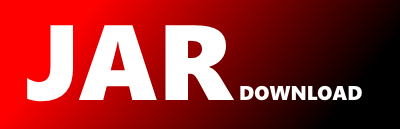
com.pulumi.awsnative.redshift.kotlin.EndpointAccess.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.redshift.kotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.EndpointAccessVpcSecurityGroup
import com.pulumi.awsnative.redshift.kotlin.outputs.VpcEndpointProperties
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.redshift.kotlin.outputs.EndpointAccessVpcSecurityGroup.Companion.toKotlin as endpointAccessVpcSecurityGroupToKotlin
import com.pulumi.awsnative.redshift.kotlin.outputs.VpcEndpointProperties.Companion.toKotlin as vpcEndpointPropertiesToKotlin
/**
* Builder for [EndpointAccess].
*/
@PulumiTagMarker
public class EndpointAccessResourceBuilder internal constructor() {
public var name: String? = null
public var args: EndpointAccessArgs = EndpointAccessArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EndpointAccessArgsBuilder.() -> Unit) {
val builder = EndpointAccessArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): EndpointAccess {
val builtJavaResource = com.pulumi.awsnative.redshift.EndpointAccess(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return EndpointAccess(builtJavaResource)
}
}
/**
* Resource schema for a Redshift-managed VPC endpoint.
*/
public class EndpointAccess internal constructor(
override val javaResource: com.pulumi.awsnative.redshift.EndpointAccess,
) : KotlinCustomResource(javaResource, EndpointAccessMapper) {
/**
* The DNS address of the endpoint.
*/
public val address: Output
get() = javaResource.address().applyValue({ args0 -> args0 })
/**
* A unique identifier for the cluster. You use this identifier to refer to the cluster for any subsequent cluster operations such as deleting or modifying. All alphabetical characters must be lower case, no hypens at the end, no two consecutive hyphens. Cluster name should be unique for all clusters within an AWS account
*/
public val clusterIdentifier: Output
get() = javaResource.clusterIdentifier().applyValue({ args0 -> args0 })
/**
* The time (UTC) that the endpoint was created.
*/
public val endpointCreateTime: Output
get() = javaResource.endpointCreateTime().applyValue({ args0 -> args0 })
/**
* The name of the endpoint.
*/
public val endpointName: Output
get() = javaResource.endpointName().applyValue({ args0 -> args0 })
/**
* The status of the endpoint.
*/
public val endpointStatus: Output
get() = javaResource.endpointStatus().applyValue({ args0 -> args0 })
/**
* The port number on which the cluster accepts incoming connections.
*/
public val port: Output
get() = javaResource.port().applyValue({ args0 -> args0 })
/**
* The AWS account ID of the owner of the cluster.
*/
public val resourceOwner: Output?
get() = javaResource.resourceOwner().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The subnet group name where Amazon Redshift chooses to deploy the endpoint.
*/
public val subnetGroupName: Output
get() = javaResource.subnetGroupName().applyValue({ args0 -> args0 })
/**
* The connection endpoint for connecting to an Amazon Redshift cluster through the proxy.
*/
public val vpcEndpoint: Output
get() = javaResource.vpcEndpoint().applyValue({ args0 ->
args0.let({ args0 ->
vpcEndpointPropertiesToKotlin(args0)
})
})
/**
* A list of vpc security group ids to apply to the created endpoint access.
*/
public val vpcSecurityGroupIds: Output>
get() = javaResource.vpcSecurityGroupIds().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* A list of Virtual Private Cloud (VPC) security groups to be associated with the endpoint.
*/
public val vpcSecurityGroups: Output>
get() = javaResource.vpcSecurityGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> endpointAccessVpcSecurityGroupToKotlin(args0) })
})
})
}
public object EndpointAccessMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.redshift.EndpointAccess::class == javaResource::class
override fun map(javaResource: Resource): EndpointAccess = EndpointAccess(
javaResource as
com.pulumi.awsnative.redshift.EndpointAccess,
)
}
/**
* @see [EndpointAccess].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [EndpointAccess].
*/
public suspend fun endpointAccess(
name: String,
block: suspend EndpointAccessResourceBuilder.() -> Unit,
): EndpointAccess {
val builder = EndpointAccessResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [EndpointAccess].
* @param name The _unique_ name of the resulting resource.
*/
public fun endpointAccess(name: String): EndpointAccess {
val builder = EndpointAccessResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy