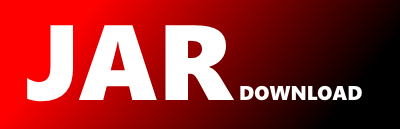
com.pulumi.awsnative.s3.kotlin.inputs.BucketServerSideEncryptionByDefaultArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.s3.kotlin.inputs
import com.pulumi.awsnative.s3.inputs.BucketServerSideEncryptionByDefaultArgs.builder
import com.pulumi.awsnative.s3.kotlin.enums.BucketServerSideEncryptionByDefaultSseAlgorithm
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Describes the default server-side encryption to apply to new objects in the bucket. If a PUT Object request doesn't specify any server-side encryption, this default encryption will be applied. If you don't specify a customer managed key at configuration, Amazon S3 automatically creates an AWS KMS key in your AWS account the first time that you add an object encrypted with SSE-KMS to a bucket. By default, Amazon S3 uses this KMS key for SSE-KMS. For more information, see [PUT Bucket encryption](https://docs.aws.amazon.com/AmazonS3/latest/API/RESTBucketPUTencryption.html) in the *Amazon S3 API Reference*.
* If you're specifying a customer managed KMS key, we recommend using a fully qualified KMS key ARN. If you use a KMS key alias instead, then KMS resolves the key within the requester’s account. This behavior can result in data that's encrypted with a KMS key that belongs to the requester, and not the bucket owner.
* @property kmsMasterKeyId AWS Key Management Service (KMS) customer AWS KMS key ID to use for the default encryption. This parameter is allowed if and only if ``SSEAlgorithm`` is set to ``aws:kms`` or ``aws:kms:dsse``.
* You can specify the key ID, key alias, or the Amazon Resource Name (ARN) of the KMS key.
* + Key ID: ``1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key ARN: ``arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key Alias: ``alias/alias-name``
* If you use a key ID, you can run into a LogDestination undeliverable error when creating a VPC flow log.
* If you are using encryption with cross-account or AWS service operations you must use a fully qualified KMS key ARN. For more information, see [Using encryption for cross-account operations](https://docs.aws.amazon.com/AmazonS3/latest/dev/bucket-encryption.html#bucket-encryption-update-bucket-policy).
* Amazon S3 only supports symmetric encryption KMS keys. For more information, see [Asymmetric keys in KMS](https://docs.aws.amazon.com//kms/latest/developerguide/symmetric-asymmetric.html) in the *Key Management Service Developer Guide*.
* @property sseAlgorithm Server-side encryption algorithm to use for the default encryption.
*/
public data class BucketServerSideEncryptionByDefaultArgs(
public val kmsMasterKeyId: Output? = null,
public val sseAlgorithm: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.s3.inputs.BucketServerSideEncryptionByDefaultArgs =
com.pulumi.awsnative.s3.inputs.BucketServerSideEncryptionByDefaultArgs.builder()
.kmsMasterKeyId(kmsMasterKeyId?.applyValue({ args0 -> args0 }))
.sseAlgorithm(sseAlgorithm.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [BucketServerSideEncryptionByDefaultArgs].
*/
@PulumiTagMarker
public class BucketServerSideEncryptionByDefaultArgsBuilder internal constructor() {
private var kmsMasterKeyId: Output? = null
private var sseAlgorithm: Output? = null
/**
* @param value AWS Key Management Service (KMS) customer AWS KMS key ID to use for the default encryption. This parameter is allowed if and only if ``SSEAlgorithm`` is set to ``aws:kms`` or ``aws:kms:dsse``.
* You can specify the key ID, key alias, or the Amazon Resource Name (ARN) of the KMS key.
* + Key ID: ``1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key ARN: ``arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key Alias: ``alias/alias-name``
* If you use a key ID, you can run into a LogDestination undeliverable error when creating a VPC flow log.
* If you are using encryption with cross-account or AWS service operations you must use a fully qualified KMS key ARN. For more information, see [Using encryption for cross-account operations](https://docs.aws.amazon.com/AmazonS3/latest/dev/bucket-encryption.html#bucket-encryption-update-bucket-policy).
* Amazon S3 only supports symmetric encryption KMS keys. For more information, see [Asymmetric keys in KMS](https://docs.aws.amazon.com//kms/latest/developerguide/symmetric-asymmetric.html) in the *Key Management Service Developer Guide*.
*/
@JvmName("mdqlwendulmqqrxw")
public suspend fun kmsMasterKeyId(`value`: Output) {
this.kmsMasterKeyId = value
}
/**
* @param value Server-side encryption algorithm to use for the default encryption.
*/
@JvmName("xdwqylhnpbdwcnbo")
public suspend fun sseAlgorithm(`value`: Output) {
this.sseAlgorithm = value
}
/**
* @param value AWS Key Management Service (KMS) customer AWS KMS key ID to use for the default encryption. This parameter is allowed if and only if ``SSEAlgorithm`` is set to ``aws:kms`` or ``aws:kms:dsse``.
* You can specify the key ID, key alias, or the Amazon Resource Name (ARN) of the KMS key.
* + Key ID: ``1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key ARN: ``arn:aws:kms:us-east-2:111122223333:key/1234abcd-12ab-34cd-56ef-1234567890ab``
* + Key Alias: ``alias/alias-name``
* If you use a key ID, you can run into a LogDestination undeliverable error when creating a VPC flow log.
* If you are using encryption with cross-account or AWS service operations you must use a fully qualified KMS key ARN. For more information, see [Using encryption for cross-account operations](https://docs.aws.amazon.com/AmazonS3/latest/dev/bucket-encryption.html#bucket-encryption-update-bucket-policy).
* Amazon S3 only supports symmetric encryption KMS keys. For more information, see [Asymmetric keys in KMS](https://docs.aws.amazon.com//kms/latest/developerguide/symmetric-asymmetric.html) in the *Key Management Service Developer Guide*.
*/
@JvmName("sgfjfvruxrevmrjh")
public suspend fun kmsMasterKeyId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kmsMasterKeyId = mapped
}
/**
* @param value Server-side encryption algorithm to use for the default encryption.
*/
@JvmName("xptluprtxupmmwqp")
public suspend fun sseAlgorithm(`value`: BucketServerSideEncryptionByDefaultSseAlgorithm) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.sseAlgorithm = mapped
}
internal fun build(): BucketServerSideEncryptionByDefaultArgs =
BucketServerSideEncryptionByDefaultArgs(
kmsMasterKeyId = kmsMasterKeyId,
sseAlgorithm = sseAlgorithm ?: throw PulumiNullFieldException("sseAlgorithm"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy