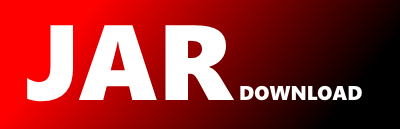
com.pulumi.awsnative.sagemaker.kotlin.inputs.InferenceComponentSpecificationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.inputs
import com.pulumi.awsnative.sagemaker.inputs.InferenceComponentSpecificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The specification for the inference component
* @property computeResourceRequirements The compute resources allocated to run the model assigned to the inference component.
* @property container Defines a container that provides the runtime environment for a model that you deploy with an inference component.
* @property modelName The name of an existing SageMaker model object in your account that you want to deploy with the inference component.
* @property startupParameters Settings that take effect while the model container starts up.
*/
public data class InferenceComponentSpecificationArgs(
public val computeResourceRequirements: Output,
public val container: Output? = null,
public val modelName: Output? = null,
public val startupParameters: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sagemaker.inputs.InferenceComponentSpecificationArgs =
com.pulumi.awsnative.sagemaker.inputs.InferenceComponentSpecificationArgs.builder()
.computeResourceRequirements(
computeResourceRequirements.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.container(container?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.modelName(modelName?.applyValue({ args0 -> args0 }))
.startupParameters(
startupParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [InferenceComponentSpecificationArgs].
*/
@PulumiTagMarker
public class InferenceComponentSpecificationArgsBuilder internal constructor() {
private var computeResourceRequirements:
Output? = null
private var container: Output? = null
private var modelName: Output? = null
private var startupParameters: Output? = null
/**
* @param value The compute resources allocated to run the model assigned to the inference component.
*/
@JvmName("efrguvgcyufnxmgc")
public suspend fun computeResourceRequirements(`value`: Output) {
this.computeResourceRequirements = value
}
/**
* @param value Defines a container that provides the runtime environment for a model that you deploy with an inference component.
*/
@JvmName("ppdwrvwcyhmnsksm")
public suspend fun container(`value`: Output) {
this.container = value
}
/**
* @param value The name of an existing SageMaker model object in your account that you want to deploy with the inference component.
*/
@JvmName("kyukyvlvsjbiggao")
public suspend fun modelName(`value`: Output) {
this.modelName = value
}
/**
* @param value Settings that take effect while the model container starts up.
*/
@JvmName("jgaewstxirkrerea")
public suspend fun startupParameters(`value`: Output) {
this.startupParameters = value
}
/**
* @param value The compute resources allocated to run the model assigned to the inference component.
*/
@JvmName("ekrkkvbfhjtsckrj")
public suspend fun computeResourceRequirements(`value`: InferenceComponentComputeResourceRequirementsArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.computeResourceRequirements = mapped
}
/**
* @param argument The compute resources allocated to run the model assigned to the inference component.
*/
@JvmName("liwdvqdtwuhabrom")
public suspend fun computeResourceRequirements(argument: suspend InferenceComponentComputeResourceRequirementsArgsBuilder.() -> Unit) {
val toBeMapped = InferenceComponentComputeResourceRequirementsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.computeResourceRequirements = mapped
}
/**
* @param value Defines a container that provides the runtime environment for a model that you deploy with an inference component.
*/
@JvmName("tyvhogaaguanlwaa")
public suspend fun container(`value`: InferenceComponentContainerSpecificationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.container = mapped
}
/**
* @param argument Defines a container that provides the runtime environment for a model that you deploy with an inference component.
*/
@JvmName("nwpoprevvxtpeuws")
public suspend fun container(argument: suspend InferenceComponentContainerSpecificationArgsBuilder.() -> Unit) {
val toBeMapped = InferenceComponentContainerSpecificationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.container = mapped
}
/**
* @param value The name of an existing SageMaker model object in your account that you want to deploy with the inference component.
*/
@JvmName("edmjtsnuxmqqwmmp")
public suspend fun modelName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.modelName = mapped
}
/**
* @param value Settings that take effect while the model container starts up.
*/
@JvmName("splcfmqptvejopns")
public suspend fun startupParameters(`value`: InferenceComponentStartupParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startupParameters = mapped
}
/**
* @param argument Settings that take effect while the model container starts up.
*/
@JvmName("cbomepecslxmlcxd")
public suspend fun startupParameters(argument: suspend InferenceComponentStartupParametersArgsBuilder.() -> Unit) {
val toBeMapped = InferenceComponentStartupParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.startupParameters = mapped
}
internal fun build(): InferenceComponentSpecificationArgs = InferenceComponentSpecificationArgs(
computeResourceRequirements = computeResourceRequirements ?: throw
PulumiNullFieldException("computeResourceRequirements"),
container = container,
modelName = modelName,
startupParameters = startupParameters,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy