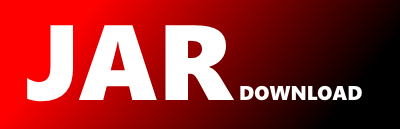
com.pulumi.awsnative.route53recoveryreadiness.kotlin.ResourceSetArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.route53recoveryreadiness.kotlin
import com.pulumi.awsnative.kotlin.inputs.TagArgs
import com.pulumi.awsnative.kotlin.inputs.TagArgsBuilder
import com.pulumi.awsnative.route53recoveryreadiness.ResourceSetArgs.builder
import com.pulumi.awsnative.route53recoveryreadiness.kotlin.inputs.ResourceSetResourceArgs
import com.pulumi.awsnative.route53recoveryreadiness.kotlin.inputs.ResourceSetResourceArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Schema for the AWS Route53 Recovery Readiness ResourceSet Resource and API.
* @property resourceSetName The name of the resource set to create.
* @property resourceSetType The resource type of the resources in the resource set. Enter one of the following values for resource type:
* AWS: :AutoScaling: :AutoScalingGroup, AWS: :CloudWatch: :Alarm, AWS: :EC2: :CustomerGateway, AWS: :DynamoDB: :Table, AWS: :EC2: :Volume, AWS: :ElasticLoadBalancing: :LoadBalancer, AWS: :ElasticLoadBalancingV2: :LoadBalancer, AWS: :MSK: :Cluster, AWS: :RDS: :DBCluster, AWS: :Route53: :HealthCheck, AWS: :SQS: :Queue, AWS: :SNS: :Topic, AWS: :SNS: :Subscription, AWS: :EC2: :VPC, AWS: :EC2: :VPNConnection, AWS: :EC2: :VPNGateway, AWS::Route53RecoveryReadiness::DNSTargetResource
* @property resources A list of resource objects in the resource set.
* @property tags A tag to associate with the parameters for a resource set.
*/
public data class ResourceSetArgs(
public val resourceSetName: Output? = null,
public val resourceSetType: Output? = null,
public val resources: Output>? = null,
public val tags: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.route53recoveryreadiness.ResourceSetArgs =
com.pulumi.awsnative.route53recoveryreadiness.ResourceSetArgs.builder()
.resourceSetName(resourceSetName?.applyValue({ args0 -> args0 }))
.resourceSetType(resourceSetType?.applyValue({ args0 -> args0 }))
.resources(
resources?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.tags(
tags?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ResourceSetArgs].
*/
@PulumiTagMarker
public class ResourceSetArgsBuilder internal constructor() {
private var resourceSetName: Output? = null
private var resourceSetType: Output? = null
private var resources: Output>? = null
private var tags: Output>? = null
/**
* @param value The name of the resource set to create.
*/
@JvmName("vqtutbcjjtilxgkr")
public suspend fun resourceSetName(`value`: Output) {
this.resourceSetName = value
}
/**
* @param value The resource type of the resources in the resource set. Enter one of the following values for resource type:
* AWS: :AutoScaling: :AutoScalingGroup, AWS: :CloudWatch: :Alarm, AWS: :EC2: :CustomerGateway, AWS: :DynamoDB: :Table, AWS: :EC2: :Volume, AWS: :ElasticLoadBalancing: :LoadBalancer, AWS: :ElasticLoadBalancingV2: :LoadBalancer, AWS: :MSK: :Cluster, AWS: :RDS: :DBCluster, AWS: :Route53: :HealthCheck, AWS: :SQS: :Queue, AWS: :SNS: :Topic, AWS: :SNS: :Subscription, AWS: :EC2: :VPC, AWS: :EC2: :VPNConnection, AWS: :EC2: :VPNGateway, AWS::Route53RecoveryReadiness::DNSTargetResource
*/
@JvmName("ynqppohwkafbcbtf")
public suspend fun resourceSetType(`value`: Output) {
this.resourceSetType = value
}
/**
* @param value A list of resource objects in the resource set.
*/
@JvmName("ljgbimfajggenrsl")
public suspend fun resources(`value`: Output>) {
this.resources = value
}
@JvmName("dufyfotgppwjlcep")
public suspend fun resources(vararg values: Output) {
this.resources = Output.all(values.asList())
}
/**
* @param values A list of resource objects in the resource set.
*/
@JvmName("bywaapeqrtaemnvy")
public suspend fun resources(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy