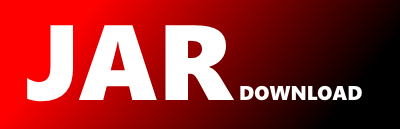
com.pulumi.awsnative.sagemaker.kotlin.inputs.AppResourceSpecArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.AppResourceSpecArgs.builder
import com.pulumi.awsnative.sagemaker.kotlin.enums.AppResourceSpecInstanceType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property instanceType The instance type that the image version runs on.
* @property lifecycleConfigArn The Amazon Resource Name (ARN) of the Lifecycle Configuration to attach to the Resource.
* @property sageMakerImageArn The ARN of the SageMaker image that the image version belongs to.
* @property sageMakerImageVersionArn The ARN of the image version created on the instance.
*/
public data class AppResourceSpecArgs(
public val instanceType: Output? = null,
public val lifecycleConfigArn: Output? = null,
public val sageMakerImageArn: Output? = null,
public val sageMakerImageVersionArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.AppResourceSpecArgs =
com.pulumi.awsnative.sagemaker.inputs.AppResourceSpecArgs.builder()
.instanceType(instanceType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.lifecycleConfigArn(lifecycleConfigArn?.applyValue({ args0 -> args0 }))
.sageMakerImageArn(sageMakerImageArn?.applyValue({ args0 -> args0 }))
.sageMakerImageVersionArn(sageMakerImageVersionArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AppResourceSpecArgs].
*/
@PulumiTagMarker
public class AppResourceSpecArgsBuilder internal constructor() {
private var instanceType: Output? = null
private var lifecycleConfigArn: Output? = null
private var sageMakerImageArn: Output? = null
private var sageMakerImageVersionArn: Output? = null
/**
* @param value The instance type that the image version runs on.
*/
@JvmName("eqdhesusdhpgnvga")
public suspend fun instanceType(`value`: Output) {
this.instanceType = value
}
/**
* @param value The Amazon Resource Name (ARN) of the Lifecycle Configuration to attach to the Resource.
*/
@JvmName("ivbnecwphisyimpk")
public suspend fun lifecycleConfigArn(`value`: Output) {
this.lifecycleConfigArn = value
}
/**
* @param value The ARN of the SageMaker image that the image version belongs to.
*/
@JvmName("ymvslqwxfaehujko")
public suspend fun sageMakerImageArn(`value`: Output) {
this.sageMakerImageArn = value
}
/**
* @param value The ARN of the image version created on the instance.
*/
@JvmName("tsrqtgcncntptfmk")
public suspend fun sageMakerImageVersionArn(`value`: Output) {
this.sageMakerImageVersionArn = value
}
/**
* @param value The instance type that the image version runs on.
*/
@JvmName("buuhxcsmneuinbgh")
public suspend fun instanceType(`value`: AppResourceSpecInstanceType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceType = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the Lifecycle Configuration to attach to the Resource.
*/
@JvmName("dgqdrppktsnradjl")
public suspend fun lifecycleConfigArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lifecycleConfigArn = mapped
}
/**
* @param value The ARN of the SageMaker image that the image version belongs to.
*/
@JvmName("eykfubklggqxxicg")
public suspend fun sageMakerImageArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sageMakerImageArn = mapped
}
/**
* @param value The ARN of the image version created on the instance.
*/
@JvmName("yhvjtgwvttnhvhmx")
public suspend fun sageMakerImageVersionArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sageMakerImageVersionArn = mapped
}
internal fun build(): AppResourceSpecArgs = AppResourceSpecArgs(
instanceType = instanceType,
lifecycleConfigArn = lifecycleConfigArn,
sageMakerImageArn = sageMakerImageArn,
sageMakerImageVersionArn = sageMakerImageVersionArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy