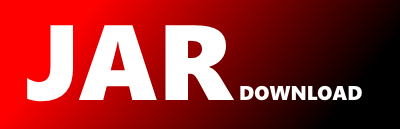
com.pulumi.awsnative.sagemaker.kotlin.inputs.DataQualityJobDefinitionDataQualityAppSpecificationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.DataQualityJobDefinitionDataQualityAppSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Container image configuration object for the monitoring job.
* @property containerArguments An array of arguments for the container used to run the monitoring job.
* @property containerEntrypoint Specifies the entrypoint for a container used to run the monitoring job.
* @property environment Sets the environment variables in the Docker container
* @property imageUri The container image to be run by the monitoring job.
* @property postAnalyticsProcessorSourceUri An Amazon S3 URI to a script that is called after analysis has been performed. Applicable only for the built-in (first party) containers.
* @property recordPreprocessorSourceUri An Amazon S3 URI to a script that is called per row prior to running analysis. It can base64 decode the payload and convert it into a flatted json so that the built-in container can use the converted data. Applicable only for the built-in (first party) containers
*/
public data class DataQualityJobDefinitionDataQualityAppSpecificationArgs(
public val containerArguments: Output>? = null,
public val containerEntrypoint: Output>? = null,
public val environment: Output? = null,
public val imageUri: Output,
public val postAnalyticsProcessorSourceUri: Output? = null,
public val recordPreprocessorSourceUri: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.DataQualityJobDefinitionDataQualityAppSpecificationArgs =
com.pulumi.awsnative.sagemaker.inputs.DataQualityJobDefinitionDataQualityAppSpecificationArgs.builder()
.containerArguments(containerArguments?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.containerEntrypoint(containerEntrypoint?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.environment(environment?.applyValue({ args0 -> args0 }))
.imageUri(imageUri.applyValue({ args0 -> args0 }))
.postAnalyticsProcessorSourceUri(postAnalyticsProcessorSourceUri?.applyValue({ args0 -> args0 }))
.recordPreprocessorSourceUri(recordPreprocessorSourceUri?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DataQualityJobDefinitionDataQualityAppSpecificationArgs].
*/
@PulumiTagMarker
public class DataQualityJobDefinitionDataQualityAppSpecificationArgsBuilder internal constructor() {
private var containerArguments: Output>? = null
private var containerEntrypoint: Output>? = null
private var environment: Output? = null
private var imageUri: Output? = null
private var postAnalyticsProcessorSourceUri: Output? = null
private var recordPreprocessorSourceUri: Output? = null
/**
* @param value An array of arguments for the container used to run the monitoring job.
*/
@JvmName("mxaxfbihssskqybw")
public suspend fun containerArguments(`value`: Output>) {
this.containerArguments = value
}
@JvmName("uxxancxesjgcgwim")
public suspend fun containerArguments(vararg values: Output) {
this.containerArguments = Output.all(values.asList())
}
/**
* @param values An array of arguments for the container used to run the monitoring job.
*/
@JvmName("qvarfxnwksjewlqb")
public suspend fun containerArguments(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy