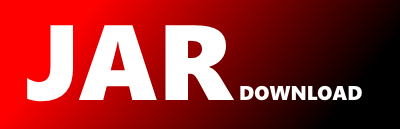
com.pulumi.awsnative.route53.kotlin.Route53Functions.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.route53.kotlin
import com.pulumi.awsnative.route53.Route53Functions.getCidrCollectionPlain
import com.pulumi.awsnative.route53.Route53Functions.getHealthCheckPlain
import com.pulumi.awsnative.route53.Route53Functions.getHostedZonePlain
import com.pulumi.awsnative.route53.Route53Functions.getKeySigningKeyPlain
import com.pulumi.awsnative.route53.kotlin.inputs.GetCidrCollectionPlainArgs
import com.pulumi.awsnative.route53.kotlin.inputs.GetCidrCollectionPlainArgsBuilder
import com.pulumi.awsnative.route53.kotlin.inputs.GetHealthCheckPlainArgs
import com.pulumi.awsnative.route53.kotlin.inputs.GetHealthCheckPlainArgsBuilder
import com.pulumi.awsnative.route53.kotlin.inputs.GetHostedZonePlainArgs
import com.pulumi.awsnative.route53.kotlin.inputs.GetHostedZonePlainArgsBuilder
import com.pulumi.awsnative.route53.kotlin.inputs.GetKeySigningKeyPlainArgs
import com.pulumi.awsnative.route53.kotlin.inputs.GetKeySigningKeyPlainArgsBuilder
import com.pulumi.awsnative.route53.kotlin.outputs.GetCidrCollectionResult
import com.pulumi.awsnative.route53.kotlin.outputs.GetHealthCheckResult
import com.pulumi.awsnative.route53.kotlin.outputs.GetHostedZoneResult
import com.pulumi.awsnative.route53.kotlin.outputs.GetKeySigningKeyResult
import kotlinx.coroutines.future.await
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.awsnative.route53.kotlin.outputs.GetCidrCollectionResult.Companion.toKotlin as getCidrCollectionResultToKotlin
import com.pulumi.awsnative.route53.kotlin.outputs.GetHealthCheckResult.Companion.toKotlin as getHealthCheckResultToKotlin
import com.pulumi.awsnative.route53.kotlin.outputs.GetHostedZoneResult.Companion.toKotlin as getHostedZoneResultToKotlin
import com.pulumi.awsnative.route53.kotlin.outputs.GetKeySigningKeyResult.Companion.toKotlin as getKeySigningKeyResultToKotlin
public object Route53Functions {
/**
* Resource schema for AWS::Route53::CidrCollection.
* @param argument null
* @return null
*/
public suspend fun getCidrCollection(argument: GetCidrCollectionPlainArgs): GetCidrCollectionResult =
getCidrCollectionResultToKotlin(getCidrCollectionPlain(argument.toJava()).await())
/**
* @see [getCidrCollection].
* @param id UUID of the CIDR collection.
* @return null
*/
public suspend fun getCidrCollection(id: String): GetCidrCollectionResult {
val argument = GetCidrCollectionPlainArgs(
id = id,
)
return getCidrCollectionResultToKotlin(getCidrCollectionPlain(argument.toJava()).await())
}
/**
* @see [getCidrCollection].
* @param argument Builder for [com.pulumi.awsnative.route53.kotlin.inputs.GetCidrCollectionPlainArgs].
* @return null
*/
public suspend fun getCidrCollection(argument: suspend GetCidrCollectionPlainArgsBuilder.() -> Unit): GetCidrCollectionResult {
val builder = GetCidrCollectionPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getCidrCollectionResultToKotlin(getCidrCollectionPlain(builtArgument.toJava()).await())
}
/**
* Resource schema for AWS::Route53::HealthCheck.
* @param argument null
* @return null
*/
public suspend fun getHealthCheck(argument: GetHealthCheckPlainArgs): GetHealthCheckResult =
getHealthCheckResultToKotlin(getHealthCheckPlain(argument.toJava()).await())
/**
* @see [getHealthCheck].
* @param healthCheckId The identifier that Amazon Route 53 assigned to the health check when you created it. When you add or update a resource record set, you use this value to specify which health check to use. The value can be up to 64 characters long.
* @return null
*/
public suspend fun getHealthCheck(healthCheckId: String): GetHealthCheckResult {
val argument = GetHealthCheckPlainArgs(
healthCheckId = healthCheckId,
)
return getHealthCheckResultToKotlin(getHealthCheckPlain(argument.toJava()).await())
}
/**
* @see [getHealthCheck].
* @param argument Builder for [com.pulumi.awsnative.route53.kotlin.inputs.GetHealthCheckPlainArgs].
* @return null
*/
public suspend fun getHealthCheck(argument: suspend GetHealthCheckPlainArgsBuilder.() -> Unit): GetHealthCheckResult {
val builder = GetHealthCheckPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getHealthCheckResultToKotlin(getHealthCheckPlain(builtArgument.toJava()).await())
}
/**
* Creates a new public or private hosted zone. You create records in a public hosted zone to define how you want to route traffic on the internet for a domain, such as example.com, and its subdomains (apex.example.com, acme.example.com). You create records in a private hosted zone to define how you want to route traffic for a domain and its subdomains within one or more Amazon Virtual Private Clouds (Amazon VPCs).
* You can't convert a public hosted zone to a private hosted zone or vice versa. Instead, you must create a new hosted zone with the same name and create new resource record sets.
* For more information about charges for hosted zones, see [Amazon Route 53 Pricing](https://docs.aws.amazon.com/route53/pricing/).
* Note the following:
* + You can't create a hosted zone for a top-level domain (TLD) such as .com.
* + If your domain is registered with a registrar other than Route 53, you must update the name servers with your registrar to make Route 53 the DNS service for the domain. For more information, see [Migrating DNS Service for an Existing Domain to Amazon Route 53](https://docs.aws.amazon.com/Route53/latest/DeveloperGuide/MigratingDNS.html) in the *Amazon Route 53 Developer Guide*.
* When you submit a ``CreateHostedZone`` request, the initial status of the hosted zone is ``PENDING``. For public hosted zones, this means that the NS and SOA records are not yet available on all Route 53 DNS servers. When the NS and SOA records are available, the status of the zone changes to ``INSYNC``.
* The ``CreateHostedZone`` request requires the caller to have an ``ec2:DescribeVpcs`` permission.
* When creating private hosted zones, the Amazon VPC must belong to the same partition where the hosted zone is created. A partition is a group of AWS-Regions. Each AWS-account is scoped to one partition.
* The following are the supported partitions:
* + ``aws`` - AWS-Regions
* + ``aws-cn`` - China Regions
* + ``aws-us-gov`` - govcloud-us-region
* For more information, see [Access Management](https://docs.aws.amazon.com/general/latest/gr/aws-arns-and-namespaces.html) in the *General Reference*.
* @param argument null
* @return null
*/
public suspend fun getHostedZone(argument: GetHostedZonePlainArgs): GetHostedZoneResult =
getHostedZoneResultToKotlin(getHostedZonePlain(argument.toJava()).await())
/**
* @see [getHostedZone].
* @param id The ID that Amazon Route 53 assigned to the hosted zone when you created it.
* @return null
*/
public suspend fun getHostedZone(id: String): GetHostedZoneResult {
val argument = GetHostedZonePlainArgs(
id = id,
)
return getHostedZoneResultToKotlin(getHostedZonePlain(argument.toJava()).await())
}
/**
* @see [getHostedZone].
* @param argument Builder for [com.pulumi.awsnative.route53.kotlin.inputs.GetHostedZonePlainArgs].
* @return null
*/
public suspend fun getHostedZone(argument: suspend GetHostedZonePlainArgsBuilder.() -> Unit): GetHostedZoneResult {
val builder = GetHostedZonePlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getHostedZoneResultToKotlin(getHostedZonePlain(builtArgument.toJava()).await())
}
/**
* Represents a key signing key (KSK) associated with a hosted zone. You can only have two KSKs per hosted zone.
* @param argument null
* @return null
*/
public suspend fun getKeySigningKey(argument: GetKeySigningKeyPlainArgs): GetKeySigningKeyResult =
getKeySigningKeyResultToKotlin(getKeySigningKeyPlain(argument.toJava()).await())
/**
* @see [getKeySigningKey].
* @param hostedZoneId The unique string (ID) used to identify a hosted zone.
* @param name An alphanumeric string used to identify a key signing key (KSK). Name must be unique for each key signing key in the same hosted zone.
* @return null
*/
public suspend fun getKeySigningKey(hostedZoneId: String, name: String): GetKeySigningKeyResult {
val argument = GetKeySigningKeyPlainArgs(
hostedZoneId = hostedZoneId,
name = name,
)
return getKeySigningKeyResultToKotlin(getKeySigningKeyPlain(argument.toJava()).await())
}
/**
* @see [getKeySigningKey].
* @param argument Builder for [com.pulumi.awsnative.route53.kotlin.inputs.GetKeySigningKeyPlainArgs].
* @return null
*/
public suspend fun getKeySigningKey(argument: suspend GetKeySigningKeyPlainArgsBuilder.() -> Unit): GetKeySigningKeyResult {
val builder = GetKeySigningKeyPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKeySigningKeyResultToKotlin(getKeySigningKeyPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy