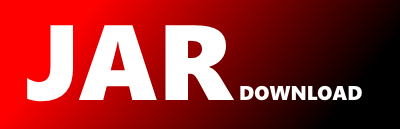
com.pulumi.awsnative.redshift.kotlin.ScheduledActionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.redshift.kotlin
import com.pulumi.awsnative.redshift.ScheduledActionArgs.builder
import com.pulumi.awsnative.redshift.kotlin.inputs.ScheduledActionTypeArgs
import com.pulumi.awsnative.redshift.kotlin.inputs.ScheduledActionTypeArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The `AWS::Redshift::ScheduledAction` resource creates an Amazon Redshift Scheduled Action.
* @property enable If true, the schedule is enabled. If false, the scheduled action does not trigger.
* @property endTime The end time in UTC of the scheduled action. After this time, the scheduled action does not trigger.
* @property iamRole The IAM role to assume to run the target action.
* @property schedule The schedule in `at( )` or `cron( )` format.
* @property scheduledActionDescription The description of the scheduled action.
* @property scheduledActionName The name of the scheduled action. The name must be unique within an account.
* @property startTime The start time in UTC of the scheduled action. Before this time, the scheduled action does not trigger.
* @property targetAction A JSON format string of the Amazon Redshift API operation with input parameters.
*/
public data class ScheduledActionArgs(
public val enable: Output? = null,
public val endTime: Output? = null,
public val iamRole: Output? = null,
public val schedule: Output? = null,
public val scheduledActionDescription: Output? = null,
public val scheduledActionName: Output? = null,
public val startTime: Output? = null,
public val targetAction: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.redshift.ScheduledActionArgs =
com.pulumi.awsnative.redshift.ScheduledActionArgs.builder()
.enable(enable?.applyValue({ args0 -> args0 }))
.endTime(endTime?.applyValue({ args0 -> args0 }))
.iamRole(iamRole?.applyValue({ args0 -> args0 }))
.schedule(schedule?.applyValue({ args0 -> args0 }))
.scheduledActionDescription(scheduledActionDescription?.applyValue({ args0 -> args0 }))
.scheduledActionName(scheduledActionName?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 }))
.targetAction(targetAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ScheduledActionArgs].
*/
@PulumiTagMarker
public class ScheduledActionArgsBuilder internal constructor() {
private var enable: Output? = null
private var endTime: Output? = null
private var iamRole: Output? = null
private var schedule: Output? = null
private var scheduledActionDescription: Output? = null
private var scheduledActionName: Output? = null
private var startTime: Output? = null
private var targetAction: Output? = null
/**
* @param value If true, the schedule is enabled. If false, the scheduled action does not trigger.
*/
@JvmName("dyqwuvmwxybgrocy")
public suspend fun enable(`value`: Output) {
this.enable = value
}
/**
* @param value The end time in UTC of the scheduled action. After this time, the scheduled action does not trigger.
*/
@JvmName("rtbommsdkphswcnq")
public suspend fun endTime(`value`: Output) {
this.endTime = value
}
/**
* @param value The IAM role to assume to run the target action.
*/
@JvmName("ucjlscwrkcqaqrwv")
public suspend fun iamRole(`value`: Output) {
this.iamRole = value
}
/**
* @param value The schedule in `at( )` or `cron( )` format.
*/
@JvmName("umbxpkaenhgqrdee")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value The description of the scheduled action.
*/
@JvmName("dphtuxuhbgfjdhad")
public suspend fun scheduledActionDescription(`value`: Output) {
this.scheduledActionDescription = value
}
/**
* @param value The name of the scheduled action. The name must be unique within an account.
*/
@JvmName("pelocafhdofbrifr")
public suspend fun scheduledActionName(`value`: Output) {
this.scheduledActionName = value
}
/**
* @param value The start time in UTC of the scheduled action. Before this time, the scheduled action does not trigger.
*/
@JvmName("nyxtegenixxcjjyj")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value A JSON format string of the Amazon Redshift API operation with input parameters.
*/
@JvmName("shlgxnfmsiydjcwu")
public suspend fun targetAction(`value`: Output) {
this.targetAction = value
}
/**
* @param value If true, the schedule is enabled. If false, the scheduled action does not trigger.
*/
@JvmName("lattghhnxogaefgx")
public suspend fun enable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enable = mapped
}
/**
* @param value The end time in UTC of the scheduled action. After this time, the scheduled action does not trigger.
*/
@JvmName("yknoajtxskdhfpno")
public suspend fun endTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endTime = mapped
}
/**
* @param value The IAM role to assume to run the target action.
*/
@JvmName("uhqehfkwhyfmbsti")
public suspend fun iamRole(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.iamRole = mapped
}
/**
* @param value The schedule in `at( )` or `cron( )` format.
*/
@JvmName("uehftjvtkksqpplr")
public suspend fun schedule(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param value The description of the scheduled action.
*/
@JvmName("kwkbdippsqcisoar")
public suspend fun scheduledActionDescription(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduledActionDescription = mapped
}
/**
* @param value The name of the scheduled action. The name must be unique within an account.
*/
@JvmName("joqtpeihthhafhei")
public suspend fun scheduledActionName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduledActionName = mapped
}
/**
* @param value The start time in UTC of the scheduled action. Before this time, the scheduled action does not trigger.
*/
@JvmName("jcvloimrntbamroi")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
/**
* @param value A JSON format string of the Amazon Redshift API operation with input parameters.
*/
@JvmName("usodvvvemtjveidp")
public suspend fun targetAction(`value`: ScheduledActionTypeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetAction = mapped
}
/**
* @param argument A JSON format string of the Amazon Redshift API operation with input parameters.
*/
@JvmName("yqdacatquxgedfvk")
public suspend fun targetAction(argument: suspend ScheduledActionTypeArgsBuilder.() -> Unit) {
val toBeMapped = ScheduledActionTypeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.targetAction = mapped
}
internal fun build(): ScheduledActionArgs = ScheduledActionArgs(
enable = enable,
endTime = endTime,
iamRole = iamRole,
schedule = schedule,
scheduledActionDescription = scheduledActionDescription,
scheduledActionName = scheduledActionName,
startTime = startTime,
targetAction = targetAction,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy