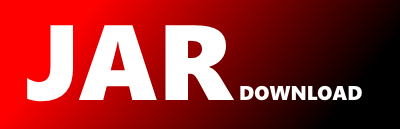
com.pulumi.awsnative.sagemaker.kotlin.outputs.GetInferenceExperimentResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sagemaker.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.sagemaker.kotlin.enums.InferenceExperimentDesiredState
import com.pulumi.awsnative.sagemaker.kotlin.enums.InferenceExperimentStatus
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property arn The Amazon Resource Name (ARN) of the inference experiment.
* @property creationTime The timestamp at which you created the inference experiment.
* @property dataStorageConfig The Amazon S3 location and configuration for storing inference request and response data.
* @property description The description of the inference experiment.
* @property desiredState The desired state of the experiment after starting or stopping operation.
* @property endpointMetadata
* @property lastModifiedTime The timestamp at which you last modified the inference experiment.
* @property modelVariants An array of ModelVariantConfig objects. Each ModelVariantConfig object in the array describes the infrastructure configuration for the corresponding variant.
* @property schedule The duration for which the inference experiment ran or will run.
* The maximum duration that you can set for an inference experiment is 30 days.
* @property shadowModeConfig The configuration of `ShadowMode` inference experiment type, which shows the production variant that takes all the inference requests, and the shadow variant to which Amazon SageMaker replicates a percentage of the inference requests. For the shadow variant it also shows the percentage of requests that Amazon SageMaker replicates.
* @property status The status of the inference experiment.
* @property statusReason The error message or client-specified reason from the StopInferenceExperiment API, that explains the status of the inference experiment.
* @property tags An array of key-value pairs to apply to this resource.
*/
public data class GetInferenceExperimentResult(
public val arn: String? = null,
public val creationTime: String? = null,
public val dataStorageConfig: InferenceExperimentDataStorageConfig? = null,
public val description: String? = null,
public val desiredState: InferenceExperimentDesiredState? = null,
public val endpointMetadata: InferenceExperimentEndpointMetadata? = null,
public val lastModifiedTime: String? = null,
public val modelVariants: List? = null,
public val schedule: InferenceExperimentSchedule? = null,
public val shadowModeConfig: InferenceExperimentShadowModeConfig? = null,
public val status: InferenceExperimentStatus? = null,
public val statusReason: String? = null,
public val tags: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.sagemaker.outputs.GetInferenceExperimentResult): GetInferenceExperimentResult = GetInferenceExperimentResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
creationTime = javaType.creationTime().map({ args0 -> args0 }).orElse(null),
dataStorageConfig = javaType.dataStorageConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.outputs.InferenceExperimentDataStorageConfig.Companion.toKotlin(args0)
})
}).orElse(null),
description = javaType.description().map({ args0 -> args0 }).orElse(null),
desiredState = javaType.desiredState().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.enums.InferenceExperimentDesiredState.Companion.toKotlin(args0)
})
}).orElse(null),
endpointMetadata = javaType.endpointMetadata().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.outputs.InferenceExperimentEndpointMetadata.Companion.toKotlin(args0)
})
}).orElse(null),
lastModifiedTime = javaType.lastModifiedTime().map({ args0 -> args0 }).orElse(null),
modelVariants = javaType.modelVariants().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.outputs.InferenceExperimentModelVariantConfig.Companion.toKotlin(args0)
})
}),
schedule = javaType.schedule().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.outputs.InferenceExperimentSchedule.Companion.toKotlin(args0)
})
}).orElse(null),
shadowModeConfig = javaType.shadowModeConfig().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.outputs.InferenceExperimentShadowModeConfig.Companion.toKotlin(args0)
})
}).orElse(null),
status = javaType.status().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.sagemaker.kotlin.enums.InferenceExperimentStatus.Companion.toKotlin(args0)
})
}).orElse(null),
statusReason = javaType.statusReason().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy