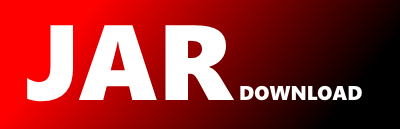
com.pulumi.awsnative.s3.kotlin.BucketPolicy.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [BucketPolicy].
*/
@PulumiTagMarker
public class BucketPolicyResourceBuilder internal constructor() {
public var name: String? = null
public var args: BucketPolicyArgs = BucketPolicyArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BucketPolicyArgsBuilder.() -> Unit) {
val builder = BucketPolicyArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BucketPolicy {
val builtJavaResource = com.pulumi.awsnative.s3.BucketPolicy(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BucketPolicy(builtJavaResource)
}
}
/**
* Applies an Amazon S3 bucket policy to an Amazon S3 bucket. If you are using an identity other than the root user of the AWS-account that owns the bucket, the calling identity must have the ``PutBucketPolicy`` permissions on the specified bucket and belong to the bucket owner's account in order to use this operation.
* If you don't have ``PutBucketPolicy`` permissions, Amazon S3 returns a ``403 Access Denied`` error. If you have the correct permissions, but you're not using an identity that belongs to the bucket owner's account, Amazon S3 returns a ``405 Method Not Allowed`` error.
* As a security precaution, the root user of the AWS-account that owns a bucket can always use this operation, even if the policy explicitly denies the root user the ability to perform this action.
* For more information, see [Bucket policy examples](https://docs.aws.amazon.com/AmazonS3/latest/userguide/example-bucket-policies.html).
* The following operations are related to ``PutBucketPolicy``:
* + [CreateBucket](https://docs.aws.amazon.com/AmazonS3/latest/API/API_CreateBucket.html)
* + [DeleteBucket](https://docs.aws.amazon.com/AmazonS3/latest/API/API_DeleteBucket.html)
*/
public class BucketPolicy internal constructor(
override val javaResource: com.pulumi.awsnative.s3.BucketPolicy,
) : KotlinCustomResource(javaResource, BucketPolicyMapper) {
/**
* The name of the Amazon S3 bucket to which the policy applies.
*/
public val bucket: Output
get() = javaResource.bucket().applyValue({ args0 -> args0 })
/**
* A policy document containing permissions to add to the specified bucket. In IAM, you must provide policy documents in JSON format. However, in CloudFormation you can provide the policy in JSON or YAML format because CloudFormation converts YAML to JSON before submitting it to IAM. For more information, see the AWS::IAM::Policy [PolicyDocument](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/aws-resource-iam-policy.html#cfn-iam-policy-policydocument) resource description in this guide and [Access Policy Language Overview](https://docs.aws.amazon.com/AmazonS3/latest/dev/access-policy-language-overview.html) in the *Amazon S3 User Guide*.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::S3::BucketPolicy` for more information about the expected schema for this property.
*/
public val policyDocument: Output
get() = javaResource.policyDocument().applyValue({ args0 -> args0 })
}
public object BucketPolicyMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.s3.BucketPolicy::class == javaResource::class
override fun map(javaResource: Resource): BucketPolicy = BucketPolicy(
javaResource as
com.pulumi.awsnative.s3.BucketPolicy,
)
}
/**
* @see [BucketPolicy].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [BucketPolicy].
*/
public suspend fun bucketPolicy(
name: String,
block: suspend BucketPolicyResourceBuilder.() -> Unit,
): BucketPolicy {
val builder = BucketPolicyResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [BucketPolicy].
* @param name The _unique_ name of the resulting resource.
*/
public fun bucketPolicy(name: String): BucketPolicy {
val builder = BucketPolicyResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy