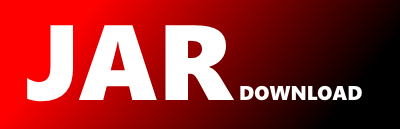
com.pulumi.awsnative.s3.kotlin.inputs.BucketIntelligentTieringConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin.inputs
import com.pulumi.awsnative.s3.inputs.BucketIntelligentTieringConfigurationArgs.builder
import com.pulumi.awsnative.s3.kotlin.enums.BucketIntelligentTieringConfigurationStatus
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Specifies the S3 Intelligent-Tiering configuration for an Amazon S3 bucket.
* For information about the S3 Intelligent-Tiering storage class, see [Storage class for automatically optimizing frequently and infrequently accessed objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/storage-class-intro.html#sc-dynamic-data-access).
* @property id The ID used to identify the S3 Intelligent-Tiering configuration.
* @property prefix An object key name prefix that identifies the subset of objects to which the rule applies.
* @property status Specifies the status of the configuration.
* @property tagFilters A container for a key-value pair.
* @property tierings Specifies a list of S3 Intelligent-Tiering storage class tiers in the configuration. At least one tier must be defined in the list. At most, you can specify two tiers in the list, one for each available AccessTier: ``ARCHIVE_ACCESS`` and ``DEEP_ARCHIVE_ACCESS``.
* You only need Intelligent Tiering Configuration enabled on a bucket if you want to automatically move objects stored in the Intelligent-Tiering storage class to Archive Access or Deep Archive Access tiers.
*/
public data class BucketIntelligentTieringConfigurationArgs(
public val id: Output,
public val prefix: Output? = null,
public val status: Output,
public val tagFilters: Output>? = null,
public val tierings: Output>,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.s3.inputs.BucketIntelligentTieringConfigurationArgs =
com.pulumi.awsnative.s3.inputs.BucketIntelligentTieringConfigurationArgs.builder()
.id(id.applyValue({ args0 -> args0 }))
.prefix(prefix?.applyValue({ args0 -> args0 }))
.status(status.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.tagFilters(
tagFilters?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.tierings(
tierings.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [BucketIntelligentTieringConfigurationArgs].
*/
@PulumiTagMarker
public class BucketIntelligentTieringConfigurationArgsBuilder internal constructor() {
private var id: Output? = null
private var prefix: Output? = null
private var status: Output? = null
private var tagFilters: Output>? = null
private var tierings: Output>? = null
/**
* @param value The ID used to identify the S3 Intelligent-Tiering configuration.
*/
@JvmName("chtqpvofdjpploew")
public suspend fun id(`value`: Output) {
this.id = value
}
/**
* @param value An object key name prefix that identifies the subset of objects to which the rule applies.
*/
@JvmName("dwjogsjiepbmibli")
public suspend fun prefix(`value`: Output) {
this.prefix = value
}
/**
* @param value Specifies the status of the configuration.
*/
@JvmName("tqswuuucewewfhah")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value A container for a key-value pair.
*/
@JvmName("avcekmuxbwodwjpk")
public suspend fun tagFilters(`value`: Output>) {
this.tagFilters = value
}
@JvmName("waeyyioltwnoiidx")
public suspend fun tagFilters(vararg values: Output) {
this.tagFilters = Output.all(values.asList())
}
/**
* @param values A container for a key-value pair.
*/
@JvmName("hpmadvnermbetyht")
public suspend fun tagFilters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy