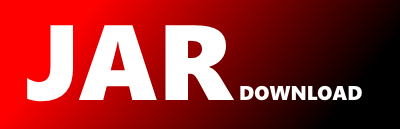
com.pulumi.awsnative.route53recoverycontrol.kotlin.SafetyRuleArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.route53recoverycontrol.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.route53recoverycontrol.SafetyRuleArgs.builder
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleAssertionRuleArgs
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleAssertionRuleArgsBuilder
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleGatingRuleArgs
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleGatingRuleArgsBuilder
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleRuleConfigArgs
import com.pulumi.awsnative.route53recoverycontrol.kotlin.inputs.SafetyRuleRuleConfigArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource schema for AWS Route53 Recovery Control basic constructs and validation rules.
* @property assertionRule An assertion rule enforces that, when you change a routing control state, that the criteria that you set in the rule configuration is met. Otherwise, the change to the routing control is not accepted. For example, the criteria might be that at least one routing control state is `On` after the transaction so that traffic continues to flow to at least one cell for the application. This ensures that you avoid a fail-open scenario.
* @property controlPanelArn The Amazon Resource Name (ARN) of the control panel.
* @property gatingRule A gating rule verifies that a gating routing control or set of gating routing controls, evaluates as true, based on a rule configuration that you specify, which allows a set of routing control state changes to complete.
* For example, if you specify one gating routing control and you set the `Type` in the rule configuration to `OR` , that indicates that you must set the gating routing control to `On` for the rule to evaluate as true; that is, for the gating control switch to be On. When you do that, then you can update the routing control states for the target routing controls that you specify in the gating rule.
* @property name The name of the assertion rule. The name must be unique within a control panel. You can use any non-white space character in the name except the following: & > < ' (single quote) " (double quote) ; (semicolon)
* @property ruleConfig The criteria that you set for specific assertion controls (routing controls) that designate how many control states must be `ON` as the result of a transaction. For example, if you have three assertion controls, you might specify `ATLEAST 2` for your rule configuration. This means that at least two assertion controls must be `ON` , so that at least two AWS Regions have traffic flowing to them.
* @property tags A collection of tags associated with a resource
*/
public data class SafetyRuleArgs(
public val assertionRule: Output? = null,
public val controlPanelArn: Output? = null,
public val gatingRule: Output? = null,
public val name: Output? = null,
public val ruleConfig: Output? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.route53recoverycontrol.SafetyRuleArgs =
com.pulumi.awsnative.route53recoverycontrol.SafetyRuleArgs.builder()
.assertionRule(assertionRule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.controlPanelArn(controlPanelArn?.applyValue({ args0 -> args0 }))
.gatingRule(gatingRule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.ruleConfig(ruleConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [SafetyRuleArgs].
*/
@PulumiTagMarker
public class SafetyRuleArgsBuilder internal constructor() {
private var assertionRule: Output? = null
private var controlPanelArn: Output? = null
private var gatingRule: Output? = null
private var name: Output? = null
private var ruleConfig: Output? = null
private var tags: Output>? = null
/**
* @param value An assertion rule enforces that, when you change a routing control state, that the criteria that you set in the rule configuration is met. Otherwise, the change to the routing control is not accepted. For example, the criteria might be that at least one routing control state is `On` after the transaction so that traffic continues to flow to at least one cell for the application. This ensures that you avoid a fail-open scenario.
*/
@JvmName("ecduphejxcptikea")
public suspend fun assertionRule(`value`: Output) {
this.assertionRule = value
}
/**
* @param value The Amazon Resource Name (ARN) of the control panel.
*/
@JvmName("kaonmnlefcebnfrt")
public suspend fun controlPanelArn(`value`: Output) {
this.controlPanelArn = value
}
/**
* @param value A gating rule verifies that a gating routing control or set of gating routing controls, evaluates as true, based on a rule configuration that you specify, which allows a set of routing control state changes to complete.
* For example, if you specify one gating routing control and you set the `Type` in the rule configuration to `OR` , that indicates that you must set the gating routing control to `On` for the rule to evaluate as true; that is, for the gating control switch to be On. When you do that, then you can update the routing control states for the target routing controls that you specify in the gating rule.
*/
@JvmName("thgrmyonkrxaovuo")
public suspend fun gatingRule(`value`: Output) {
this.gatingRule = value
}
/**
* @param value The name of the assertion rule. The name must be unique within a control panel. You can use any non-white space character in the name except the following: & > < ' (single quote) " (double quote) ; (semicolon)
*/
@JvmName("qpvfoydfsqosvyoy")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The criteria that you set for specific assertion controls (routing controls) that designate how many control states must be `ON` as the result of a transaction. For example, if you have three assertion controls, you might specify `ATLEAST 2` for your rule configuration. This means that at least two assertion controls must be `ON` , so that at least two AWS Regions have traffic flowing to them.
*/
@JvmName("yrkyvvfpxyetvsqc")
public suspend fun ruleConfig(`value`: Output) {
this.ruleConfig = value
}
/**
* @param value A collection of tags associated with a resource
*/
@JvmName("yogqntvuhaslcnta")
public suspend fun tags(`value`: Output>) {
this.tags = value
}
@JvmName("fxugtefibxesaecp")
public suspend fun tags(vararg values: Output) {
this.tags = Output.all(values.asList())
}
/**
* @param values A collection of tags associated with a resource
*/
@JvmName("xenteevadwvtaicj")
public suspend fun tags(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy