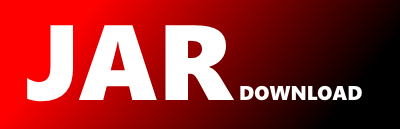
com.pulumi.awsnative.s3.kotlin.inputs.BucketReplicationDestinationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin.inputs
import com.pulumi.awsnative.s3.inputs.BucketReplicationDestinationArgs.builder
import com.pulumi.awsnative.s3.kotlin.enums.BucketReplicationDestinationStorageClass
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* A container for information about the replication destination and its configurations including enabling the S3 Replication Time Control (S3 RTC).
* @property accessControlTranslation Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS-account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS-account that owns the source object.
* @property account Destination bucket owner account ID. In a cross-account scenario, if you direct Amazon S3 to change replica ownership to the AWS-account that owns the destination bucket by specifying the ``AccessControlTranslation`` property, this is the account ID of the destination bucket owner. For more information, see [Cross-Region Replication Additional Configuration: Change Replica Owner](https://docs.aws.amazon.com/AmazonS3/latest/dev/crr-change-owner.html) in the *Amazon S3 User Guide*.
* If you specify the ``AccessControlTranslation`` property, the ``Account`` property is required.
* @property bucket The Amazon Resource Name (ARN) of the bucket where you want Amazon S3 to store the results.
* @property encryptionConfiguration Specifies encryption-related information.
* @property metrics A container specifying replication metrics-related settings enabling replication metrics and events.
* @property replicationTime A container specifying S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. Must be specified together with a ``Metrics`` block.
* @property storageClass The storage class to use when replicating objects, such as S3 Standard or reduced redundancy. By default, Amazon S3 uses the storage class of the source object to create the object replica.
* For valid values, see the ``StorageClass`` element of the [PUT Bucket replication](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketPUTreplication.html) action in the *Amazon S3 API Reference*.
*/
public data class BucketReplicationDestinationArgs(
public val accessControlTranslation: Output? = null,
public val account: Output? = null,
public val bucket: Output,
public val encryptionConfiguration: Output? = null,
public val metrics: Output? = null,
public val replicationTime: Output? = null,
public val storageClass: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.s3.inputs.BucketReplicationDestinationArgs =
com.pulumi.awsnative.s3.inputs.BucketReplicationDestinationArgs.builder()
.accessControlTranslation(
accessControlTranslation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.account(account?.applyValue({ args0 -> args0 }))
.bucket(bucket.applyValue({ args0 -> args0 }))
.encryptionConfiguration(
encryptionConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.metrics(metrics?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.replicationTime(replicationTime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.storageClass(storageClass?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [BucketReplicationDestinationArgs].
*/
@PulumiTagMarker
public class BucketReplicationDestinationArgsBuilder internal constructor() {
private var accessControlTranslation: Output? = null
private var account: Output? = null
private var bucket: Output? = null
private var encryptionConfiguration: Output? = null
private var metrics: Output? = null
private var replicationTime: Output? = null
private var storageClass: Output? = null
/**
* @param value Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS-account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS-account that owns the source object.
*/
@JvmName("hkffsmiicnrkclkd")
public suspend fun accessControlTranslation(`value`: Output) {
this.accessControlTranslation = value
}
/**
* @param value Destination bucket owner account ID. In a cross-account scenario, if you direct Amazon S3 to change replica ownership to the AWS-account that owns the destination bucket by specifying the ``AccessControlTranslation`` property, this is the account ID of the destination bucket owner. For more information, see [Cross-Region Replication Additional Configuration: Change Replica Owner](https://docs.aws.amazon.com/AmazonS3/latest/dev/crr-change-owner.html) in the *Amazon S3 User Guide*.
* If you specify the ``AccessControlTranslation`` property, the ``Account`` property is required.
*/
@JvmName("nesxmbinfytfdrfl")
public suspend fun account(`value`: Output) {
this.account = value
}
/**
* @param value The Amazon Resource Name (ARN) of the bucket where you want Amazon S3 to store the results.
*/
@JvmName("cadupgpegyxexhws")
public suspend fun bucket(`value`: Output) {
this.bucket = value
}
/**
* @param value Specifies encryption-related information.
*/
@JvmName("rfsusqvbugllbscj")
public suspend fun encryptionConfiguration(`value`: Output) {
this.encryptionConfiguration = value
}
/**
* @param value A container specifying replication metrics-related settings enabling replication metrics and events.
*/
@JvmName("jweselddevtxpaii")
public suspend fun metrics(`value`: Output) {
this.metrics = value
}
/**
* @param value A container specifying S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. Must be specified together with a ``Metrics`` block.
*/
@JvmName("tdiespkchyjiyvfi")
public suspend fun replicationTime(`value`: Output) {
this.replicationTime = value
}
/**
* @param value The storage class to use when replicating objects, such as S3 Standard or reduced redundancy. By default, Amazon S3 uses the storage class of the source object to create the object replica.
* For valid values, see the ``StorageClass`` element of the [PUT Bucket replication](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketPUTreplication.html) action in the *Amazon S3 API Reference*.
*/
@JvmName("lcihroxsrongotsv")
public suspend fun storageClass(`value`: Output) {
this.storageClass = value
}
/**
* @param value Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS-account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS-account that owns the source object.
*/
@JvmName("jpfwwsdojtpsvvmh")
public suspend fun accessControlTranslation(`value`: BucketAccessControlTranslationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessControlTranslation = mapped
}
/**
* @param argument Specify this only in a cross-account scenario (where source and destination bucket owners are not the same), and you want to change replica ownership to the AWS-account that owns the destination bucket. If this is not specified in the replication configuration, the replicas are owned by same AWS-account that owns the source object.
*/
@JvmName("kpdxxdotcotvxedo")
public suspend fun accessControlTranslation(argument: suspend BucketAccessControlTranslationArgsBuilder.() -> Unit) {
val toBeMapped = BucketAccessControlTranslationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.accessControlTranslation = mapped
}
/**
* @param value Destination bucket owner account ID. In a cross-account scenario, if you direct Amazon S3 to change replica ownership to the AWS-account that owns the destination bucket by specifying the ``AccessControlTranslation`` property, this is the account ID of the destination bucket owner. For more information, see [Cross-Region Replication Additional Configuration: Change Replica Owner](https://docs.aws.amazon.com/AmazonS3/latest/dev/crr-change-owner.html) in the *Amazon S3 User Guide*.
* If you specify the ``AccessControlTranslation`` property, the ``Account`` property is required.
*/
@JvmName("belxmdadstpwaphl")
public suspend fun account(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.account = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the bucket where you want Amazon S3 to store the results.
*/
@JvmName("ffxtktwyapfavhaq")
public suspend fun bucket(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucket = mapped
}
/**
* @param value Specifies encryption-related information.
*/
@JvmName("iwibjrtlbyinrsfk")
public suspend fun encryptionConfiguration(`value`: BucketEncryptionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfiguration = mapped
}
/**
* @param argument Specifies encryption-related information.
*/
@JvmName("ypvnkvpxgwcokpas")
public suspend fun encryptionConfiguration(argument: suspend BucketEncryptionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = BucketEncryptionConfigurationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.encryptionConfiguration = mapped
}
/**
* @param value A container specifying replication metrics-related settings enabling replication metrics and events.
*/
@JvmName("gpbdccaoxnijyiup")
public suspend fun metrics(`value`: BucketMetricsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metrics = mapped
}
/**
* @param argument A container specifying replication metrics-related settings enabling replication metrics and events.
*/
@JvmName("bsmotudddqxperda")
public suspend fun metrics(argument: suspend BucketMetricsArgsBuilder.() -> Unit) {
val toBeMapped = BucketMetricsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metrics = mapped
}
/**
* @param value A container specifying S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. Must be specified together with a ``Metrics`` block.
*/
@JvmName("sxmlckosxqxjlgas")
public suspend fun replicationTime(`value`: BucketReplicationTimeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replicationTime = mapped
}
/**
* @param argument A container specifying S3 Replication Time Control (S3 RTC), including whether S3 RTC is enabled and the time when all objects and operations on objects must be replicated. Must be specified together with a ``Metrics`` block.
*/
@JvmName("rxgbasxgblkphvga")
public suspend fun replicationTime(argument: suspend BucketReplicationTimeArgsBuilder.() -> Unit) {
val toBeMapped = BucketReplicationTimeArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.replicationTime = mapped
}
/**
* @param value The storage class to use when replicating objects, such as S3 Standard or reduced redundancy. By default, Amazon S3 uses the storage class of the source object to create the object replica.
* For valid values, see the ``StorageClass`` element of the [PUT Bucket replication](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketPUTreplication.html) action in the *Amazon S3 API Reference*.
*/
@JvmName("ojsdxbaoojtxnpvf")
public suspend fun storageClass(`value`: BucketReplicationDestinationStorageClass?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.storageClass = mapped
}
internal fun build(): BucketReplicationDestinationArgs = BucketReplicationDestinationArgs(
accessControlTranslation = accessControlTranslation,
account = account,
bucket = bucket ?: throw PulumiNullFieldException("bucket"),
encryptionConfiguration = encryptionConfiguration,
metrics = metrics,
replicationTime = replicationTime,
storageClass = storageClass,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy