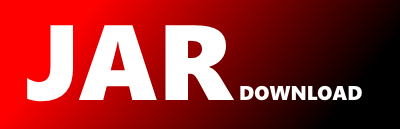
com.pulumi.awsnative.sagemaker.kotlin.FeatureGroup.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag
import com.pulumi.awsnative.sagemaker.kotlin.outputs.FeatureGroupFeatureDefinition
import com.pulumi.awsnative.sagemaker.kotlin.outputs.FeatureGroupThroughputConfig
import com.pulumi.awsnative.sagemaker.kotlin.outputs.OfflineStoreConfigProperties
import com.pulumi.awsnative.sagemaker.kotlin.outputs.OnlineStoreConfigProperties
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag.Companion.toKotlin as createOnlyTagToKotlin
import com.pulumi.awsnative.sagemaker.kotlin.outputs.FeatureGroupFeatureDefinition.Companion.toKotlin as featureGroupFeatureDefinitionToKotlin
import com.pulumi.awsnative.sagemaker.kotlin.outputs.FeatureGroupThroughputConfig.Companion.toKotlin as featureGroupThroughputConfigToKotlin
import com.pulumi.awsnative.sagemaker.kotlin.outputs.OfflineStoreConfigProperties.Companion.toKotlin as offlineStoreConfigPropertiesToKotlin
import com.pulumi.awsnative.sagemaker.kotlin.outputs.OnlineStoreConfigProperties.Companion.toKotlin as onlineStoreConfigPropertiesToKotlin
/**
* Builder for [FeatureGroup].
*/
@PulumiTagMarker
public class FeatureGroupResourceBuilder internal constructor() {
public var name: String? = null
public var args: FeatureGroupArgs = FeatureGroupArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend FeatureGroupArgsBuilder.() -> Unit) {
val builder = FeatureGroupArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): FeatureGroup {
val builtJavaResource = com.pulumi.awsnative.sagemaker.FeatureGroup(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return FeatureGroup(builtJavaResource)
}
}
/**
* Resource Type definition for AWS::SageMaker::FeatureGroup
*/
public class FeatureGroup internal constructor(
override val javaResource: com.pulumi.awsnative.sagemaker.FeatureGroup,
) : KotlinCustomResource(javaResource, FeatureGroupMapper) {
/**
* A timestamp of FeatureGroup creation time.
*/
public val creationTime: Output
get() = javaResource.creationTime().applyValue({ args0 -> args0 })
/**
* Description about the FeatureGroup.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Event Time Feature Name.
*/
public val eventTimeFeatureName: Output
get() = javaResource.eventTimeFeatureName().applyValue({ args0 -> args0 })
/**
* An Array of Feature Definition
*/
public val featureDefinitions: Output>
get() = javaResource.featureDefinitions().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> featureGroupFeatureDefinitionToKotlin(args0) })
})
})
/**
* The Name of the FeatureGroup.
*/
public val featureGroupName: Output
get() = javaResource.featureGroupName().applyValue({ args0 -> args0 })
/**
* The status of the feature group.
*/
public val featureGroupStatus: Output
get() = javaResource.featureGroupStatus().applyValue({ args0 -> args0 })
/**
* The configuration of an `OfflineStore` .
*/
public val offlineStoreConfig: Output?
get() = javaResource.offlineStoreConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> offlineStoreConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* The configuration of an `OnlineStore` .
*/
public val onlineStoreConfig: Output?
get() = javaResource.onlineStoreConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> onlineStoreConfigPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* The Record Identifier Feature Name.
*/
public val recordIdentifierFeatureName: Output
get() = javaResource.recordIdentifierFeatureName().applyValue({ args0 -> args0 })
/**
* Role Arn
*/
public val roleArn: Output?
get() = javaResource.roleArn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* An array of key-value pair to apply to this resource.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> createOnlyTagToKotlin(args0) })
})
}).orElse(null)
})
/**
* Used to set feature group throughput configuration. There are two modes: `ON_DEMAND` and `PROVISIONED` . With on-demand mode, you are charged for data reads and writes that your application performs on your feature group. You do not need to specify read and write throughput because Feature Store accommodates your workloads as they ramp up and down. You can switch a feature group to on-demand only once in a 24 hour period. With provisioned throughput mode, you specify the read and write capacity per second that you expect your application to require, and you are billed based on those limits. Exceeding provisioned throughput will result in your requests being throttled.
* Note: `PROVISIONED` throughput mode is supported only for feature groups that are offline-only, or use the [`Standard`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_OnlineStoreConfig.html#sagemaker-Type-OnlineStoreConfig-StorageType) tier online store.
*/
public val throughputConfig: Output?
get() = javaResource.throughputConfig().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> featureGroupThroughputConfigToKotlin(args0) })
}).orElse(null)
})
}
public object FeatureGroupMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.sagemaker.FeatureGroup::class == javaResource::class
override fun map(javaResource: Resource): FeatureGroup = FeatureGroup(
javaResource as
com.pulumi.awsnative.sagemaker.FeatureGroup,
)
}
/**
* @see [FeatureGroup].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [FeatureGroup].
*/
public suspend fun featureGroup(
name: String,
block: suspend FeatureGroupResourceBuilder.() -> Unit,
): FeatureGroup {
val builder = FeatureGroupResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [FeatureGroup].
* @param name The _unique_ name of the resulting resource.
*/
public fun featureGroup(name: String): FeatureGroup {
val builder = FeatureGroupResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy