalingConfigurationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The ``ScalingConfiguration`` property type specifies the scaling configuration of an Aurora Serverless v1 DB cluster.
* For more information, see [Using Amazon Aurora Serverless](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless.html) in the *Amazon Aurora User Guide*.
* This property is only supported for Aurora Serverless v1. For Aurora Serverless v2, Use the ``ServerlessV2ScalingConfiguration`` property.
* Valid for: Aurora Serverless v1 DB clusters only
* @property autoPause Indicates whether to allow or disallow automatic pause for an Aurora DB cluster in ``serverless`` DB engine mode. A DB cluster can be paused only when it's idle (it has no connections).
* If a DB cluster is paused for more than seven days, the DB cluster might be backed up with a snapshot. In this case, the DB cluster is restored when there is a request to connect to it.
* @property maxCapacity The maximum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The maximum capacity must be greater than or equal to the minimum capacity.
* @property minCapacity The minimum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The minimum capacity must be less than or equal to the maximum capacity.
* @property secondsBeforeTimeout The amount of time, in seconds, that Aurora Serverless v1 tries to find a scaling point to perform seamless scaling before enforcing the timeout action. The default is 300.
* Specify a value between 60 and 600 seconds.
* @property secondsUntilAutoPause The time, in seconds, before an Aurora DB cluster in ``serverless`` mode is paused.
* Specify a value between 300 and 86,400 seconds.
* @property timeoutAction The action to take when the timeout is reached, either ``ForceApplyCapacityChange`` or ``RollbackCapacityChange``.
* ``ForceApplyCapacityChange`` sets the capacity to the specified value as soon as possible.
* ``RollbackCapacityChange``, the default, ignores the capacity change if a scaling point isn't found in the timeout period.
* If you specify ``ForceApplyCapacityChange``, connections that prevent Aurora Serverless v1 from finding a scaling point might be dropped.
* For more information, see [Autoscaling for Aurora Serverless v1](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless.how-it-works.html#aurora-serverless.how-it-works.auto-scaling) in the *Amazon Aurora User Guide*.
*/
public data class DbClusterScalingConfigurationArgs(
public val autoPause: Output? = null,
public val maxCapacity: Output? = null,
public val minCapacity: Output? = null,
public val secondsBeforeTimeout: Output? = null,
public val secondsUntilAutoPause: Output? = null,
public val timeoutAction: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.rds.inputs.DbClusterScalingConfigurationArgs =
com.pulumi.awsnative.rds.inputs.DbClusterScalingConfigurationArgs.builder()
.autoPause(autoPause?.applyValue({ args0 -> args0 }))
.maxCapacity(maxCapacity?.applyValue({ args0 -> args0 }))
.minCapacity(minCapacity?.applyValue({ args0 -> args0 }))
.secondsBeforeTimeout(secondsBeforeTimeout?.applyValue({ args0 -> args0 }))
.secondsUntilAutoPause(secondsUntilAutoPause?.applyValue({ args0 -> args0 }))
.timeoutAction(timeoutAction?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DbClusterScalingConfigurationArgs].
*/
@PulumiTagMarker
public class DbClusterScalingConfigurationArgsBuilder internal constructor() {
private var autoPause: Output? = null
private var maxCapacity: Output? = null
private var minCapacity: Output? = null
private var secondsBeforeTimeout: Output? = null
private var secondsUntilAutoPause: Output? = null
private var timeoutAction: Output? = null
/**
* @param value Indicates whether to allow or disallow automatic pause for an Aurora DB cluster in ``serverless`` DB engine mode. A DB cluster can be paused only when it's idle (it has no connections).
* If a DB cluster is paused for more than seven days, the DB cluster might be backed up with a snapshot. In this case, the DB cluster is restored when there is a request to connect to it.
*/
@JvmName("juilteykytifradu")
public suspend fun autoPause(`value`: Output) {
this.autoPause = value
}
/**
* @param value The maximum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The maximum capacity must be greater than or equal to the minimum capacity.
*/
@JvmName("vkylwoycrvwtmtwr")
public suspend fun maxCapacity(`value`: Output) {
this.maxCapacity = value
}
/**
* @param value The minimum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The minimum capacity must be less than or equal to the maximum capacity.
*/
@JvmName("maxyosgdasvfgtpn")
public suspend fun minCapacity(`value`: Output) {
this.minCapacity = value
}
/**
* @param value The amount of time, in seconds, that Aurora Serverless v1 tries to find a scaling point to perform seamless scaling before enforcing the timeout action. The default is 300.
* Specify a value between 60 and 600 seconds.
*/
@JvmName("rnjcyutiywtmguof")
public suspend fun secondsBeforeTimeout(`value`: Output) {
this.secondsBeforeTimeout = value
}
/**
* @param value The time, in seconds, before an Aurora DB cluster in ``serverless`` mode is paused.
* Specify a value between 300 and 86,400 seconds.
*/
@JvmName("keaxciyeftjobkjm")
public suspend fun secondsUntilAutoPause(`value`: Output) {
this.secondsUntilAutoPause = value
}
/**
* @param value The action to take when the timeout is reached, either ``ForceApplyCapacityChange`` or ``RollbackCapacityChange``.
* ``ForceApplyCapacityChange`` sets the capacity to the specified value as soon as possible.
* ``RollbackCapacityChange``, the default, ignores the capacity change if a scaling point isn't found in the timeout period.
* If you specify ``ForceApplyCapacityChange``, connections that prevent Aurora Serverless v1 from finding a scaling point might be dropped.
* For more information, see [Autoscaling for Aurora Serverless v1](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless.how-it-works.html#aurora-serverless.how-it-works.auto-scaling) in the *Amazon Aurora User Guide*.
*/
@JvmName("dxtsdalasydioqrg")
public suspend fun timeoutAction(`value`: Output) {
this.timeoutAction = value
}
/**
* @param value Indicates whether to allow or disallow automatic pause for an Aurora DB cluster in ``serverless`` DB engine mode. A DB cluster can be paused only when it's idle (it has no connections).
* If a DB cluster is paused for more than seven days, the DB cluster might be backed up with a snapshot. In this case, the DB cluster is restored when there is a request to connect to it.
*/
@JvmName("iborbrftkaqqqlag")
public suspend fun autoPause(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoPause = mapped
}
/**
* @param value The maximum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The maximum capacity must be greater than or equal to the minimum capacity.
*/
@JvmName("snkjphieagirrepr")
public suspend fun maxCapacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxCapacity = mapped
}
/**
* @param value The minimum capacity for an Aurora DB cluster in ``serverless`` DB engine mode.
* For Aurora MySQL, valid capacity values are ``1``, ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``128``, and ``256``.
* For Aurora PostgreSQL, valid capacity values are ``2``, ``4``, ``8``, ``16``, ``32``, ``64``, ``192``, and ``384``.
* The minimum capacity must be less than or equal to the maximum capacity.
*/
@JvmName("qxvkdktniftddnbb")
public suspend fun minCapacity(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minCapacity = mapped
}
/**
* @param value The amount of time, in seconds, that Aurora Serverless v1 tries to find a scaling point to perform seamless scaling before enforcing the timeout action. The default is 300.
* Specify a value between 60 and 600 seconds.
*/
@JvmName("sjwvkowpnjnqyjys")
public suspend fun secondsBeforeTimeout(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondsBeforeTimeout = mapped
}
/**
* @param value The time, in seconds, before an Aurora DB cluster in ``serverless`` mode is paused.
* Specify a value between 300 and 86,400 seconds.
*/
@JvmName("mkbuvntaueoqfwyy")
public suspend fun secondsUntilAutoPause(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secondsUntilAutoPause = mapped
}
/**
* @param value The action to take when the timeout is reached, either ``ForceApplyCapacityChange`` or ``RollbackCapacityChange``.
* ``ForceApplyCapacityChange`` sets the capacity to the specified value as soon as possible.
* ``RollbackCapacityChange``, the default, ignores the capacity change if a scaling point isn't found in the timeout period.
* If you specify ``ForceApplyCapacityChange``, connections that prevent Aurora Serverless v1 from finding a scaling point might be dropped.
* For more information, see [Autoscaling for Aurora Serverless v1](https://docs.aws.amazon.com/AmazonRDS/latest/AuroraUserGuide/aurora-serverless.how-it-works.html#aurora-serverless.how-it-works.auto-scaling) in the *Amazon Aurora User Guide*.
*/
@JvmName("sqwwdmhictqmqooy")
public suspend fun timeoutAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutAction = mapped
}
internal fun build(): DbClusterScalingConfigurationArgs = DbClusterScalingConfigurationArgs(
autoPause = autoPause,
maxCapacity = maxCapacity,
minCapacity = minCapacity,
secondsBeforeTimeout = secondsBeforeTimeout,
secondsUntilAutoPause = secondsUntilAutoPause,
timeoutAction = timeoutAction,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy