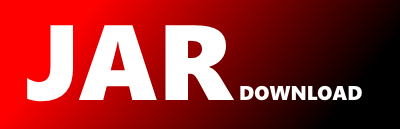
com.pulumi.awsnative.s3.kotlin.Bucket.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.s3.kotlin.enums.BucketAccessControl
import com.pulumi.awsnative.s3.kotlin.outputs.BucketAccelerateConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketAnalyticsConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketCorsConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketEncryption
import com.pulumi.awsnative.s3.kotlin.outputs.BucketIntelligentTieringConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketInventoryConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketLifecycleConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketLoggingConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketMetricsConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketNotificationConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketObjectLockConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketOwnershipControls
import com.pulumi.awsnative.s3.kotlin.outputs.BucketPublicAccessBlockConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketReplicationConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketVersioningConfiguration
import com.pulumi.awsnative.s3.kotlin.outputs.BucketWebsiteConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.s3.kotlin.enums.BucketAccessControl.Companion.toKotlin as bucketAccessControlToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketAccelerateConfiguration.Companion.toKotlin as bucketAccelerateConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketAnalyticsConfiguration.Companion.toKotlin as bucketAnalyticsConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketCorsConfiguration.Companion.toKotlin as bucketCorsConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketEncryption.Companion.toKotlin as bucketEncryptionToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketIntelligentTieringConfiguration.Companion.toKotlin as bucketIntelligentTieringConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketInventoryConfiguration.Companion.toKotlin as bucketInventoryConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketLifecycleConfiguration.Companion.toKotlin as bucketLifecycleConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketLoggingConfiguration.Companion.toKotlin as bucketLoggingConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketMetricsConfiguration.Companion.toKotlin as bucketMetricsConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketNotificationConfiguration.Companion.toKotlin as bucketNotificationConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketObjectLockConfiguration.Companion.toKotlin as bucketObjectLockConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketOwnershipControls.Companion.toKotlin as bucketOwnershipControlsToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketPublicAccessBlockConfiguration.Companion.toKotlin as bucketPublicAccessBlockConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketReplicationConfiguration.Companion.toKotlin as bucketReplicationConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketVersioningConfiguration.Companion.toKotlin as bucketVersioningConfigurationToKotlin
import com.pulumi.awsnative.s3.kotlin.outputs.BucketWebsiteConfiguration.Companion.toKotlin as bucketWebsiteConfigurationToKotlin
/**
* Builder for [Bucket].
*/
@PulumiTagMarker
public class BucketResourceBuilder internal constructor() {
public var name: String? = null
public var args: BucketArgs = BucketArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BucketArgsBuilder.() -> Unit) {
val builder = BucketArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Bucket {
val builtJavaResource = com.pulumi.awsnative.s3.Bucket(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Bucket(builtJavaResource)
}
}
/**
* The ``AWS::S3::Bucket`` resource creates an Amazon S3 bucket in the same AWS Region where you create the AWS CloudFormation stack.
* To control how AWS CloudFormation handles the bucket when the stack is deleted, you can set a deletion policy for your bucket. You can choose to *retain* the bucket or to *delete* the bucket. For more information, see [DeletionPolicy Attribute](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-attribute-deletionpolicy.html).
* You can only delete empty buckets. Deletion fails for buckets that have contents.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* ### Example
* No Java example available.
*/
public class Bucket internal constructor(
override val javaResource: com.pulumi.awsnative.s3.Bucket,
) : KotlinCustomResource(javaResource, BucketMapper) {
/**
* Configures the transfer acceleration state for an Amazon S3 bucket. For more information, see [Amazon S3 Transfer Acceleration](https://docs.aws.amazon.com/AmazonS3/latest/dev/transfer-acceleration.html) in the *Amazon S3 User Guide*.
*/
public val accelerateConfiguration: Output?
get() = javaResource.accelerateConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketAccelerateConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* This is a legacy property, and it is not recommended for most use cases. A majority of modern use cases in Amazon S3 no longer require the use of ACLs, and we recommend that you keep ACLs disabled. For more information, see [Controlling object ownership](https://docs.aws.amazon.com//AmazonS3/latest/userguide/about-object-ownership.html) in the *Amazon S3 User Guide*.
* A canned access control list (ACL) that grants predefined permissions to the bucket. For more information about canned ACLs, see [Canned ACL](https://docs.aws.amazon.com/AmazonS3/latest/dev/acl-overview.html#canned-acl) in the *Amazon S3 User Guide*.
* S3 buckets are created with ACLs disabled by default. Therefore, unless you explicitly set the [AWS::S3::OwnershipControls](https://docs.aws.amazon.com//AWSCloudFormation/latest/UserGuide/aws-properties-s3-bucket-ownershipcontrols.html) property to enable ACLs, your resource will fail to deploy with any value other than Private. Use cases requiring ACLs are uncommon.
* The majority of access control configurations can be successfully and more easily achieved with bucket policies. For more information, see [AWS::S3::BucketPolicy](https://docs.aws.amazon.com//AWSCloudFormation/latest/UserGuide/aws-properties-s3-policy.html). For examples of common policy configurations, including S3 Server Access Logs buckets and more, see [Bucket policy examples](https://docs.aws.amazon.com/AmazonS3/latest/userguide/example-bucket-policies.html) in the *Amazon S3 User Guide*.
*/
public val accessControl: Output?
get() = javaResource.accessControl().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketAccessControlToKotlin(args0) })
}).orElse(null)
})
/**
* Specifies the configuration and any analyses for the analytics filter of an Amazon S3 bucket.
*/
public val analyticsConfigurations: Output>?
get() = javaResource.analyticsConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketAnalyticsConfigurationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Returns the Amazon Resource Name (ARN) of the specified bucket.
* Example: `arn:aws:s3:::DOC-EXAMPLE-BUCKET`
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* Specifies default encryption for a bucket using server-side encryption with Amazon S3-managed keys (SSE-S3), AWS KMS-managed keys (SSE-KMS), or dual-layer server-side encryption with KMS-managed keys (DSSE-KMS). For information about the Amazon S3 default encryption feature, see [Amazon S3 Default Encryption for S3 Buckets](https://docs.aws.amazon.com/AmazonS3/latest/dev/bucket-encryption.html) in the *Amazon S3 User Guide*.
*/
public val bucketEncryption: Output?
get() = javaResource.bucketEncryption().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketEncryptionToKotlin(args0) })
}).orElse(null)
})
/**
* A name for the bucket. If you don't specify a name, AWS CloudFormation generates a unique ID and uses that ID for the bucket name. The bucket name must contain only lowercase letters, numbers, periods (.), and dashes (-) and must follow [Amazon S3 bucket restrictions and limitations](https://docs.aws.amazon.com/AmazonS3/latest/dev/BucketRestrictions.html). For more information, see [Rules for naming Amazon S3 buckets](https://docs.aws.amazon.com/AmazonS3/latest/dev/BucketRestrictions.html#bucketnamingrules) in the *Amazon S3 User Guide*.
* If you specify a name, you can't perform updates that require replacement of this resource. You can perform updates that require no or some interruption. If you need to replace the resource, specify a new name.
*/
public val bucketName: Output?
get() = javaResource.bucketName().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Describes the cross-origin access configuration for objects in an Amazon S3 bucket. For more information, see [Enabling Cross-Origin Resource Sharing](https://docs.aws.amazon.com/AmazonS3/latest/dev/cors.html) in the *Amazon S3 User Guide*.
*/
public val corsConfiguration: Output?
get() = javaResource.corsConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketCorsConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Returns the IPv4 DNS name of the specified bucket.
* Example: `DOC-EXAMPLE-BUCKET.s3.amazonaws.com`
*/
public val domainName: Output
get() = javaResource.domainName().applyValue({ args0 -> args0 })
/**
* Returns the IPv6 DNS name of the specified bucket.
* Example: `DOC-EXAMPLE-BUCKET.s3.dualstack.us-east-2.amazonaws.com`
* For more information about dual-stack endpoints, see [Using Amazon S3 Dual-Stack Endpoints](https://docs.aws.amazon.com/AmazonS3/latest/dev/dual-stack-endpoints.html) .
*/
public val dualStackDomainName: Output
get() = javaResource.dualStackDomainName().applyValue({ args0 -> args0 })
/**
* Defines how Amazon S3 handles Intelligent-Tiering storage.
*/
public val intelligentTieringConfigurations: Output>?
get() = javaResource.intelligentTieringConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketIntelligentTieringConfigurationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies the inventory configuration for an Amazon S3 bucket. For more information, see [GET Bucket inventory](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketGETInventoryConfig.html) in the *Amazon S3 API Reference*.
*/
public val inventoryConfigurations: Output>?
get() = javaResource.inventoryConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketInventoryConfigurationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Specifies the lifecycle configuration for objects in an Amazon S3 bucket. For more information, see [Object Lifecycle Management](https://docs.aws.amazon.com/AmazonS3/latest/dev/object-lifecycle-mgmt.html) in the *Amazon S3 User Guide*.
*/
public val lifecycleConfiguration: Output?
get() = javaResource.lifecycleConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketLifecycleConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Settings that define where logs are stored.
*/
public val loggingConfiguration: Output?
get() = javaResource.loggingConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketLoggingConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Specifies a metrics configuration for the CloudWatch request metrics (specified by the metrics configuration ID) from an Amazon S3 bucket. If you're updating an existing metrics configuration, note that this is a full replacement of the existing metrics configuration. If you don't include the elements you want to keep, they are erased. For more information, see [PutBucketMetricsConfiguration](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketPUTMetricConfiguration.html).
*/
public val metricsConfigurations: Output>?
get() = javaResource.metricsConfigurations().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
bucketMetricsConfigurationToKotlin(args0)
})
})
}).orElse(null)
})
/**
* Configuration that defines how Amazon S3 handles bucket notifications.
*/
public val notificationConfiguration: Output?
get() = javaResource.notificationConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketNotificationConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* This operation is not supported by directory buckets.
* Places an Object Lock configuration on the specified bucket. The rule specified in the Object Lock configuration will be applied by default to every new object placed in the specified bucket. For more information, see [Locking Objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/object-lock.html).
* + The ``DefaultRetention`` settings require both a mode and a period.
* + The ``DefaultRetention`` period can be either ``Days`` or ``Years`` but you must select one. You cannot specify ``Days`` and ``Years`` at the same time.
* + You can enable Object Lock for new or existing buckets. For more information, see [Configuring Object Lock](https://docs.aws.amazon.com/AmazonS3/latest/userguide/object-lock-configure.html).
*/
public val objectLockConfiguration: Output?
get() = javaResource.objectLockConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketObjectLockConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Indicates whether this bucket has an Object Lock configuration enabled. Enable ``ObjectLockEnabled`` when you apply ``ObjectLockConfiguration`` to a bucket.
*/
public val objectLockEnabled: Output?
get() = javaResource.objectLockEnabled().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Configuration that defines how Amazon S3 handles Object Ownership rules.
*/
public val ownershipControls: Output?
get() = javaResource.ownershipControls().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketOwnershipControlsToKotlin(args0) })
}).orElse(null)
})
/**
* Configuration that defines how Amazon S3 handles public access.
*/
public val publicAccessBlockConfiguration: Output?
get() = javaResource.publicAccessBlockConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketPublicAccessBlockConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Returns the regional domain name of the specified bucket.
* Example: `DOC-EXAMPLE-BUCKET.s3.us-east-2.amazonaws.com`
*/
public val regionalDomainName: Output
get() = javaResource.regionalDomainName().applyValue({ args0 -> args0 })
/**
* Configuration for replicating objects in an S3 bucket. To enable replication, you must also enable versioning by using the ``VersioningConfiguration`` property.
* Amazon S3 can store replicated objects in a single destination bucket or multiple destination buckets. The destination bucket or buckets must already exist.
*/
public val replicationConfiguration: Output?
get() = javaResource.replicationConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketReplicationConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* An arbitrary set of tags (key-value pairs) for this S3 bucket.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* Enables multiple versions of all objects in this bucket. You might enable versioning to prevent objects from being deleted or overwritten by mistake or to archive objects so that you can retrieve previous versions of them.
* When you enable versioning on a bucket for the first time, it might take a short amount of time for the change to be fully propagated. We recommend that you wait for 15 minutes after enabling versioning before issuing write operations (``PUT`` or ``DELETE``) on objects in the bucket.
*/
public val versioningConfiguration: Output?
get() = javaResource.versioningConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketVersioningConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Information used to configure the bucket as a static website. For more information, see [Hosting Websites on Amazon S3](https://docs.aws.amazon.com/AmazonS3/latest/dev/WebsiteHosting.html).
*/
public val websiteConfiguration: Output?
get() = javaResource.websiteConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> bucketWebsiteConfigurationToKotlin(args0) })
}).orElse(null)
})
/**
* Returns the Amazon S3 website endpoint for the specified bucket.
* Example (IPv4): `http://DOC-EXAMPLE-BUCKET.s3-website.us-east-2.amazonaws.com`
* Example (IPv6): `http://DOC-EXAMPLE-BUCKET.s3.dualstack.us-east-2.amazonaws.com`
*/
public val websiteUrl: Output
get() = javaResource.websiteUrl().applyValue({ args0 -> args0 })
}
public object BucketMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.s3.Bucket::class == javaResource::class
override fun map(javaResource: Resource): Bucket = Bucket(
javaResource as
com.pulumi.awsnative.s3.Bucket,
)
}
/**
* @see [Bucket].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Bucket].
*/
public suspend fun bucket(name: String, block: suspend BucketResourceBuilder.() -> Unit): Bucket {
val builder = BucketResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Bucket].
* @param name The _unique_ name of the resulting resource.
*/
public fun bucket(name: String): Bucket {
val builder = BucketResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy