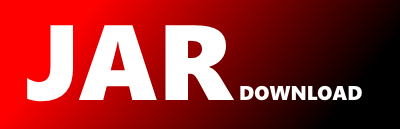
com.pulumi.awsnative.sagemaker.kotlin.UserProfile.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag
import com.pulumi.awsnative.sagemaker.kotlin.outputs.UserProfileUserSettings
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.CreateOnlyTag.Companion.toKotlin as createOnlyTagToKotlin
import com.pulumi.awsnative.sagemaker.kotlin.outputs.UserProfileUserSettings.Companion.toKotlin as userProfileUserSettingsToKotlin
/**
* Builder for [UserProfile].
*/
@PulumiTagMarker
public class UserProfileResourceBuilder internal constructor() {
public var name: String? = null
public var args: UserProfileArgs = UserProfileArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend UserProfileArgsBuilder.() -> Unit) {
val builder = UserProfileArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): UserProfile {
val builtJavaResource = com.pulumi.awsnative.sagemaker.UserProfile(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return UserProfile(builtJavaResource)
}
}
/**
* Resource Type definition for AWS::SageMaker::UserProfile
*/
public class UserProfile internal constructor(
override val javaResource: com.pulumi.awsnative.sagemaker.UserProfile,
) : KotlinCustomResource(javaResource, UserProfileMapper) {
/**
* The ID of the associated Domain.
*/
public val domainId: Output
get() = javaResource.domainId().applyValue({ args0 -> args0 })
/**
* A specifier for the type of value specified in SingleSignOnUserValue. Currently, the only supported value is "UserName". If the Domain's AuthMode is SSO, this field is required. If the Domain's AuthMode is not SSO, this field cannot be specified.
*/
public val singleSignOnUserIdentifier: Output?
get() = javaResource.singleSignOnUserIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The username of the associated AWS Single Sign-On User for this UserProfile. If the Domain's AuthMode is SSO, this field is required, and must match a valid username of a user in your directory. If the Domain's AuthMode is not SSO, this field cannot be specified.
*/
public val singleSignOnUserValue: Output?
get() = javaResource.singleSignOnUserValue().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of tags to apply to the user profile.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> createOnlyTagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The user profile Amazon Resource Name (ARN).
*/
public val userProfileArn: Output
get() = javaResource.userProfileArn().applyValue({ args0 -> args0 })
/**
* A name for the UserProfile.
*/
public val userProfileName: Output
get() = javaResource.userProfileName().applyValue({ args0 -> args0 })
/**
* A collection of settings.
*/
public val userSettings: Output?
get() = javaResource.userSettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
userProfileUserSettingsToKotlin(args0)
})
}).orElse(null)
})
}
public object UserProfileMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.sagemaker.UserProfile::class == javaResource::class
override fun map(javaResource: Resource): UserProfile = UserProfile(
javaResource as
com.pulumi.awsnative.sagemaker.UserProfile,
)
}
/**
* @see [UserProfile].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [UserProfile].
*/
public suspend fun userProfile(name: String, block: suspend UserProfileResourceBuilder.() -> Unit): UserProfile {
val builder = UserProfileResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [UserProfile].
* @param name The _unique_ name of the resulting resource.
*/
public fun userProfile(name: String): UserProfile {
val builder = UserProfileResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy